Question
PLEASE HELP, I ONLY NEED TO WRITE Library.java . PLease follow instructions and UML diagram.Don't write your own random program. Make sure it pass the
PLEASE HELP, I ONLY NEED TO WRITE Library.java .
PLease follow instructions and UML diagram.Don't write your own random program. Make sure it pass the Junit test, preferrably using Jgrasp and Dr.java to test it .
I'm providing all the other files necessary. Please help I've been stuck on it for days.
*******************************************************************************************************************************************************************************
Library.java
The Library constructor will simply store the given books and patrons arrays in the object. There is no need to make copies of these arrays.
The checkin method will check in a book upon return. If the returned book belongs in the Library, and it is currently checked out, this method should update the status of the book, reset its patron and due date, determine the fine for this book (if any), and update the patron's balance. The return value indicates success or failure. For example, if the book is not in the library, checkin should return false.
The checkout method will let a patron checkout a book that is available in the Library. If both the patron and the book belong to the library, and the book is available, this method will update the book's status and patron, and set the due date to ten days from today's date. The return value indicates success or failure. For example, if the book is not in the library, checkout should return false.
The determineFine method will compute the fine currently due for a book that is checked out. For this method, the fine rate is $0.50 per day for each day after the due date.
The searchBooks method searches for books, depending on the key and type, and returns an array of the books found. If no books are found, the resulting array will have a length of zero.
When type is TITLE_SEARCH, key will be a string containing the title to search. The method should return all books with that title.
When type is AUTHOR_SEARCH, key will be a String containing the author's name. The method should return all books for that author.
When type is PATRON_SEARCH, key will either be a Patron object or an Integer with the Patron's id. The method should return all books checked out to that patron.
When type is OVERDUE_SEARCH, key will be a Date object. The method should return all books that will be overdue on that date (if not checked in before then).
***************************************************************************************************************************************************************************************
Dr.Java file for Testing code
import junit.framework.TestCase; import java.util.Date;
/** * Tests Library: currently one test method. * * @author * @version * */ public class LibraryTest extends TestCase {
/** A single test for Library. **/ public void testLibrary() {
String title = "Starting Out With Java"; String author = "Tony Gaddis"; String isbn = "978-0-13-395705-1"; int year = 2016; int pages = 1188; String title2 = "Starting Out With Java Edition 4"; String author2 = "T. Gaddis"; String isbn2 = "978-0-13-608020-6"; int year2 = 2008; int pages2 = 977; Book b0 = new Book(title2, author2, isbn2, year2, pages2); Book b1 = new Book(title, author, isbn, year, pages); Book b2 = new Book(title, author, isbn, year, pages); Book[] deesBooks = {b0, b1, b2}; Patron p0 = new Patron("Dee A. B. Weikle", "weikleda@jmu.edu", 2, 1.50); Patron p1 = new Patron("Chris Mayfield", "mayfiecs@jmu.edu", 1, 1.00); Patron p2 = new Patron("Alvin Chao", "chaoaj@jmu.edu", 0, 0); Patron[] deesPatrons = {p0, p1, p2}; Library deesLibrary = new Library(deesBooks, deesPatrons); if (deesLibrary.checkout(deesBooks[1], deesPatrons[2])) { assertEquals("Library checkout1:", Book.UNAVAILABLE, deesBooks[1].getStatus()); assertTrue("Library checkout3:", deesPatrons[2].equals(deesBooks[1].getPatron())); assertEquals("Library checkout 4:", 10, DateUtils.interval(new Date(), deesBooks[1].getDue())); if (deesLibrary.checkin(deesBooks[1])) { assertEquals("Library