Question
Please help me to do the lab hw, which is queue. Thank you so much! First, here is queue file. import java.util.NoSuchElementException; public class Queue
Please help me to do the lab hw, which is queue. Thank you so much!
First, here is queue file. import java.util.NoSuchElementException; public class Queue
return false;
} /****MUTATORS****/ /** * Inserts a new value at the end of * the Queue * @param data the new data to insert * @postcondition a new node at the end * of the Queue */ public void enqueue(T data) {} /** * Removes the front element in the Queue * @precondition !isEmpty() * @throws NoSuchElementException when * the precondition is violated * @postcondition the front element has * been removed */ public void dequeue() throws NoSuchElementException {} /****ADDITONAL OPERATIONS****/ /** * Returns the values stored in the Queue * as a String, separated by a blank space * with a new line character at the end * @return a String of Queue values */ @Override public String toString() { String result = ""; result += " "; return result; } }
Part 2: Adding Recursion to Your Queue and Stack
PrintReverse Methods:
- For *both* the Queue and Stack classes, write the below recursive methods to display the Queue and Stack in reverse.
/** * Prints in reverse order to the * console, followed by a new line * by calling the recursive helper * method printReverse */ public void printReverse() { return; } /** * Recursively prints to the console * the data in reverse order (no loops) * @param node the current node */
private void printReverse(Node node) { return; }
Sorted Order Methods (10 pts):
- For both the Queue and Stack classes, write a recursive method to determine whether or not a Queue or Stack is in sorted order (smallest value to largest value).
- First, update your class signature as follows to extend Comparable.
public class Queue
public class Stack
/** * Determines whether data is sorted * in ascending order by calling * its recursive helper method isSorted() * Note: when length == 0 * data is (trivially) sorted * @return whether the data is sorted */ public boolean isSorted() { return false; }
/** * Helper method to isSorted * Recursively determines whether data is sorted
* @param node the current node
* @return whether the data is sorted */ private boolean isSorted(Node node) { return false; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
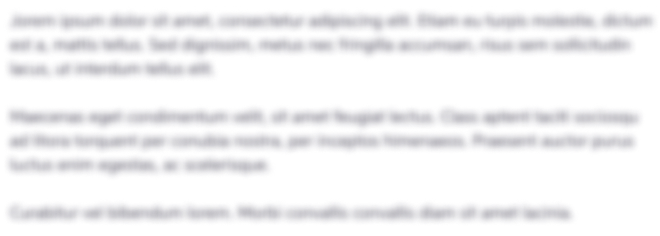
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started