Question
Please help me correct the follow code, I try to make the pause code working, to pause this game. Please let me know where should
Please help me correct the follow code, I try to make the pause code working, to pause this game.
Please let me know where should I add / delete / fix the code.
try:
import random
import time
import pygame
from pygame.color import THECOLORS as COLORS
except:
print("Error, please import correct modules")
else:
pass
# BACKGROUND
W = 800
H = 600
SIZE = 20
# GAME ROW AND COL
ROW = 30
COL = 40
#color set
ORANGE = (255, 128, 0) #snake body color
RED = (255, 0, 0) #snake head color
GREEN = (0, 139, 0) #food color
#
class Point:
def __init__(self, row, col):
self.row = row
self.col = col
def copy(self):
return Point(row=self.row, col=self.col)
# DEFINED DRAW
def rect(point, color):
cell_width = W / COL
cell_height = H / ROW
left = point.col * cell_width
top = point.row * cell_height
pygame.draw.rect(screen, color, (left, top, int(cell_width), int(cell_height)))
# Random create food
def genfood():
while True:
food_x = Point(row=random.randint(0, ROW - 1), col=random.randint(0, COL - 1))
if food_x.row != head.row and food_x.col != head.col and food_x not in snakebody:
return food_x
def draw_pause():
FONT_S = pygame.font.SysFont('Times', 50)
FONT_M = pygame.font.SysFont('Times', 90)
s = pygame.Surface(SIZE,pygame.SRCALPHA)
s.fill(pygame.Color("black"))
screen.blit(s,(0,0))
txt = FONT_M.render('PAUSE',True,pygame.Color('Red'))
W,H = SIZE[0]/2,SIZE[1]/2
W,H = int(W-FONT_M.size('PAUSE')[0]/2),int(H-FONT_M.size('PAUSE')[1]/2)
screen.blit(txt,(W,H))
if __name__ == "__main__":
# pygame init
pygame.init()
screen = pygame.display.set_mode((W,H))
pygame.display.set_caption('Snake Game')
clock = pygame.time.Clock()
# snake body
snakebody = [2]
snakebody_color = ORANGE
# snake head
head = Point(row=0, col=0)
head_color = RED
# food
food = genfood()
food_color = GREEN
# counting score
score = 0
#level
frame = 0.05
level = 1
# direction
direct = 'right'
# while loop
running = True
pause = False
dead = False
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
pause = not pause
elif event.type == pygame.KEYUP:
if event.key == pygame.K_UP:
if direct in ['right','left']:
direct = 'up'
elif event.key == pygame.K_DOWN:
if direct in ['right','left']:
direct = 'down'
elif event.key == pygame.K_LEFT:
if direct in ['up','down']:
direct = 'left'
elif event.key == pygame.K_RIGHT:
if direct in ['up','down']:
direct = 'right'
pause = True
if pause:
draw_pause()
pygame.time.delay(int(frame/level*1000))
# add snake body
snakebody.insert(0, head.copy())
# food that sneak ate
eat = (head.row == food.row and head.col == food.col)
if eat:
food = genfood()
score += 1
else:
if len(snakebody) != 0:
snakebody.pop()
# movement
if not pause and not dead:
if direct == 'up':
head.row -= 1
elif direct == 'down':
head.row += 1
elif direct == 'left':
head.col -= 1
elif direct == 'right':
head.col += 1
# set of dead
dead = False
if head.row < 0 or head.col < 0 or head.row >= ROW or head.col >= COL:
dead = True
for snake in snakebody:
if snake.row == head.row and snake.col == head.col:
dead = True
if dead:
running = False
#
# pygame.draw.rect(screen, (255, 255, 255))
screen.fill(pygame.Color('Wheat'))
# snake head , body, and food.
rect(head, head_color)
for snake in snakebody:
rect(snake, snakebody_color)
rect(food, food_color)
#flip
pygame.display.flip()
#speed of the snake move
clock.tick(10)
else:
print(f"Game Over, your score is :{score}")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
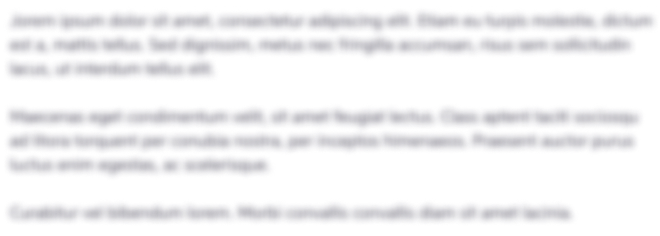
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started