Question
Please help me to convert below code to acceptable pseudocode // Java implementation of Min Heap public class MinHeap { private int [] Heap; private
Please help me to convert below code to acceptable pseudocode
// Java implementation of Min Heap
public class MinHeap {
private int[] Heap;
private int size;
private int maxsize;
private static final int FRONT = 1;
public MinHeap(int maxsize) {
this.maxsize = maxsize;
this.size = 0;
Heap = new int[this.maxsize + 1];
Heap[0] = Integer.MIN_VALUE;
}
// Function to return the position of // the parent for the node currently // at pos
private int parent(int pos) {
return pos / 2;
}
// Function to return the position of the // left child for the node currently at pos
private int leftChild(int pos) {
return (2 * pos);
}
// Function to return the position of // the right child for the node currently // at pos
private int rightChild(int pos)
{
return (2 * pos) + 1;
}
// Function that returns true if the passed // node is a leaf node
private boolean isLeaf(int pos)
{
if (pos >= (size / 2) && pos
return true;
}
return false;
}
// Function to swap two nodes of the heap
private void swap(int fpos, int spos) {
int tmp;
tmp = Heap[fpos];
Heap[fpos] = Heap[spos];
Heap[spos] = tmp;
} // Function to heapify the node at pos
private void minHeapify(int pos)
{
// If the node is a non-leaf node and greater // than any of its child
if (!isLeaf(pos)) {
if (Heap[pos] > Heap[leftChild(pos)]
|| Heap[pos] > Heap[rightChild(pos)]) {
// Swap with the left child and heapify // the left child
if (Heap[leftChild(pos)]
swap(pos, leftChild(pos));
minHeapify(leftChild(pos));
}
// Swap with the right child and heapify // the right child
else {
swap(pos, rightChild(pos));
minHeapify(rightChild(pos));
}
}
}
}
// Function to insert a node into the heap
public void insert(int element) {
if (size >= maxsize) {
return;
}
Heap[++size] = element;
int current = size;
while (Heap[current]
swap(current, parent(current));
current = parent(current);
}
}
// Function to print the contents of the heap
public void print() {
for (int i = 1; i
System.out.print(" PARENT : " + Heap[i]
+ " LEFT CHILD : " + Heap[2 * i]
+ " RIGHT CHILD :" + Heap[2 * i + 1]);
System.out.println();
}
}
// Function to build the min heap using // the minHeapify
public void minHeap() {
for (int pos = (size / 2); pos >= 1; pos--) {
minHeapify(pos);
}
}
// Function to remove and return the minimum // element from the heap
public int remove() {
int popped = Heap[FRONT];
Heap[FRONT] = Heap[size--];
minHeapify(FRONT);
return popped;
}
// Driver code
public static void main(String[] arg) {
System.out.println("The Min Heap is ");
MinHeap minHeap = new MinHeap(15);
minHeap.insert(5);
minHeap.insert(3);
minHeap.insert(17);
minHeap.insert(10);
minHeap.insert(84);
minHeap.insert(19);
minHeap.insert(6);
minHeap.insert(22);
minHeap.insert(9);
minHeap.minHeap();
minHeap.print();
System.out.println("The Min val is " + minHeap.remove());
}
}
Pseudocode guidelines Pseudocode means that you don't have to write every line in Java with correct syntax. English explanations of operations are acceptable. In general, you may substitute English for any constant-time operation. The following example is not acceptable pseudocode. scan the list and count all elements less than x The following example is acceptable pseudocode. while the list has elements: increment the counter if the current element is greater than x move to the next element of the list The idea is that you don't have to give all the nitty-gritty coding details, but you should demonstrate a clear understanding of what your algorithm does and where those nitty-gritty details would have to go. To indicate blocks of code, either use braces {} or indentation levelsStep by Step Solution
There are 3 Steps involved in it
Step: 1
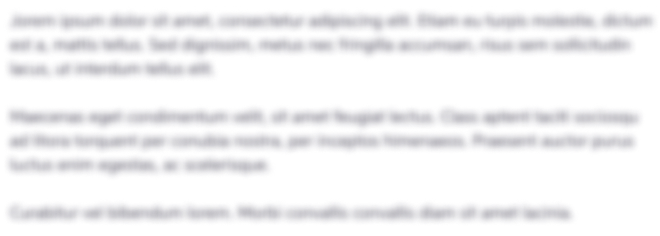
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started