Question
Please help me on all the questions !!!!!!!! Really need help! Will give a thumb up for helping. True/False (13) Chapter 14 - A List
Please help me on all the questions !!!!!!!! Really need help! Will give a thumb up for helping.
True/False (13)
Chapter 14 - A List Implementation that Links Data
-
Adding a node to an empty chain is the same as adding a node to the beginning of a chain.
-
Adding a node at the end of a chain of n nodes is the same as adding a node at position n.
-
You need a temporary variable to reference nodes as you traverse a list.
-
The efficiency of the displayList method is directly related to the efficiency of the getEntry
method.
-
You cannot use an assert statement to determine of a list is empty.
-
A fundamental operation of a list is a traversal.
-
You must know how much memory to allocate before creating a linked implementation of a list.
-
A linked implementation of a list grows and shrinks dynamically as nodes are added and deleted.
-
In a linked implementation of a list, you only call the method getNodeAt when we remove an
entry other than the first one.
-
Replacing a node in a linked implementation of a list only requires locating the node and
replacing the data portion of the node.
-
In a linked implementation of a list, the replace method replaces the entire node.
-
Retrieving a list entry using a linked implementation is faster than using an array representation.
-
Replacing a list entry using an array implementation is faster than using a linked representation.
Short Answer (6)
-
Explain why a public method should be declared to be final if it is called by a constructor.
-
In the linked implementation of a list, why shouldnt the constructor call the method clear even
though they do the same exact assignment statements?
-
Outline the basic steps to add a node to the end of a linked implementation of a list.
-
Outline the basic steps to add a node to the beginning of an empty linked implementation of a
list.
-
Outline the basic steps to add a node to the beginning of a non-empty linked implementation of
a list.
-
Outline the basic steps to remove a node from the beginning of a list.
Multiple Choice (30)
1. A linked implementation of a list
-
uses memory only as need for new entries
-
returns unneeded memory to the system when an entry is removed
-
avoids moving data when adding or removing entries
-
all of the above
-
In a chain of linked nodes you can
-
add nodes from the beginning of a chain
-
add nodes from the end of a chain
-
add nodes that are between other nodes
-
all of the above
-
-
In a chain of linked nodes you can
-
remove nodes from the beginning of a chain
-
remove nodes from the end of a chain
-
remove nodes that are between other nodes
-
all of the above
-
-
If a chain is empty, the head reference
-
is null
-
is an empty node with the data field not set
-
is in an illegal state
-
none of the above
-
-
When
-
the node before the insertion position and the node after the insertion position
-
the first node and the node before the insertion position
-
the first node and the node after the insertion position
-
the last node and the node after the insertion position
-
-
Adding a node at the end of a chain of n nodes is the same as adding a node at position a. n+1
b. n c. n1 d. 0
-
If the reference to the first node is null, this means
-
the list is empty
-
the list is full
-
the garbage collector should be invoked
-
the list is in an unstable state
-
-
The last node is a list has a reference to
-
null
-
itself
-
the node before it
-
none of the above
-
inserting a node between adjacent nodes in a chain you must locate
9. To locate a node within a chain that is near the end of the list, we must
-
start at the first node and traverse the chain
-
start at the last node and traverse the chain
-
directly access the node
-
none of the above
10. Moving a. traversing
through a linked implementation of a list from one node to another is called
-
walking
-
hopping
-
none of the above
11. In the LList implementation of a list, when a list is empty the firstNode is _____ and the numberOfEntries is _____.
a. b. c. d.
-
In the a. b. c. d.
-
In the
null, 0 null, 1 an empty node, 0 an empty node, 1
LList implementation of a list, the constructor and the clear method have the same functionality do completely different things unnecessary
none of the above
linked implementation of a list, the add method public void add(T newEntry)
inserts a new entry a. at the end of the list b. at the beginning of the list c. between adjacent nodes of the list d. all of the above
14. In the linked implementation of a list, the add method public void add(int newPosition, T newEntry)
inserts a new entry
-
between adjacent nodes of the list
-
at the end of the list
-
at the beginning of the list
-
all of the above
-
In the linked implementation of a list, what happens when you try to add a node at an invalid position?
-
it throws an IndesOutOfBoundsException
-
it throws an InvalidLinkException
-
it returns null
-
it returns false
-
-
To determine if a list is empty you can a. check to see if the length of the list is zero b. check to see if the reference to firstNode is null c. use an assertion on firstNode == null d. all of the above **
-
In the LList implementation of a list, given a node called currentNode, which statement moves
the nodes reference to the next node?
a. currentNode.getNextNode(); b. currentNode.get(); c. currentNode.forward(); d. currentNode.traverse();
-
A reference to the last node in a linked implementation of a list is commonly called the
-
tail
-
end
-
final
-
none of the above
-
-
Including a tail reference to a linked implementation of a list makes which functionality more efficient?
-
adding a node to the end of a list
-
searching for a node on the list
-
removing a node from the list
-
all of the above
-
-
In a linked implementation of a list with a tail reference, removing an entry affects the tail reference if
-
the list only contains one entry
-
the list is empty
-
the list is full
-
all of the above
-
-
In a linked implementation of a list with a tail reference, removing an entry affects the tail reference if
-
the list has multiple entries and the entry to be removed is the last one
-
the list has multiple entries and the entry to be removed is the first one
-
the list is empty
-
all of the above
-
-
In a linked-based implementation of the ADT list with only a head reference, what is the performance of adding an entry at the end of the list?
-
O(n)
-
O(n2)
-
O(log n)
-
O(1)
-
-
In a linked-based implementation of the ADT list with a tail reference, what is the performance of adding an entry at the end of the list?
-
O(1)
-
O(log n)
-
O(n)
-
O(n2)
-
-
In a linked-based implementation of the ADT list with a tail reference, what is the performance of adding an entry that is not at the beginning of the list?
-
O(n)
-
O(n2)
-
O(log n)
-
O(1)
-
-
In a linked-based implementation of the ADT list with only a head reference, what is the performance of removing an entry at the beginning of the list?
-
O(1)
-
O(log n)
-
O(n)
-
O(n2)
-
-
In a linked-based implementation of the ADT list with only a head reference, what is the performance of removing an entry at the end of the list?
-
O(n)
-
O(n2)
-
O(log n)
-
O(1)
-
-
In a linked-based implementation of the ADT list with a tail reference, what is the performance of removing an entry at the beginning of the list?
-
O(1)
-
O(log n)
-
O(n)
-
O(n2)
-
-
In a linked-based implementation of the ADT list with a tail reference, what is the performance of removing an entry that is not at the beginning of the list?
-
O(n)
-
O(n2)
-
O(log n)
-
O(1)
-
-
In a linked-based implementation of the ADT list with only a head reference, which method has a time efficiency of O(1)?
-
clear
-
toArray
-
contains
-
all of the above
-
-
In a linked-based implementation of the ADT list with a tail reference, which method has a time efficiency of O(1)?
-
adding an entry to the end of the list
-
adding an entry to the beginning of the list
-
clear
-
all of the above
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
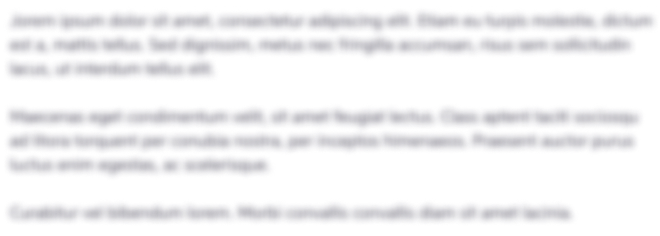
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started