Question
Please help me on this question in Java, other answer on chegg doesn't work. Thank you so much Question: Create a simple game of mine
Please help me on this question in Java, other answer on chegg doesn't work. Thank you so much
Question:
Create a simple game of mine sweeper with the following classes and a driver
Download the driver
Put the driver in your project
DO NOT ALTER THE DRIVER!
Write a class file called MineSpace
Attributes of this class
Selected determines whether or not this space has been clicked on
IsAMine this is whether or not it is a mine
SurroundingMines The number of mines surrounding that space
Create a default constructor with the following
Selected is set to false
IsAMine is randomly set to either true or false
Create Accessors and Mutators for each instance variable
MAKE SURE TO CHECK FOR VALID VALUES
Write another class SimpleMineSweeperBackEnd
Attributes of this class are
Spaces a 2D array of type mine space
SPACE_AMT a constant value set to 5 as this is a 5x5 minefield
numNotMinesLeft the number of mines left on the board
gameOver whether or not the game is over
win whether or not the player wins
Create a default constructor
Declare the 2D array
Call reset spaces
Create the following Methods
resetSpaces this method returns nothing and takes no parameters constructs each individual space and counts the number of not mines. It also sets each mine surroundingMines value by looking at each of its neighbors (HINT: looking setting the number of mines value maybe best done in a private method). Also it sets both gameOver and win to false.
getNumNotMinesLeft returns how many spaces that arent mines remain
getGameOver returns whether or not the game is over
getWin returns whether or not the player has won
printMines prints each mine space in the 2D array. It should print whether or not it is a mine and the number of surrounding mines. This is really good for debugging.
selectSpace this method which returns an instance of Space takes in two integers corresponding to the button that was selected. Much like other projects the x increase left to right and the y increases from top to bottom. This method should check if it is already select. If it hasnt been then set selected to true. Next check if that space is a mine. If it is then set gameOver to true and win to false. If not then subtract the number of not mines left. Next check if the number of not mines is 0 then game over should be set to true and win should be set to true.
A Driver:
import java.awt.Color;
/** * * @author w00t */ public class SimpleMineSweeperFrontEnd extends javax.swing.JFrame {
/** * Creates new form NewJFrame */ SimpleMineSweeperBackEnd backEnd; public SimpleMineSweeperFrontEnd() { initComponents(); backEnd = new SimpleMineSweeperBackEnd(); }
/** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // //GEN-BEGIN:initComponents private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane(); jTable1 = new javax.swing.JTable(); jButton1 = new javax.swing.JButton(); jButton2 = new javax.swing.JButton(); jButton3 = new javax.swing.JButton(); jButton4 = new javax.swing.JButton(); jButton5 = new javax.swing.JButton(); jButton6 = new javax.swing.JButton(); jButton7 = new javax.swing.JButton(); jButton8 = new javax.swing.JButton(); jButton9 = new javax.swing.JButton(); jButton10 = new javax.swing.JButton(); jButton11 = new javax.swing.JButton(); jButton12 = new javax.swing.JButton(); jButton13 = new javax.swing.JButton(); jButton14 = new javax.swing.JButton(); jButton15 = new javax.swing.JButton(); jButton16 = new javax.swing.JButton(); jButton17 = new javax.swing.JButton(); jButton18 = new javax.swing.JButton(); jButton19 = new javax.swing.JButton(); jButton20 = new javax.swing.JButton(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jButton21 = new javax.swing.JButton();
jTable1.setModel(new javax.swing.table.DefaultTableModel( new Object [][] { {null, null, null, null}, {null, null, null, null}, {null, null, null, null}, {null, null, null, null} }, new String [] { "Title 1", "Title 2", "Title 3", "Title 4" } )); jScrollPane1.setViewportView(jTable1);
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } });
jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } });
jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } });
jButton4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton4ActionPerformed(evt); } });
jButton5.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton5ActionPerformed(evt); } });
jButton6.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton6ActionPerformed(evt); } });
jButton7.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton7ActionPerformed(evt); } });
jButton8.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton8ActionPerformed(evt); } });
jButton9.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton9ActionPerformed(evt); } });
jButton10.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton10ActionPerformed(evt); } });
jButton11.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton11ActionPerformed(evt); } });
jButton12.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton12ActionPerformed(evt); } });
jButton13.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton13ActionPerformed(evt); } });
jButton14.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton14ActionPerformed(evt); } });
jButton15.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton15ActionPerformed(evt); } });
jButton16.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton16ActionPerformed(evt); } });
