PLEASE HELP ME TO ANSWER THOSE QUESTIONS PLEASE AND THANK YOU
Do Not allow your lines to be longer than the line of "=" characters. ======================================================================== 1. What is the effect of declaring a class to be abstract?
======================================================================== 2. What is the effect of declaring methods to be abstract?
======================================================================== 3. How many classes can one class extend?
======================================================================== 4. How many Interfaces can one class implement?
======================================================================== 5. Can an abstract class be instantiated?
======================================================================== 6. What was the hardest part of this lab for you and why was it hard.
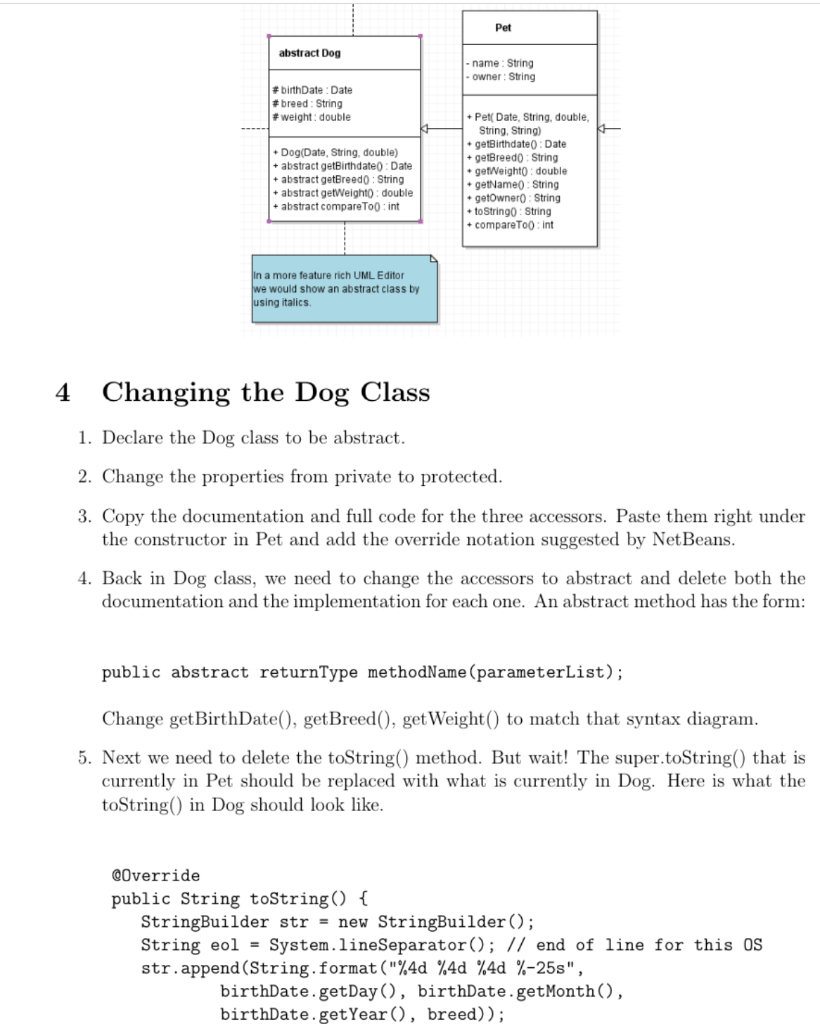
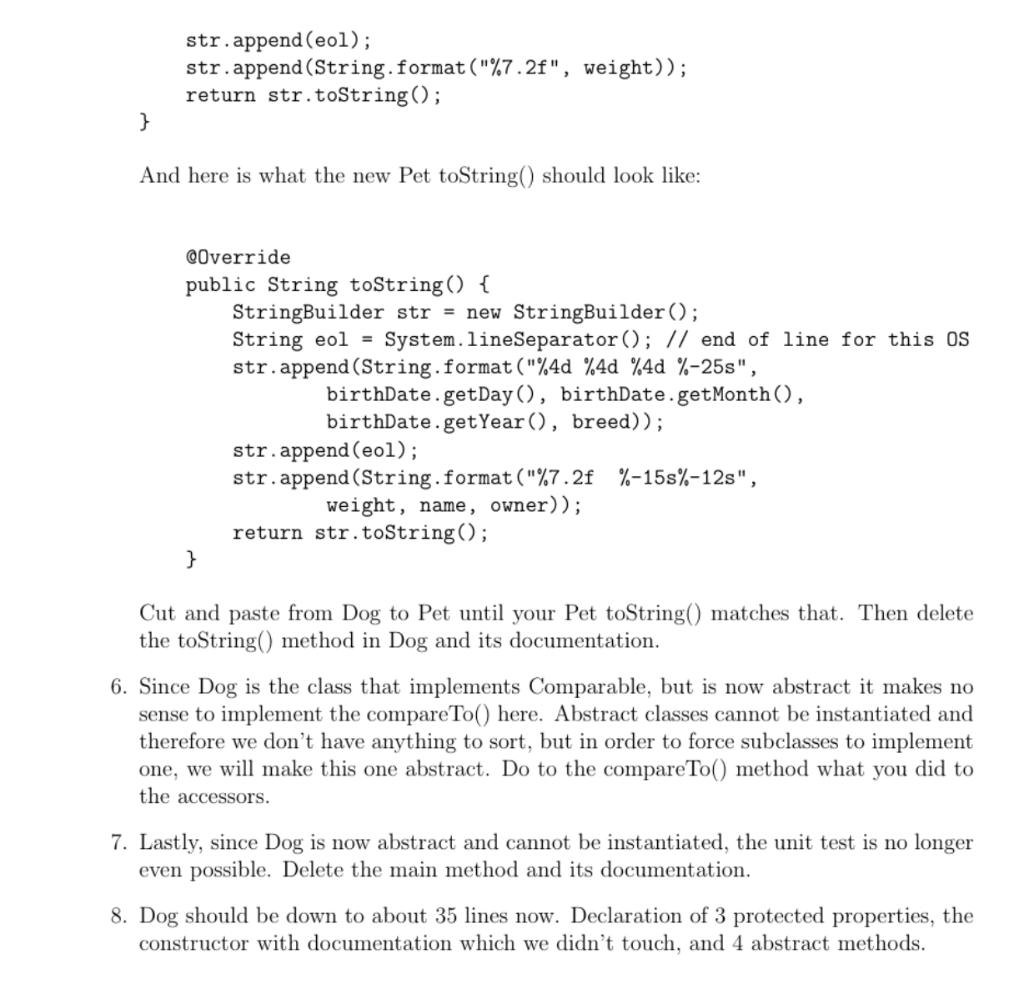
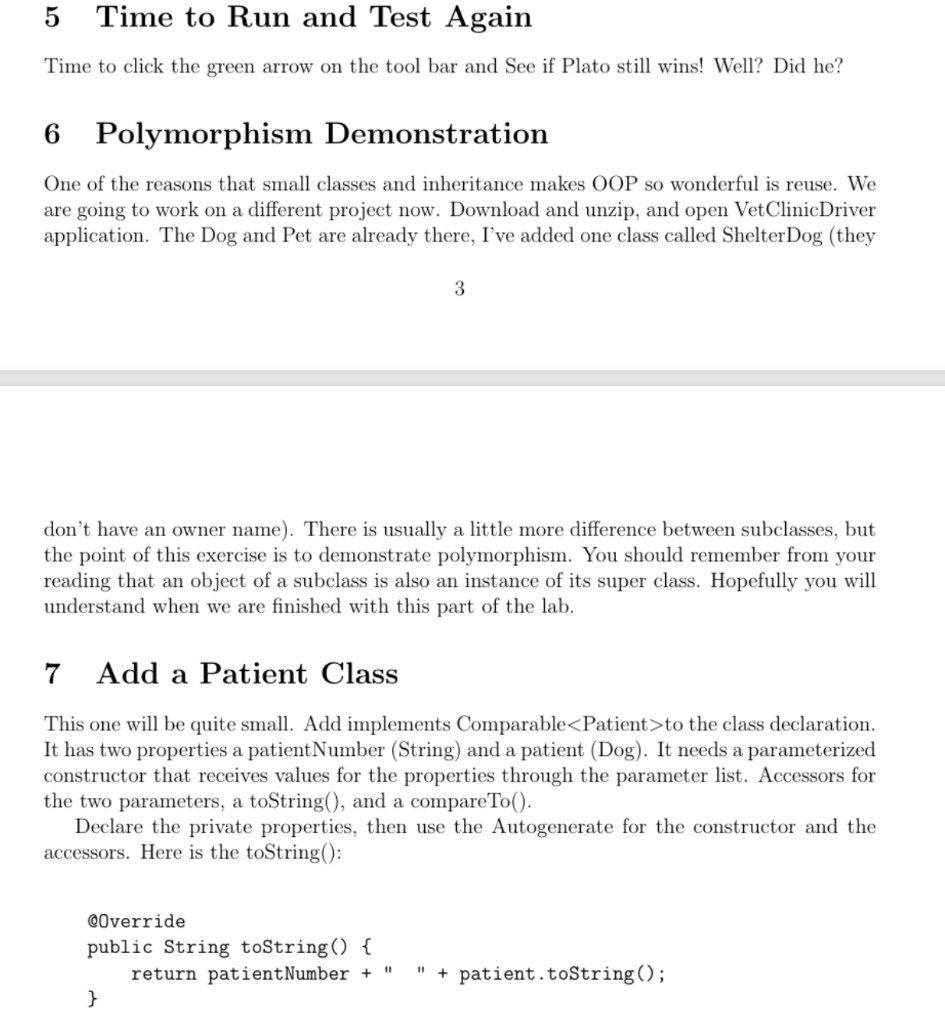
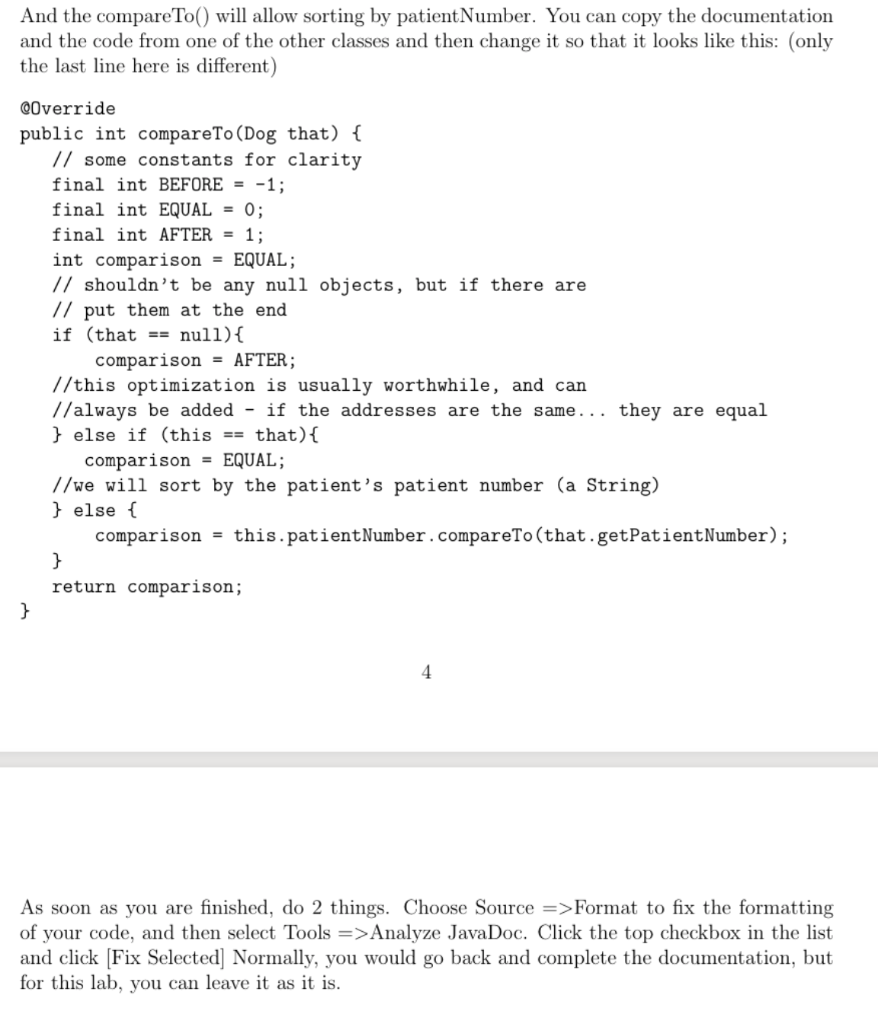
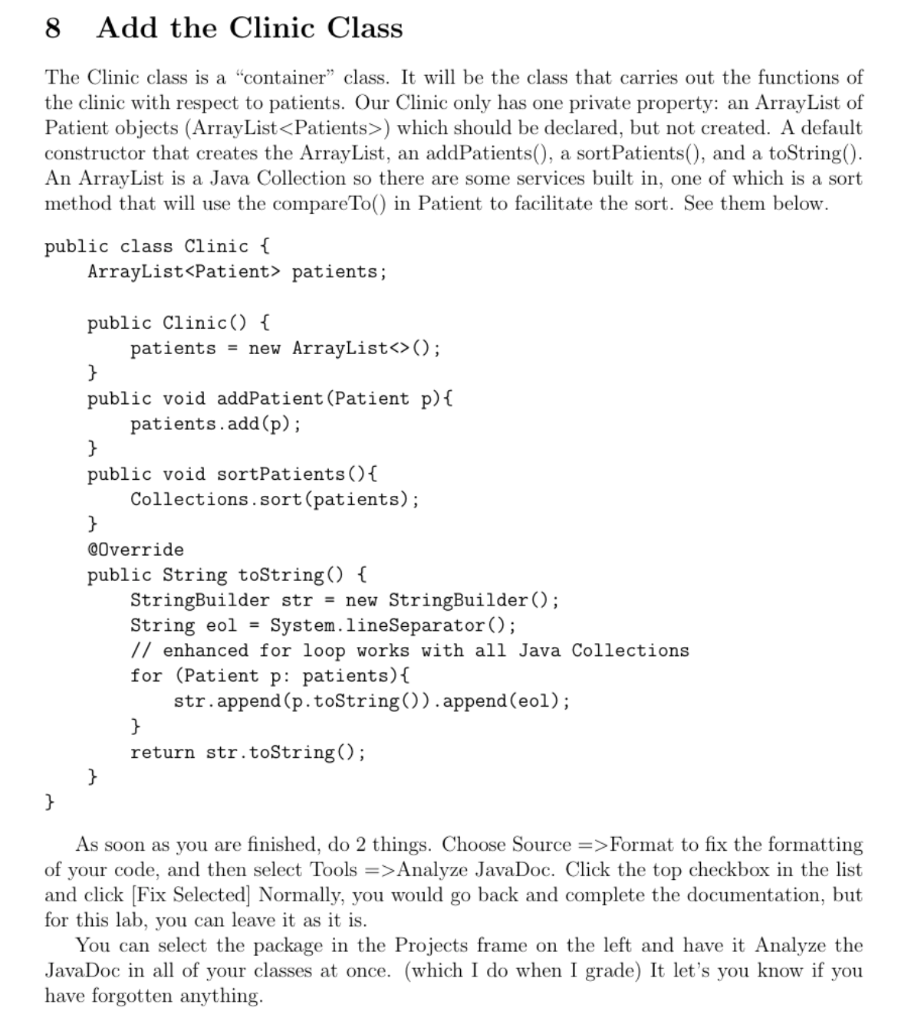
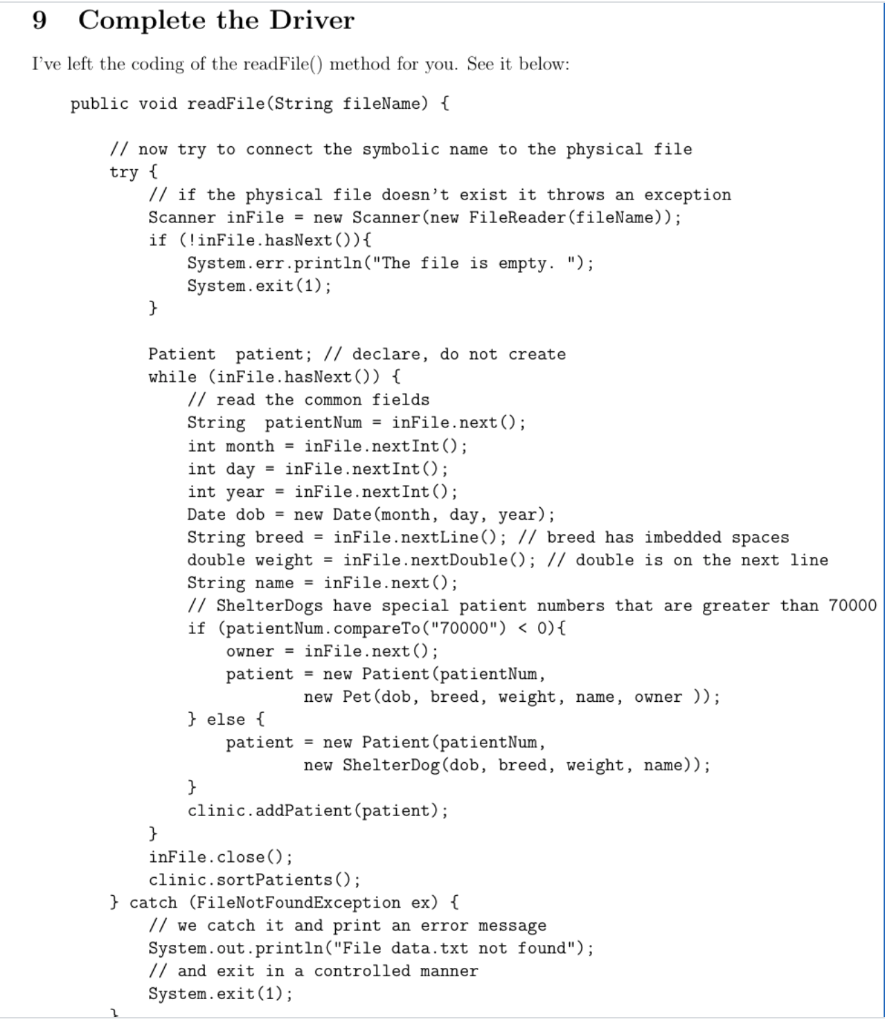
Pet abstract Dog - name: String - owner: String #birthDate: Date #breed: String #weight: double + Dog(Date, String, double) + abstract getBirthdate(): Date + abstract getBreedo: String + abstract getWeight): double + abstract compare Toint + Petr Date. String, double, String, String) * GetBirthdate(): Date + getBreed String .getWeight: double - getName(): String .getOwner : String to String : String + compareToo: int In a more feature rich UML Editor we would show an abstract class by using italics. 