Question
Please help !!! I submitted the code below but I need to use getline instead of cin so the program would read a string with
Please help !!! I submitted the code below but I need to use getline instead of cin so the program would read a string with spaces and I don't know how to do that; also the language is C++
Code I submitted:
// The Header Section
#include
#include
using namespace std;
// The Function Prototypes
char* decrypting(char*);
char* encrypting(char*);
char* input_string();
// The Main function
int main()
{
int opt;
char *str, *opstr;
while(1)
{
// Giving the User Options
cout << " Please Pick a Number From Below: 1 To Encrypt 2 To Decrypt 3 To Exit Your Pick: ";
cin >> opt;
if(opt == 1)
{
// Reading the String
str = input_string();
opstr = encrypting(str);
cout << " Encrypted To: " << opstr;
delete[] str;
}
else if(opt == 2)
{
str = input_string();
opstr = decrypting(str);
cout << " Decrypted To: " << opstr;
delete[] str;
}
else
{
return 0;
}
}
return 0;
}
char* input_string()
{
char *str;
// Allocating The Memory
str = new char[25];
cout << " Input Your String: ";
cin >> str;
return str;
}
// A Function that Decodes the Inputted Message that Uses the Left Rotational Shift Key to a Cypher
char* decrypting(char* cypher)
{
int i,j;
int val, shift=13;
char *decoded_mesg = new char[25];
for(i=0; i { val = (int)(cypher[i]); if(val >= 65 && val <= 90) { if( (val - shift) < 65 ) val = 90 - abs(( (val - shift) - 65 )) + 1; else val = val - shift; decoded_mesg[i] = (char)(val); } else if(val >= 97 && val <= 122) { if( (val - shift) < 97 ) val = 122 - abs(( (val - shift) - 97 )) + 1; else val = val - shift; decoded_mesg[i] = (char)(val); } else decoded_mesg[i] = cypher[i]; } decoded_mesg[i] = '\0'; return decoded_mesg; } // Another Function that Decodes the Inputted Message that Uses the Left Rotational Shift Key to a Plain Text char* encrypting(char* plain_text) { int i,j; int val, shift=13; char *decoded_mesg = new char[25]; for(i=0; i { val = (int)(plain_text[i]); if(val >= 65 && val <= 90) { if( (val + shift) > 90 ) val = 65 + abs(( 90 - (val + shift) )) - 1; else val = val + shift; decoded_mesg[i] = (char)(val); } else if(val >= 97 && val <= 122) { if( (val + shift) > 122 ) val = 97 + abs(( 122 - (val + shift) )) - 1; else val = val + shift; decoded_mesg[i] = (char)(val); } else decoded_mesg[i] = plain_text[i]; } decoded_mesg[i] = '\0'; return decoded_mesg; } The Instructions for writing this code: Cryptography the science of secret writing is an old science; the first recorded use was well before 1900 B.C. An Egyptian writer used previously unknown hieroglyphs in an inscription. We will use a simple substitution cypher called rot13 to encode and decode our secret messages. ROT13 ("rotate by 13 places", sometimes hyphenated ROT-13) is a simple letter substitution cipher that replaces a letter with the 13th letter after it, in the alphabet. ROT13 is a special case of the Caesar cipher which was developed in ancient Rome. Decryption Key A|B|C|D|E|F|G|H|I|J|K|L|M ------------------------- N|O|P|Q|R|S|T|U|V|W|X|Y|Z (letter above equals below, and vice versa) As you can see, A becomes N, B becomes O and so on. Your job is to write a program, with at least four functions, including main, which must do the following: Input will be a string of no more than 25 characters. Blanks get replaced with blanks. Do not worry about punctuation; there will be no punctuation in the string. ALPHABET becomes NYCUNORG Test your program with the following strings: TAF VF paddrpf
Step by Step Solution
There are 3 Steps involved in it
Step: 1
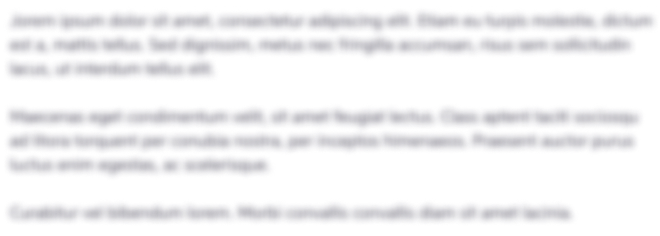
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started