Question
Please help me complete this project. Please Follow the instructions given and code accordingly. Thanks a lot! Programming Project A. Circular linked list game In
Please help me complete this project. Please Follow the instructions given and code accordingly.
Thanks a lot!
Programming Project
A. Circular linked list game
In an ancient land, a King had many prisoners. He decided on the following procedure to determine which prisoner to grand freedom. First, all of the prisoners would be lined up one after the other and assigned numbers. The first prisoner would be number 1, the second number 2, and so on up to the last prisoner, number n. Starting at the prisoner in the first position, he would then count k prisoners down the line, and the kth prisoner would be eliminated from winning her/his freedom removed from the line. The King would then continue, counting k more prisoners, and eliminate every kth prisoner. When he reached the end of the line, he would continue counting from the beginning. For example, if there were six prisoners, the elimination process would proceed as follows (with step k=2):
1->2->3->4->5->6 Initial list of prisoners; start counting from 1.
1->2->4->5->6 Prisoner 3 eliminated; continue counting from 4.
1->2->4->5 Prisoner 6 eliminated; continue counting from 1.
1->2->5 Prisoner 4 eliminated continue counting from 5.
1->5 Prisoner 2 eliminated; continue counting from 5.
1 Prisoner 5 eliminated; 1 is the lucky winner.
Write a program that creates a circular linked list of nodes to determine which position you should stand in to win your freedom if there are n prisoners. Your program should simulate the elimination process by deleting the node that corresponds to the prisoner that is eliminated for each step in the process.
Count the time to create the list, to delete one single node and time to find the winner.
Note: To count the time use system.currentTimeMillis().
Create appropriate JUnits to test your program.
Help with JUnits:
Instructions for developing JUnit:
1.Upon creation of linked list,
1.Check if linked list is empty using assertTrue.
2. Check if length is 0 using assertEquals.
3. Similarly, after adding elements, linked list should not be empty and size not equal to 0.
Sample code:
@Test
public void test() {
linkedList prisoners=new linkedList();
assertTrue(prisoners.isEmpty()); //before inserting, list is empty
assertEquals(0, prisoners.size); // Size is 0
prisoners.insert(5);
assertFalse(prisoners.isEmpty()); // after inserting element, list is not
empty
assertEquals(1,prisoners.size); //size is 1
Test cases:
1. For n={1,2,3,..,6}, k =2,Output:1
2. For n= {1}, k = 9,Output =1
3. n={1,2,...,7}, k =7,Output =4
4. n ={1, 2,...,12}, k =8,Output=2
5. n ={1,2,...,5}, k =1,Output =3
Programming Standards:
Your header comment must describe what your program does.
You must include a comment explaining the purpose of every variable or named constant you use in your program.
You must use meaningful identifier names that suggest the meaning or purpose of the constant, variable, function, etc.
Precede every major block of your code with a comment explaining its purpose. You don't have to describe how it works unless you do something tricky.
You must use indentation and blank lines to make control structures more readable.
Deliverables:
Your main grade will be based on (a) how well your tests cover your own code, (b) how well your code does on your tests (create for all non-trivial methods), and (c) how well your code does on my tests (which you have to add to your test file). For JUnit tests check canvas.
Use cs146S19.
Do not use any fancy libraries. We should be able to compile it under standard installs. Include a readme file on how to compile the project.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
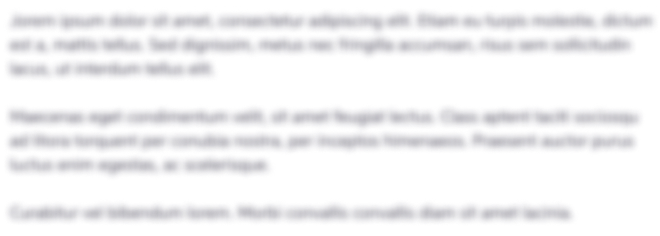
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started