4 Changing the Dog Class 1. Declare the Dog class to be abstract. 2. Change the properties from private to protected. 3. Copy the documentation and full code for the three accessors. Paste them right under the constructor in Pet and add the override notation suggested by NetBeans. 4. Back in Dog class, we need to change the accessors to abstract and delete both the documentation and the implementation for each one. An abstract method has the form: public abstract returnType methodName (parameterList); Change get BirthDate(), getBreed(), get Weight() to match that syntax diagram. 5. Next we need to delete the toString() method. But wait! The super.toString() that is currently in Pet should be replaced with what is currently in Dog. Here is what the toString() in Dog should look like. @Override public String toString() { StringBuilder str = new StringBuilder(); String eol = System.lineSeparator(); // end of line for this os str.append(String.format("%40 %40 %40 %-25s", birthDate.getDay(), birthDate.getMonth(), birthDate.getYear(), breed)); str.append(eol); str.append(String.format("%7.2f", weight)); return str.toString(); } And here is what the new Pet toString() should look like: @Override public String toString() { StringBuilder str = new StringBuilder(); String eol = System.lineSeparator(); // end of line for this OS str.append(String.format("%40 %40 %40 %-25s", birthDate.getDay(), birthDate.getMonth(), birthDate.getYear(), breed)); str.append(eol); str.append(String.format("%7.2f%-15s%-12s", weight, name, owner)); return str.toString(); } Cut and paste from Dog to Pet until your Pet toString() matches that. Then delete the toString() method in Dog and its documentation. 6. Since Dog is the class that implements Comparable, but is now abstract it makes no sense to implement the compareTo() here. Abstract classes cannot be instantiated and therefore we don't have anything to sort, but in order to force subclasses to implement one, we will make this one abstract. Do to the compareTo() method what you did to the accessors. 7. Lastly, since Dog is now abstract and cannot be instantiated, the unit test is no longer even possible. Delete the main method and its documentation. 8. Dog should be down to about 35 lines now. Declaration of 3 protected properties, the constructor with documentation which we didn't touch, and 4 abstract methods. 5 Time to Run and Test Again Time to click the green arrow on the tool bar and See if Plato still wins! Well? Did he? 6 Polymorphism Demonstration One of the reasons that small classes and inheritance makes OOP so wonderful is reuse. We are going to work on a different project now. Download and unzip, and open Vet ClinicDriver application. The Dog and Pet are already there, I've added one class called Shelter Dog (they 3 don't have an owner