jButton17.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton17ActionPerformed(evt); } });
jButton18.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton18ActionPerformed(evt); } });
jButton19.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton19ActionPerformed(evt); } });
jButton20.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton20ActionPerformed(evt); } });
jLabel1.setText("Mines:");
jLabel2.setText("0");
jLabel3.setText(" ");
jButton21.setText("Game Over"); jButton21.setEnabled(false); jButton21.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton21ActionPerformed(evt); } });
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton5, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jLabel1) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 46, javax.swing.GroupLayout.PREFERRED_SIZE)) .addComponent(jLabel3) .addComponent(jButton21))) .addGroup(layout.createSequentialGroup() .addComponent(jButton6, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton7, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton8, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton9, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton10, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addComponent(jButton11, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton12, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton13, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton14, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton15, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addComponent(jButton16, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton17, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton18, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton19, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton20, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton5, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel1) .addComponent(jLabel2)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jLabel3) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton21))) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton6, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton7, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton8, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton9, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton10, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton11, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton12, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton13, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton14, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton15, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton16, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton17, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton18, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton19, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton20, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE))) );
pack(); }// //GEN-END:initComponents
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed // TODO add your handling code here: buttonCheck(jButton1,0); }//GEN-LAST:event_jButton1ActionPerformed
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton2ActionPerformed // TODO add your handling code here: buttonCheck(jButton2,1); }//GEN-LAST:event_jButton2ActionPerformed
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton3ActionPerformed // TODO add your handling code here: buttonCheck(jButton3,2); }//GEN-LAST:event_jButton3ActionPerformed
private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton4ActionPerformed // TODO add your handling code here: buttonCheck(jButton4,3); }//GEN-LAST:event_jButton4ActionPerformed
private void jButton5ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton5ActionPerformed // TODO add your handling code here: buttonCheck(jButton5,4); }//GEN-LAST:event_jButton5ActionPerformed
private void jButton6ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton6ActionPerformed // TODO add your handling code here: buttonCheck(jButton6,5); }//GEN-LAST:event_jButton6ActionPerformed
private void jButton7ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton7ActionPerformed // TODO add your handling code here: buttonCheck(jButton7,6); }//GEN-LAST:event_jButton7ActionPerformed
private void jButton8ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton8ActionPerformed // TODO add your handling code here: buttonCheck(jButton8,7); }//GEN-LAST:event_jButton8ActionPerformed
private void jButton9ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton9ActionPerformed // TODO add your handling code here: buttonCheck(jButton9,8); }//GEN-LAST:event_jButton9ActionPerformed
private void jButton10ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton10ActionPerformed // TODO add your handling code here: buttonCheck(jButton10,9); }//GEN-LAST:event_jButton10ActionPerformed
private void jButton11ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton11ActionPerformed // TODO add your handling code here: buttonCheck(jButton11,10); }//GEN-LAST:event_jButton11ActionPerformed
private void jButton12ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton12ActionPerformed // TODO add your handling code here: buttonCheck(jButton12,11); }//GEN-LAST:event_jButton12ActionPerformed
private void jButton13ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton13ActionPerformed // TODO add your handling code here: buttonCheck(jButton13,12); }//GEN-LAST:event_jButton13ActionPerformed
