Question
Please help me fix my code, it is supposed to do the following listed below. I don't understand what I am missing. The method can
Please help me fix my code, it is supposed to do the following listed below. I don't understand what I am missing.
The method can take 0, 1 or 2 numbers, and will return their sum (for an empty string it will return 0) for example"" or "1" or "1,2".
- Start with the simplest test case of an empty string and move to 1 and two numbers
- Remember to solve things as simply as possible so that you force yourself to write tests you did not think about
- Remember to refactor after each passing test
- Allow the Add method to handle an unknown amount of numbers
- Calling Add with a negative number will throw an exception "negatives not allowed" - and the negative that was passed.if there are multiple negatives, show all of them in the exception message. There should be a test case that tests for the exception.
- Numbers bigger than 1000 should be ignored, so adding 2 + 1001= 2
- Remember that your input is one string containing 1 or more numbers separated by commas.
Here is my code:
import unittest
import os
import re
negs = []
def get_calculator(input):
symbols = [',']
replace = ''
#findall reference "The Python Standard Library. (n.d.). 7.2."
for match in re.findall(r'(//)(.*)( )', input):
(start, delim_token, end) = match
replace = f'{start}{delim_token}{end}'
symbols = []
for i in re.split(r'\[|\]',delim_token):
if i != "":
symbols.append(i)
return (symbols, replace)
def add(input):
if input == "":
return 0
(calculator, strip) = get_calculator(input)
input = input.replace(strip,'')
for d in calculator:
input = input.replace(d,',')
numbers = re.split(r' |,', input)
sum = 0
for n in numbers:
if n != '':
sum += int(n)
return sum
class StringCalculatorTests(unittest.TestCase):
def test_empty(self):
"""Empty test,"" return 0"""
actual = add('')
self.assertEqual(actual,0)
def test_1(self):
"""Test 1, "1" should return 1"""
actual = add('1')
self.assertEqual(actual, 1)
def test_1_and_2(self):
"""Test 2, "1,2" should return 3"""
actual = add('1,2')
self.assertEqual(actual, 3)
def test_1_newline_2_and_3(self):
"""Test 3, "1\ 2,3" should return 6"""
actual = add('1 2,3')
self.assertEqual(actual, 6)
def test_1_defined_semi (self):
"""Test 4, "//;\ 1;2" should return 3"""
actual = add('//; 1;2')
self.assertEqual(actual, 3)
def test_defined_multi_semi(self):
"""Test 5, "//[***]\ 1***2***3" should return 6"""
actual = add('//[***] 1***2***3')
self.assertEqual(actual, 6)
def test_defined_multi_semi(self):
"""Test 6, " //[*][%]\ 1*2%3" should return -6"""
actual = add('//[*][%] 1*2%3')
self.assertEqual(actual, -6)
if len(negs) > 0:
negStr = ','.join(str(neg) for neg in negs)
raise ValueError('negative value not allowed ' + negStr)
if __name__ == '__main__':
unittest.main()
#References
#The Python Standard Library. (n.d.). 7.2. re Regular expression operations Python v3.1.5 documentation. Retrieved from https://docs.python.org/3.1/library/re.html
#re.findall(pattern, string[, flags])
#spaulk
Step by Step Solution
There are 3 Steps involved in it
Step: 1
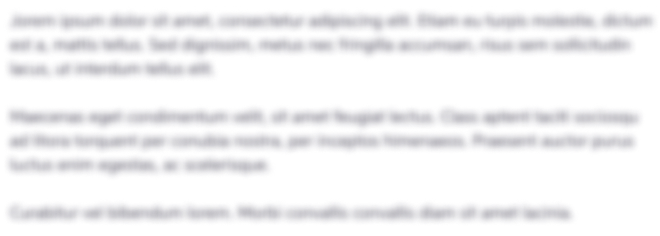
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started