name). There is usually a little more difference between subclasses, but the point of this exercise is to demonstrate polymorphism. You should remember from your reading that an object of a subclass is also an instance of its super class. Hopefully you will understand when we are finished with this part of the lab. 7 Add a Patient Class This one will be quite small. Add implements Comparable
to the class declaration. It has two properties a patient Number (String) and a patient (Dog). It needs a parameterized constructor that receives values for the properties through the parameter list. Accessors for the two parameters, a toString(), and a compareTo(). Declare the private properties, then use the Autogenerate for the constructor and the accessors. Here is the toString(): @Override public String toString() { return patient Number + } 11 11 + patient.toString(); And the compare To() will allow sorting by patient Number. You can copy the documentation and the code from one of the other classes and then change it so that it looks like this: (only the last line here is different) @Override public int compareTo (Dog that) { // some constants for clarity final int BEFORE = -1; final int EQUAL = 0; final int AFTER = 1; int comparison = EQUAL; // shouldn't be any null objects, but if there are // put them at the end if (that == null) { comparison = AFTER; //this optimization is usually worthwhile, and can //always be added - if the addresses are the same... they are equal } else if (this == that) { comparison = EQUAL; 1/we will sort by the patient's patient number (a String) } else { comparison = this.patientNumber.compareTo (that.getPatientNumber); } return comparison; } 4 As soon as you are finished, do 2 things. Choose Source =>Format to fix the formatting of your code, and then select Tools =>Analyze JavaDoc. Click the top checkbox in the list and click [Fix Selected] Normally, you would go back and complete the documentation, but for this lab, you can leave it as it is. 8 Add the Clinic Class The Clinic class is a "container" class. It will be the class that carries out the functions of the clinic with respect to patients. Our Clinic only has one private property: an ArrayList of Patient objects (ArrayList) which should be declared, but not created. A default constructor that creates the ArrayList, an addPatients(), a sort Patients(), and a toString(). An ArrayList is a Java Collection so there are some services built in, one of which is a sort method that will use the compareTo() in Patient to facilitate the sort. See them below. public class Clinic { ArrayList patients; public Clinic() { patients = new ArrayList(); } public void addPatient (Patient p) { patients.add(p); } public void sortPatients() { Collections. sort(patients); } @Override public String toString() { StringBuilder str = new StringBuilder(); String eol = System.line Separator(); // enhanced for loop works with all Java Collections for (Patient p: