Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please help me implement these functions. Ive included node.hpp and stackinterface #pragma once #include StackInterface.hpp #include Node.hpp #include #include using namespace std; template < class
Please help me implement these functions. Ive included node.hpp and stackinterface
#pragma once #include "StackInterface.hpp" #include "Node.hpp" #include#include using namespace std; template<class T> class DLinkedStack : public StackInterface { private: Node *headPtr; // Pointer to first node in the chain; Node *topPtr; // Pointer to (last) node in the chain that contains the stack's top public: DLinkedStack(); DLinkedStack(const DLinkedStack &aStack); // Copy constructor virtual ~ DLinkedStack(); // Destructor Node *getPointerTo(const T &target) const; bool isEmpty() const; bool push(const T &newItem); bool pop(); T peek() const; vector toVector() const; Node *getHeadPTR() const; Node *getTopPTR() const; }; template<class T> DLinkedStack ::DLinkedStack() : headPtr(nullptr), topPtr(nullptr) { } template<class T> DLinkedStack ::DLinkedStack(const DLinkedStack &aStack) { //TODO - Implement the copy constructor } template<class T> DLinkedStack ::~DLinkedStack() { //TODO - Implement the destructor } template<class T> Node *DLinkedStack ::getPointerTo(const T &target) const { //TODO - Return the Node pointer that contains the target(return nullptr if not found) //when there is more than one target exists, return the first target from top. } template<class T> bool DLinkedStack ::isEmpty() const { //TODO - Return True if the list is empty } template<class T> bool DLinkedStack ::push(const T &newItem) { //TODO - Push an item on the Doubly Linked Stack } template<class T> bool DLinkedStack ::pop() { //TODO - Pop an item from the stack - Return true if successful } template<class T> T DLinkedStack ::peek() const { //Assume this never fails. //TODO - return the element stored at the top of the stack (topPtr) } template<class T> vector DLinkedStack ::toVector() const { // DO NOT MODIFY THIS FUNCTION vector returnVector; // Put stack items into a vector in top to bottom order Node *curPtr = topPtr; while (curPtr != nullptr) { returnVector.push_back(curPtr->getItem()); curPtr = curPtr->getPrev(); } return returnVector; } template<class T> Node *DLinkedStack ::getHeadPTR() const { // DO NOT MODIFY THIS FUNCTION return headPtr; } template<class T> Node *DLinkedStack ::getTopPTR() const { // DO NOT MODIFY THIS FUNCTION return topPtr; }
Node file
#pragma once template<class T> class Node { private: T data; Node*prev; Node *next; public: Node(); Node(const T &anItem); Node(const T &anItem, Node *prevNodePtr, Node *nextNodePtr); void setItem(const T &anItem); void setPrev(Node *prevNodePtr); void setNext(Node *nextNodePtr); T getItem() const; Node *getPrev() const; Node *getNext() const; }; template<class T> Node ::Node() : data(0), prev(nullptr), next(nullptr) { } template<class T> Node ::Node(const T &anItem) : data(anItem), prev(nullptr), next(nullptr) { } template<class T> Node ::Node(const T &anItem, Node *prevNodePtr, Node *nextNodePtr) : data(anItem), prev(prevNodePtr), next(nextNodePtr) { } template<class T> void Node ::setItem(const T &anItem) { data = anItem; } template<class T> void Node ::setPrev(Node *prevNodePtr) { prev = prevNodePtr; } template<class T> void Node ::setNext(Node *nextNodePtr) { next = nextNodePtr; } template<class T> T Node ::getItem() const { return data; } template<class T> Node *Node ::getPrev() const { return prev; } template<class T> Node *Node ::getNext() const { return next; }
Stack interface
#pragma once template<class T> class StackInterface { public: /** Sees whether this stack is empty. @return True if the stack is empty, or false if not. */ virtual bool isEmpty() const = 0; /** Adds a new entry to the top of this stack. @post If the operation was successful, newEntry is at the top of the stack. @param newEntry The object to be added as a new entry. @return True if the addition is successful or false if not. */ virtual bool push(const T &newEntry) = 0; /** Removes the top of this stack. @post If the operation was successful, the top of the stack has been removed. @return True if the removal is successful or false if not. */ virtual bool pop() = 0; /** Returns the top of this stack. @pre The stack is not empty. @post The top of the stack has been returned, and the stack is unchanged. @return The top of the stack. */ virtual T peek() const = 0; }; // end StackInterface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
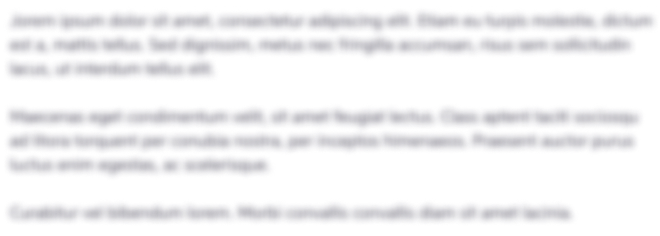
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started