Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please help me answer the following questions related to the C programs given below. Please put where you changed the file and added the print
Please help me answer the following questions related to the C programs given below. Please put where you changed the file and added the print statements along with the answer. Thank You!
cache_sim.c:
#include#include #include "cache.h" // // A simple data store with cache // #define DATA_SIZE 1000 #define CACHE_SIZE 10 // // Data is stored in array data[] // float data[DATA_SIZE]; int nhits = 0; int nmisses = 0; CACHE *cache; // initialize the data array void init() { int i; // initialize data for (i = 0; i = DATA_SIZE) { fprintf(stderr, "get: no data element %d ", i); exit(EXIT_FAILURE); } float x = cache_lookup(cache, i); if (x >= 0) { // cache hit nhits++; return x; } else { // cache miss nmisses++; x = data[i]; cache_insert(cache, i, x); return data[i]; } } // set the ith data value to x void set(int i, float x) { if (i = DATA_SIZE) { fprintf(stderr, "set: no data element %d ", i); exit(EXIT_FAILURE); } data[i] = x; // clear cache entry cache_clear(cache, i); } // simulate a lot of data accesses int main(int argc, char *argv[]) { // simulation parameters int num_accesses = 100000; int dist_size = 10; int move_size1[] = {-3,-2,-1,0,0,0,0,1,2,3}; int move_size2[] = {-5,-4,-3,-2,-1,1,2,3,4,5}; init(); int i,j,delta; j = 0; for (i = 0; i = DATA_SIZE) { j = DATA_SIZE - 1; } get(j); } exit(0); }
random_cache.c
#include#include "random_cache.h" // // A simple cache for positive float values, with a random replacement policy // CACHE *cache_new(int size) { CACHE *cache = malloc(sizeof(CACHE)); cache->size = size; cache->addr = malloc(size * sizeof(int)); cache->data = malloc(size * sizeof(float)); // initialize -- an addr value of -1 means the cache element is unused int i; for (i = 0; i size; i++) { cache->addr[i] = -1; } return cache; } // return value of data element i if it is cached; else return -1 float cache_lookup(CACHE *cache, int i) { int j; for (j = 0; j size; j++) { if (cache->addr[j] == i) { // cache hit return cache->data[j]; } } // cache miss return -1.0; } // record in the cache that the ith data element has value x // a random replacement policy is used void cache_insert(CACHE *cache, int i, float x) { int j = rand() % cache->size; cache->addr[j] = i; cache->data[j] = x; } // clear the ith element of the cache void cache_clear(CACHE *cache, int i) { int j; for (j = 0; j size; j++) { if (cache->addr[j] == i) { cache->addr[j] = -1; break; } } }
random_cache.h
typedef struct cache { int size; // number of cache elements int *addr; // index of element float *data; // value of element } CACHE; CACHE *cache_new(int size); float cache_lookup(CACHE *cache, int i); void cache_insert(CACHE *cache, int i, float x); void cache_clear(CACHE *cache, int i);
Makefile
# the include file in cache_sim.c must be set to select cache implementation rand: sed 's/\#include.*cache\.h/\#include \"random_cache\.h/' cache_sim.c > temp.c gcc -o cache_sim_rand temp.c random_cache.c ./cache_sim_rand tarball: tar cvf cache.tar *.c *.h Makefile clean: rm -f cache_sim_rand temp.c cache.tar *~You will see two C files: cache_sim.c and random_cache.c. Program cache_sim.c models the use of a cached data store. Program random_cache.c implements a cache with a random "cache replacement policy". Read the program to see how it works. The Makefile shows how to compile it. Then answer the following questions f. What is the hit ratio of the cache? (The hit ratio is the fraction of accesses for which there is a cache hit. It can be computed as the number of cache hits divided by the number of accesses, which is the sum of cache hits and cache misses.) You will need to add a print statement to cache sim.c to get this g. The cache size in the program is 10, and the size of the data store is 1000 (look at the constants defined near the top of cache_sim.c). Run the program with cache sizes of 3, 5, 10, 20, and 50. What is the hit ratio in each case? (Note: to run these tests you can either make cache size a command-line argument, or simply edit the CACHE_SIZE value and recompile.) Reset the cache size to 10 after these tests h. Look at the main program. It makes a bunch of memory accesses, always accessing the value at address j. After every access, it modifies j. It does this by randomly selecting a value out of array move_size1, and then adding that value to the current value of j. Within the loop over i in the main program of cache_sim.c, modify move_size1 to move_size2, and rerun the program. What is the new hit ratio value? i. Suppose that in 10,000 accesses, you get 6500 cache hits, and 3500 cache misses. Suppose also that time to perform an access is 0.1 ms if a cache hit, but 10 ms if a cache miss. What is the average time for an access? (Give answer in ms.) j. Repeat the same problem, but assume a poorer caching algorithm is used, and you have 4100 cache hits, and 5900 cache misses. What is the average time for an access in this case? (Give answer in ms.) You will see two C files: cache_sim.c and random_cache.c. Program cache_sim.c models the use of a cached data store. Program random_cache.c implements a cache with a random "cache replacement policy". Read the program to see how it works. The Makefile shows how to compile it. Then answer the following questions f. What is the hit ratio of the cache? (The hit ratio is the fraction of accesses for which there is a cache hit. It can be computed as the number of cache hits divided by the number of accesses, which is the sum of cache hits and cache misses.) You will need to add a print statement to cache sim.c to get this g. The cache size in the program is 10, and the size of the data store is 1000 (look at the constants defined near the top of cache_sim.c). Run the program with cache sizes of 3, 5, 10, 20, and 50. What is the hit ratio in each case? (Note: to run these tests you can either make cache size a command-line argument, or simply edit the CACHE_SIZE value and recompile.) Reset the cache size to 10 after these tests h. Look at the main program. It makes a bunch of memory accesses, always accessing the value at address j. After every access, it modifies j. It does this by randomly selecting a value out of array move_size1, and then adding that value to the current value of j. Within the loop over i in the main program of cache_sim.c, modify move_size1 to move_size2, and rerun the program. What is the new hit ratio value? i. Suppose that in 10,000 accesses, you get 6500 cache hits, and 3500 cache misses. Suppose also that time to perform an access is 0.1 ms if a cache hit, but 10 ms if a cache miss. What is the average time for an access? (Give answer in ms.) j. Repeat the same problem, but assume a poorer caching algorithm is used, and you have 4100 cache hits, and 5900 cache misses. What is the average time for an access in this case? (Give answer in ms.)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
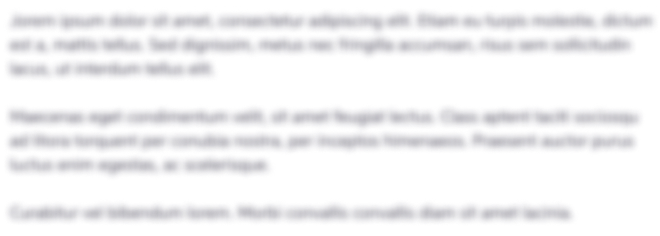
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started