Question
Please help improve and organize the following code using information hiding (code should be separated into modules like: deck, hand, flush, pair, two pair, high
Please help improve and organize the following code using information hiding (code should be separated into modules like: deck, hand, flush, pair, two pair, high card, counts, player_sim)
Any larger functions like pairsCounting and play_rounds should be broken up into smaller functions for better organization.
The play_rounds function should be the main function in the main module player_sim used to run the entire code. (Should have if __name__ == "__play_rounds__":
play_rounds() at the end)
Please also replace single-line comments with one doc string for each function.
Please do NOT use classes or objects.
# This program will help someone figure out how likely certain kinds of hands are # in a simplified version of the game of Poker. import random # Function that calculates the number of pairs in hand: def pairsCounting(cards): # Getting list of ranks from cards in hand: all_ranks = [cards[0][0], cards[1][0], cards[2][0], cards[3][0], cards[4][0]] # Getting unique ranks: unique_ranks = [] for i in all_ranks: if i not in unique_ranks: unique_ranks.append(i) # Calculation of frequencies for ranks in hand: counters = [] for i in unique_ranks: counter = 0 for j in all_ranks: if i == j: counter = counter + 1 counters.append(counter) # Calculation of number of pairs: pairs_n = 0 for i in counters: if i == 4: pairs_n = 2 break elif i == 2 or i == 3: pairs_n = pairs_n + 1 return pairs_n # Function that checks if hand is flush: def isFlush(cards): hasHand = False # If suit is the same for all cards in hand, the value True is assigned to variable hasHand: if cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == \ cards[4][1]: hasHand = True return hasHand # Function that creates deck of cards: def createDeck(): # Creation of deck as list of tuples: initial_deck = [('A', 'Clubs'), ('2', 'Clubs'), ('3', 'Clubs'), ('4', 'Clubs'), ('5', 'Clubs'), ('6', 'Clubs'), ('7', 'Clubs'), ('8', 'Clubs'), ('9', 'Clubs'), ('10', 'Clubs'), ('J', 'Clubs'), ('Q', 'Clubs'), ('K', 'Clubs'), ('A', 'Diamonds'), ('2', 'Diamonds'), ('3', 'Diamonds'), ('4', 'Diamonds'), ('5', 'Diamonds'), ('6', 'Diamonds'), ('7', 'Diamonds'), ('8', 'Diamonds'), ('9', 'Diamonds'), ('10', 'Diamonds'), ('J', 'Diamonds'), ('Q', 'Diamonds'), ('K', 'Diamonds'), ('A', 'Hearts'), ('2', 'Hearts'), ('3', 'Hearts'), ('4', 'Hearts'), ('5', 'Hearts'), ('6', 'Hearts'), ('7', 'Hearts'), ('8', 'Hearts'), ('9', 'Hearts'), ('10', 'Hearts'), ('J', 'Hearts'), ('Q', 'Hearts'), ('K', 'Hearts'), ('A', 'Spades'), ('2', 'Spades'), ('3', 'Spades'), ('4', 'Spades'), ('5', 'Spades'), ('6', 'Spades'), ('7', 'Spades'), ('8', 'Spades'), ('9', 'Spades'), ('10', 'Spades'), ('J', 'Spades'), ('Q', 'Spades'), ('K', 'Spades')] return initial_deck # Function that shuffles deck: def shuffleDeck(input_deck): random.shuffle(input_deck) # Function that takes hand from deck: def dealCards(input_deck): # First five cards are taken to hand from deck (it is supposed that deck is shuffled): new_hand = [input_deck[0], input_deck[1], input_deck[2], input_deck[3], input_deck[4]] return new_hand # Analogue of main function: def play_rounds(): # Creation of deck: deck = createDeck() # Initialization of counters and containers: iterations_n = 0 pairs_counters = [] two_pairs_counters = [] flushes_counters = [] high_cards_counters = [] pairs_counter = 0 two_pairs_counter = 0 flushes_counter = 0 high_cards_counter = 0 # Making 100,000 iterations: for i in range(1, 100000 + 1): # Deck is shuffled: shuffleDeck(deck) # Hand is taken from deck: hand = dealCards(deck) # Checking rank of hand: if isFlush(hand) == True: flushes_counter = flushes_counter + 1 # If hand is flush, respective counter is increased by 1 elif pairsCounting(hand) == 2: two_pairs_counter = two_pairs_counter + 1 # Else if hand is 2 pairs, respective counter is increased by 1 elif pairsCounting(hand) == 1: pairs_counter = pairs_counter + 1 # Else if hand is pair, respective counter is increased by 1 else: high_cards_counter = high_cards_counter + 1 # Else, counter of high card hands is increased by 1 # Number of iterations is increased by 1: iterations_n = iterations_n + 1 # If number of iterations reaches 10000, append results to respective containers and set 0 to number of iterations: if iterations_n == 10000: pairs_counters.append(pairs_counter) two_pairs_counters.append(two_pairs_counter) flushes_counters.append(flushes_counter) high_cards_counters.append(high_cards_counter) iterations_n = 0 # Create list with bins for number of hands: bins = list(range(10000, 100000 + 1, 10000)) # Create and fill lists for shares of different hands: pairs_shares = [] two_pairs_shares = [] flushes_shares = [] high_cards_shares = [] for i in range(0, len(bins)): pairs_shares.append(100 * pairs_counters[i] / bins[i]) two_pairs_shares.append(100 * two_pairs_counters[i] / bins[i]) flushes_shares.append(100 * flushes_counters[i] / bins[i]) high_cards_shares.append(100 * high_cards_counters[i] / bins[i]) # Print the results table: print("# of hands".rjust(12), end="") print("pairs".rjust(10), end="") print("%".center(10), end="") print("2 pairs".rjust(10), end="") print("%".center(10), end="") print("flushes".rjust(10), end="") print("%".center(10), end="") print("high card".rjust(10), end="") print("%".center(10)) for i in range(0, len(bins)): print(format(bins[i], ',d').rjust(12), end="") print(str(pairs_counters[i]).rjust(10), end="") print("{0:05.2f}".format(pairs_shares[i], '02d').center(10), end="") print(str(two_pairs_counters[i]).rjust(10), end="") print("{0:05.2f}".format(two_pairs_shares[i], '02d').center(10), end="") print(str(flushes_counters[i]).rjust(10), end="") print("{0:05.2f}".format(flushes_shares[i], '02d').center(10), end="") print(str(high_cards_counters[i]).rjust(10), end="") print("{0:05.2f}".format(high_cards_shares[i]).center(10)) # Calling the function play_rounds: play_rounds()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
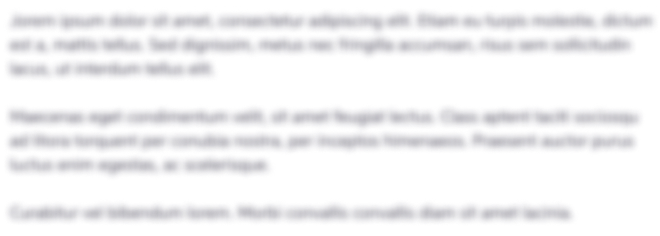
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started