checkout5", Book.AVAILABLE, deesBooks[1].getStatus()); assertEquals("Library checkout6", null, deesBooks[1].getPatron()); assertEquals("Library checkout7", null, deesBooks[1].getDue()); } } else { assertTrue("Library checkout failed", false); } // Test with book not in the library Book b3 = new Book("Title", "Author", "isbn", 2010, 220); assertFalse("Library no book checkout", deesLibrary.checkout(b3, p0)); // Test determine fine, checkout book with overdue date Date today = new Date(); Date tenDaysAgo = DateUtils.addDays(today, -10); b0.checkout(p0, tenDaysAgo); assertEquals("5.00 fine", 5.00, deesLibrary.determineFine(b0)); // Test search books - something there Book[] actual = deesLibrary.searchBooks("Tony Gaddis", Library.AUTHOR_SEARCH); assertEquals("Library author search 2 books:", 2, actual.length); assertTrue("Library author search 2 books, b1", actual[0] == b1 || actual[1] == b1); assertTrue("Library author search 2 books, b2", actual[0] == b2 || actual[1] == b2); Book[] actual2 = deesLibrary.searchBooks(title, Library.TITLE_SEARCH); assertEquals("Library title search 2 books:", 2, actual2.length); assertTrue("Library title search 2 books, b1", actual2[0] == b1 || actual2[1] == b1); assertTrue("Library title search 2 books, b2", actual2[0] == b2 || actual2[1] == b2); Book[] actual3 = deesLibrary.searchBooks(p0, Library.PATRON_SEARCH); assertEquals("Library patron search 2 books:", 1, actual3.length); assertTrue("Library patron search 2 books, b1", actual3[0] == b0); Book[] actual4 = deesLibrary.searchBooks(new Date(), Library.OVERDUE_SEARCH); assertEquals("Library overdue search 2 books:", 1, actual4.length); assertTrue("Library overdue search 2 books, b1", actual4[0] == b0); // Test search books nothing there Book[] actual5 = deesLibrary.searchBooks("Author", Library.AUTHOR_SEARCH); assertEquals("Library author search not there:", 0, actual5.length); Book[] actual6 = deesLibrary.searchBooks("Title", Library.TITLE_SEARCH); assertEquals("Library title search not there:", 0, actual6.length); Book[] actual7 = deesLibrary.searchBooks(p2, Library.PATRON_SEARCH); assertEquals("Library patron search not there:", 0, actual7.length); Date d = new Date(); Book[] actual8 = deesLibrary.searchBooks(DateUtils.addDays(d, -20), Library.OVERDUE_SEARCH); assertEquals("Library overdue search not there:", 0, actual8.length); // Test patron search with int idnumber instead Book[] actual9 = deesLibrary.searchBooks(0, Library.PATRON_SEARCH); assertEquals("Library patron search idnum not there:", 0, actual9.length); Book[] actual10 = deesLibrary.searchBooks(2, Library.PATRON_SEARCH); assertEquals("Library patron search idnum b0", 1, actual10.length); assertTrue("Library patron search idnum b0", actual10[0] == b0); } }
************************************************************************************************************************************************************************************
Book.java
import java.util.Date;
public class Book {
public static final int AVAILABLE = 1;
public static final int UNAVAILABLE = 2;
private String title;
private String author;
private String isbn;
private int year;
private int pages;
private int status;
private Date due;
private Patron patron;
public Book (String title, String author, String isbn,
int year, int pages) {
this.title = title;
this.author = author;
this.isbn = isbn;
this.year = year;
this.pages = pages;
this.status = AVAILABLE;
Date due = null;
Patron patron = null;
}
public void checkin () {
this.status = AVAILABLE;
this.due = null;
this.patron = null;
}
public void checkout (Patron patron, Date due) {
this.status = UNAVAILABLE;
this.patron = patron;
this.due = due;
}
public boolean equals(Object other) {
boolean result = false;
if (other instanceof Book)
{
Book book = (Book) other;
result = this.isbn.equals(book.isbn);
}
else if (other instanceof String)
{
String str = (String) other;
result = this.isbn.equals(str);
}
return result;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getIsbn() {
return isbn;
}
public int getPages() {
return pages;
}
public int getYear() {
return year;
}
public int getStatus() {
