Question
Please help me the find my error in my Python code. I cant print out the right output of distance.Here is my code: # This
Please help me the find my error in my Python code. I cant print out the right output of distance.Here is my code: # This program can be used to search for places of interest near a given # location. Read the give_intro method for more details. It depends on a file # called places.txt for the data. from place_information import * from geolocator import * def main(): give_intro() lines = open("places.txt").readlines() data = parse_file(lines) place = input("target? ") target = GeoLocator.find_place(place) if (target == None): print("no matches for that search string.") else: print("found " + target.get_name()) show_matches(data, target) # introduces the program to the user def give_intro(): print("This program allows you to search for places of") print("interest near a target location. For each") print("search, you will be asked for a target radius,") print("and a search string for name and tags. If you") print("don't want to filter on name and/or tag, simply") print("hit enter to see all results.") print() # Parses data from the given file to construct a list of # PlaceInformation objects. Assumes the data is in standard format # (tab-delimited lines with name, address, tag, latitude, longitude). def parse_file(lines): entries = [] # put the code here to go thru each line in lines and split using tab as the delimeter and create a PlaceInformation object for each line and store in the list entries for line in lines: line = line.split("\t") name = line[0] address = line[1] tag = line[2] latitude = float(line[3]) longitude = float(line[4]) information = PlaceInformation(name, address, tag, latitude, longitude) entries.append(information) return entries # Prompts the user for a radius and search strings and shows matching # entries from data and their distance from the given target. def show_matches(data, target): radius = 42 # not a real radius, priming the while loop while (radius > 0): print() radius = float(input("radius (0 to quit)? ")) if (radius > 0): show_entries(data, target, radius) # Prompts the user for search strings and shows matching entries from data # that are within the given distance of the given target. def show_entries(data, target, radius): name_filter = input("name filter (enter for none)? ") tag_filter = input("tag filter (enter for none)? ") for entry in data: name_match = match(entry.get_name(), name_filter) tag_match = match(entry.get_tag(), tag_filter) if (name_match and tag_match): distance = entry.distance_from(target.get_location()) print("distance: ", distance) if (float(distance) <= radius): # <= not supported between instances of str and float print(str(distance) + " miles: " + entry.get_name() + " " + entry.get_address()) # returns true if the bigger string contains the smaller string ignoring # case def match(big, small): return small.lower() in big.lower() main()
my output:
This program allows you to search for places of interest near a target location. For each search, you will be asked for a target radius, and a search string for name and tags. If you don't want to filter on name and/or tag, simply hit enter to see all results.
target?
expected output:
This program allows you to search for places of interest near a target location. For each search, you will be asked for a target radius, and a search string for name and tags. If you don't want to filter on name and/or tag, simply hit enter to see all results.
target? found Atlanta
radius (0 to quit)? name filter (enter for none)? tag filter (enter for none)? distance: 163.14685011543787 163.14685011543787 miles: Spill the Beans Coffee Shop 174 E Main St, Spartanburg
geolocator.py
import urllib import urllib.request import urllib.parse import xml.etree.ElementTree as et from place_information import * from geolocation import * class GeoLocator: # Given a query string, returns a GeoLocation representing the coordinates # of Google's best guess at what the query string represents. # # Returns None if there are no results for the given query, or if we # cannot connect to the Maps API. @staticmethod def find(location): root = GeoLocator.__get_xml(location) if(root == None): return None result = root.find('result').find('geometry').find('location') lat = float(result.find('lat').text) lng = float(result.find('lat').text) return GeoLocation(lat, lng) # Given a query string, returns a PlaceInformation representing the data # of Google's best guess at what the query string represents. # # Returns None if there are no results for the given query, or if we # cannot connect to the Maps API. @staticmethod def find_place(location): root = GeoLocator.__get_xml(location) if (root == None): return None result = root.find('result').find('geometry').find('location') lat = float(result.find('lat').text) lng = float(result.find('lat').text) address = root.find('result').find('formatted_address').text name = root.find('result').find('address_component').find('long_name').text # tag = " ".join(root.find('result').findall('type')) tag = root.find('result').find('type').text return PlaceInformation(name, address, tag, lat, lng) # takes a location string to search the Google Maps API for as a parameter # returns an XML object with data about the search @staticmethod def __get_xml(location): url_string = ("https://maps.googleapis.com/maps/api/geocode/xml?sensor=false&address=" + urllib.parse.quote_plus(location) + "&key=APIKEY") # i have the key but cant share try: # Get the first result in the XML document, bail if no results f = urllib.request.urlopen(url_string) xml = f.read() root = et.fromstring(xml) result = root.find('result').find('geometry').find('location') if (None == result or "" == result): # No results found for query. return None return root except RuntimeError: print("Error") # Given any failures trying to retrieve results... return None
place_ifnformation.py
from geolocation import GeoLocation class PlaceInformation: def __init__(self, name, address, tag, latitude, longitude): self.__name = name self.__address = address self.__tag = tag self.__latitude = latitude self.__longitude = longitude self.__location = GeoLocation(latitude, longitude) def get_name(self): return self.__name def get_address(self): return self.__address def get_tag(self): return self.__tag def get_location(self): return self.__location; def __str__(self): return self.__name + '(latitude:' + str(self.__latitude) + ',longitude:' + str(self.__longitude) + ')' def distance_from(self, spot): distance = self.get_location().distance_from(spot) return str(distance)
geolocation.py
from math import * RADIUS = 3963.1676 # Earth radius in miles class GeoLocation: # constructs a geo location object with given latitude and longitude def __init__(self, latitude, longitude): self.__latitude = float(latitude) self.__longitude = float(longitude) # returns the latitude of this geo location def get_latitude(self): return self.__latitude # returns the longitude of this geo location def get_longitude(self): return self.__longitude # returns a string representation of this geo location def __str__(self): return "latitude: " + str(self.__latitude) + ", longitude: " + str(self.__longitude) # WRITE THIS METHOD FOR AN A # returns the distance in miles between this geo location and the given # other geo location # # YOU NEED TO WRITE THIS METHOD; EMAIL ME IF YOU CANNOT FIGURE IT OUT; YOUR GRADE WILL BE LOWER def distance_from(self, other): latitude1 = radians(self.__latitude) longitude1 = radians(self.__longitude) latitude2 = radians(other.__latitude) longitude2 = radians(other.__longitude) # apply the spherical law of cosines with a triangle composed of the # two locations and the north pole cosin = sin(latitude1) * sin(latitude2) + cos(latitude1) * cos(latitude2) * cos(longitude1 - longitude2) theAnswer = acos(cosin) return theAnswer * RADIUS
Step by Step Solution
There are 3 Steps involved in it
Step: 1
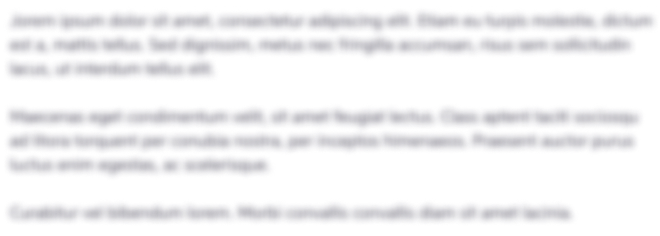
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started