private void jButton14ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton14ActionPerformed // TODO add your handling code here: buttonCheck(jButton14,13); }//GEN-LAST:event_jButton14ActionPerformed
private void jButton15ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton15ActionPerformed // TODO add your handling code here: buttonCheck(jButton15,14); }//GEN-LAST:event_jButton15ActionPerformed
private void jButton16ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton16ActionPerformed // TODO add your handling code here: buttonCheck(jButton16,15); }//GEN-LAST:event_jButton16ActionPerformed
private void jButton17ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton17ActionPerformed // TODO add your handling code here: buttonCheck(jButton17,16); }//GEN-LAST:event_jButton17ActionPerformed
private void jButton18ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton18ActionPerformed // TODO add your handling code here: buttonCheck(jButton18,17); }//GEN-LAST:event_jButton18ActionPerformed
private void jButton19ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton19ActionPerformed // TODO add your handling code here: buttonCheck(jButton19,18); }//GEN-LAST:event_jButton19ActionPerformed
private void jButton20ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton20ActionPerformed // TODO add your handling code here: buttonCheck(jButton20,19); }//GEN-LAST:event_jButton20ActionPerformed
private void jButton21ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton21ActionPerformed // TODO add your handling code here: resetAllButtons(); }//GEN-LAST:event_jButton21ActionPerformed private void buttonCheck(javax.swing.JButton aButton, int index) { if(backEnd.getGameOver()) return; int i = index / SimpleMineSweeperBackEnd.SPACE_AMT; int j = index % SimpleMineSweeperBackEnd.SPACE_AMT; System.out.println(i+" "+j); MineSpace space = backEnd.selectSpace(i,j); jLabel2.setText(backEnd.getNumNotMinesLeft()+""); if(space.isIsAMine() == false) { aButton.setBackground(Color.green); aButton.setText(space.getSurroundingMines()+""); } else aButton.setBackground(Color.red); if(backEnd.getGameOver()) { jButton21.setEnabled(true); if(backEnd.getWin()) { jLabel3.setText("WINNER!"); } else { jLabel3.setText("LOSER!"); } } } private void resetAllButtons() { jButton1.setBackground(Color.lightGray); jButton2.setBackground(Color.lightGray); jButton3.setBackground(Color.lightGray); jButton4.setBackground(Color.lightGray); jButton5.setBackground(Color.lightGray); jButton6.setBackground(Color.lightGray); jButton7.setBackground(Color.lightGray); jButton8.setBackground(Color.lightGray); jButton9.setBackground(Color.lightGray); jButton10.setBackground(Color.lightGray); jButton11.setBackground(Color.lightGray); jButton12.setBackground(Color.lightGray); jButton13.setBackground(Color.lightGray); jButton14.setBackground(Color.lightGray); jButton15.setBackground(Color.lightGray); jButton16.setBackground(Color.lightGray); jButton17.setBackground(Color.lightGray); jButton18.setBackground(Color.lightGray); jButton19.setBackground(Color.lightGray); jButton20.setBackground(Color.lightGray); jButton1.setText(""); jButton2.setText(""); jButton3.setText(""); jButton4.setText(""); jButton5.setText(""); jButton6.setText(""); jButton7.setText(""); jButton8.setText(""); jButton9.setText(""); jButton10.setText(""); jButton11.setText(""); jButton12.setText(""); jButton13.setText(""); jButton14.setText(""); jButton15.setText(""); jButton16.setText(""); jButton17.setText(""); jButton18.setText(""); jButton19.setText(""); jButton20.setText(""); backEnd.resetSpaces(); jLabel2.setText(backEnd.getNumNotMinesLeft()+""); jLabel3.setText(""); } /** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ // /* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel. * For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html */ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(SimpleMineSweeperFrontEnd.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(SimpleMineSweeperFrontEnd.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(SimpleMineSweeperFrontEnd.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(SimpleMineSweeperFrontEnd.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } // // // // // // // // /* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new SimpleMineSweeperFrontEnd().setVisible(true); } }); }
// Variables declaration - do not modify//GEN-BEGIN:variables private javax.swing.JButton jButton1; private javax.swing.JButton jButton10; private javax.swing.JButton jButton11; private javax.swing.JButton jButton12; private javax.swing.JButton jButton13; private javax.swing.JButton jButton14; private javax.swing.JButton jButton15; private javax.swing.JButton jButton16; private javax.swing.JButton jButton17; private javax.swing.JButton jButton18; private javax.swing.JButton jButton19; private javax.swing.JButton jButton2; private javax.swing.JButton jButton20; private javax.swing.JButton jButton21; private javax.swing.JButton jButton3; private javax.swing.JButton jButton4; private javax.swing.JButton jButton5; private javax.swing.JButton jButton6; private javax.swing.JButton jButton7; private javax.swing.JButton jButton8; private javax.swing.JButton jButton9; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; private javax.swing.JScrollPane jScrollPane1; private javax.swing.JTable jTable1; // End of variables declaration//GEN-END:variables }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
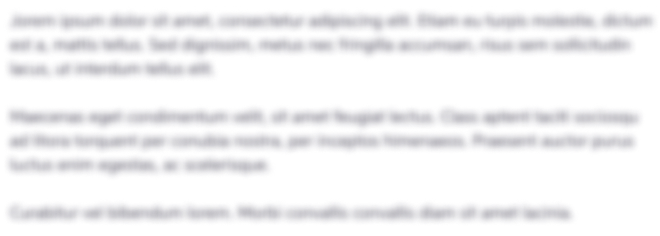
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started