return status;
}
public Date getDue() {
return due;
}
public Patron getPatron () {
return patron;
}
public String toString() {
return String.format("Title: %s, Author: %s, ISBN: %s, Year: %d, Pages: %d.",
title, author, isbn, year, pages);
}
}
******************************************************************************************************************************************************************************************
Patron.java
public class Patron {
private String name;
private String email;
private int idNumber;
private double balance;
public Patron(String name, String email,
int idNumber, double balance) {
this.name = name;
this.email = email;
this.idNumber = idNumber;
this.balance = balance;
}
public double adjustBalance(double amount) {
this.balance = balance;
this.balance = this.balance + amount;
return this.balance;
}
public boolean equals(Object other) {
boolean result = false;
if (other instanceof Patron)
{ Patron patron = (Patron) other;
result = this.idNumber == patron.idNumber;
}
else if (other instanceof Integer)
{ Integer id = (Integer) other;
result = this.idNumber == id;
}
return result;
}
public String toString() {
return String.format("Name: %s, Email: %s, ID: %d, Balance: $%.2f.",
name, email, idNumber, balance);
}
}
**********************************************************************************************************************************************************************************
DateUtils.java
import java.util.Calendar; import java.util.Date;
/** * Utility methods for java.util.Date objects. * * @author * @author * @version */ public class DateUtils { private static final long MILLIS_PER_DAY = 86400000; /** * Adds a number of days to a date. * * @param date the date (unchanged) * @param days number of days to add * @return the new date */ public static Date addDays(Date date, int days) { Calendar c = Calendar.getInstance(); c.setTime(date); c.add(Calendar.DATE, days); return c.getTime(); } /** * Compute the number of days from the "from" date to the "to" date. * The time fields (hour, minute, second, millisecond) are set to zero * before the calculation is made, so that only the year, month, and day * are used to calculate the difference. * * @param from the date starting the interval * @param to the date ending the interval * @return number of whole days in the interval */ public static int interval(Date from, Date to) { Calendar fromCal = Calendar.getInstance(); fromCal.setTime(from); fromCal.set(Calendar.HOUR, 0); fromCal.set(Calendar.MINUTE, 0); fromCal.set(Calendar.SECOND, 0); fromCal.set(Calendar.MILLISECOND, 0); Calendar toCal = Calendar.getInstance(); toCal.setTime(to); toCal.set(Calendar.HOUR, 0); toCal.set(Calendar.MINUTE, 0); toCal.set(Calendar.SECOND, 0); toCal.set(Calendar.MILLISECOND, 0); long diff = toCal.getTimeInMillis() - fromCal.getTimeInMillis(); return (int) (diff / MILLIS_PER_DAY); } }
NEEDED AS SOON AS POSSIBLE, BUT PLEASE MAKE SURE IT PASSES ALL THE DR.JAVA TEST WHEN TESTING IT.
THANKS IN ADVANCE.
sual Paradigm Standardliames Madison Uni versity Driver +main(args: Strin Librar +AUTHOR SEARCH: char'A" +PATRON SEARCH char'P +TITLE SEARCH: char' books : Book[] patrons : Patronl] +Library(books Book[l, patrons: Patron[]) +checkin(book: Book): boolean +checkout(book : Book, patron Patron): boolean DateUtils +interval(from Date, to: date): int +addDays(date Date, days: int): Date in +searchBooks(key: Object, type char) Book[] Book +AVAILABLE : int 1 +UNAVAILABLE int2 title: String author String isbn String pages: int -year: int status : int -due Date patron Patron +Book(title: String, author: String, isbn : String, year: int, pages: int) +checkin() +checkout(patron Patron, due Date) +equals(other: Object): boolean +getx() +toString) String Patron name String email : String idNumber int balance double +Patron(name : String, email String idNumber int, balance: double) +adjustBalance(amount: double) : double +equals(other: Object): boolean +toString) String
Step by Step Solution
There are 3 Steps involved in it
Step: 1
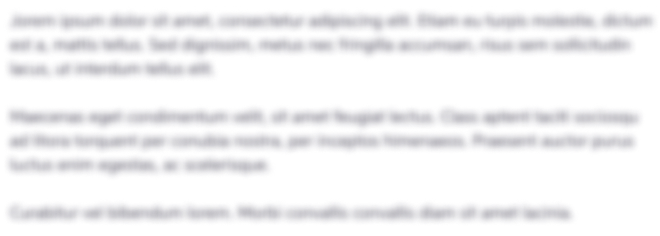
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started