Question
Please help! I'm having a very hard time with java [Stack.java] package ds; public class Stack { public int size; public int top; public int[]
Please help! I'm having a very hard time with java
[Stack.java]
package ds;
public class Stack {
public int size;
public int top;
public int[] array;
public Stack () {
size = 0;
top = -1;
array = null;
}
public Stack (int _size) {
size = _size;
top = -1;
array = new int[size];
}
/*
* Implement the Stack-Empty(S) function
*/
public boolean empty () {
}
/*
* Implement the Push(S, x) function
*/
public void push (int x) {
}
/*
* Implement the Pop(S) function
* Return -1 if the stack is empty
*/
public int pop () {
}
/*
* Convert stack to string in the format of #size, [#elements]
*/
public String toString () {
String str;
str = size + ", [";
for (int i = 0; i
str += array[i] + ", ";
str += "]";
return str;
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Stack s;
s = new Stack(10);
for (int i = 0; i
s.push(i);
System.out.println(s.toString());
for (int i = 0; i
s.pop();
System.out.println(s.toString());
}
}
[Queue.java]
package ds;
public class Queue {
public int size;
public int[] array;
public int head;
public int tail;
public Queue () {
size = 0;
array = null;
head = -1;
tail = 0;
}
public Queue (int _size) {
size = _size;
array = new int[size];
head = -1;
tail = 0;
}
/*
* Implement the ENQUEUE(Q, x) function
*/
public void enqueue (int x) {
}
/*
* Implement the DEQUEUE(Q) function
*/
public int dequeue () {
}
/*
* Convert queue to string in the format of #size, head, tail,
[#elements]
*/
public String toString () {
String str;
str = size + ", " + head + ", " + tail + ", [";
for (int i = head; i%size
str += array[i] + ",";
str += "]";
return str;
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Queue q;
q = new Queue(10);
for (int i = 0; i
q.enqueue(i);
System.out.println(q.toString());
for (int i = 0; i
q.dequeue();
System.out.println(q.toString());
}
}
LinkedList.java
package ds;
public class LinkedList {
public ListNode head;
public LinkedList () {
head = null;
}
/*
* Implement the LIST-SEARCH(L, k) function
*/
public ListNode search (int k) {
}
/*
* Implement the LIST-INSERT(L, x) function
* Note that x is a integer value, not a ListNode
*/
public void insert (int x) {
}
/*
* Implement the LIST-DELETE(L, x) function
*/
public void delete (ListNode x) {
}
/*
* Convert a LinkedList to a string in the format of [#elements]
*/
public String toString () {
String str;
ListNode n;
str = "[";
n = this.head;
while (n != null) {
str += n.key + ",";
n = n.next;
}
str += "]";
return str;
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
LinkedList l;
l = new LinkedList();
for (int i = 0; i
l.insert(i);
System.out.println(l.toString());
for (int i = 0; i
l.delete(l.head.next);
System.out.println(l.toString());
}
}
ListNode.java
package ds;
public class ListNode {
public int key;
public ListNode prev;
public ListNode next;
public ListNode () {
prev = next = null;
}
public ListNode (int _key) {
key = _key;
prev = next = null;
}
}
Instructions. You are provided four skeleton program named Stack.java, Queue.java, ListNode.java and LinkedList.java. The source files are available on Canvas in a folder named HW3. Please modify the skeleton code to solve the following tasks. Task (30 pts). Implement the empty(), push(int z), and pop() function Task 2 (30 pts). Im plem ent the enqueue(int r), dequeue() function for . Task 3 (40 pts). Implement the search(int k), insert(int z), delete) func- for Stack in Stack.java as discussed in Lecture 5. Queue in Queue.java as discussed in Lecture 5. tion in LinkedList.java as discussed in Lecture 6 . Task 4 (10 pts Extra Credit). In the push(int x) function of Stack.java, by default, we never check if the stack is already full. If we insert an element into a full stack, we should get an error. Implement the capacity check feature for push(int x). (Hint: Use System.err.println to print the error message.) . Task 5 (10 pts Extra Credit). In the enqueue(int ) function of Queue.java, we do not check if the queue is already full. Implement the capacity check feature for enqueue(int z). (Hint: Use System.err.println to print the error message.) . Task 6 (10 pts Extra Credit). In the dequeue function of Queue.java, we do not check if the queue is empty. If we dequeue an empty queue, we should also get an error. Implement the empty check feature for en- queue(int z). (Hint: Use System.err.println) to print the error message.)Step by Step Solution
There are 3 Steps involved in it
Step: 1
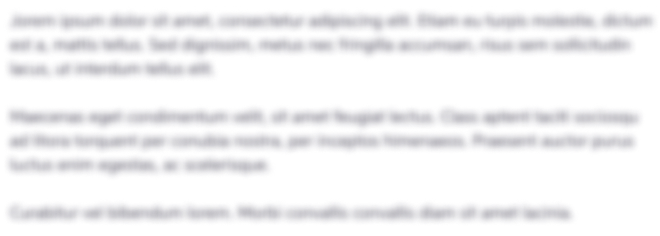
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started