patients) { str.append(p.toString()).append(eol); } return str.toString(); } } As soon as you are finished, do 2 things. Choose Source =>Format to fix the formatting of your code, and then select Tools =>Analyze JavaDoc. Click the top checkbox in the list and click [Fix Selected] Normally, you would go back and complete the documentation, but for this lab, you can leave it as it is. You can select the package in the Projects frame on the left and have it Analyze the JavaDoc in all of your classes at once. (which I do when I grade) It let's you know if you have forgotten anything. 9 Complete the Driver I've left the coding of the readFile() method for you. See it below: public void readFile(String fileName) { // now try to connect the symbolic name to the physical file try { // if the physical file doesn't exist it throws an exception Scanner inFile = new Scanner(new FileReader(fileName)); if (!inFile.hasNext()){ System.err.println("The file is empty."); System.exit(1); } Patient patient; // declare, do not create while (inFile.hasNext()) { // read the common fields String patientNum = inFile.next(); int month = inFile.nextInt(); int day = inFile.nextInt(); int year = inFile.nextInt(); Date dob = new Date(month, day, year); String breed = inFile.nextLine(); // breed has imbedded spaces double weight = inFile.nextDouble(); // double is on the next line String name = inFile.next(); // ShelterDogs have special patient numbers that are greater than 70000 if (patient Num.compareTo("70000")