Question
Please help me to finish my C Programming... Step 1: print all command-line arguments If a user enters a command-line arguments such as: God loves
Please help me to finish my C Programming...
Step 1: print all command-line arguments
If a user enters a command-line arguments such as: God loves you! For example: $> struct.exe God loves you! $> c:\users\user\Dropbox\CodeBlock\struct\struct.exe $> God $> loves $> you! $>
Step 2 ? invoke argscat() with argc and argv
1. First of all, define a variable to store a delimiter. 2. Define a function prototype to access the argscat() in main.c. - char *argscat(int argc, char *argv[], char *delimiter); 3. Then create a file called argscat.c to implement the argscat() function. - Use assert() after malloc() to check the return status of malloc() call. 4. Invoke argscat() with argc and argv in main.c. 5. Use assert() function. 6. Debug the code if necessary. You may run argscat.c provided.
Step 3 invoke argscat() such that it excludes the first argument
If argscat() is properly implemented, you dont need to change it in this step. You just modify how to invoke the function itself.
Step 4 use a pointer to print the args string in uppercase
- Use the pointer to string you got in step 3 here. - Print it in uppercase excluding non-alpha using a while loop. - Use toupper() to convert and isalpha() built-in functions. - Don't make a copy of the string. - Dont convert the string itself to an uppercase string, but use a pointer and pointer arithmetic to access each character.
Step 5 use an array indexing to print the args string in lowercase
- Use the pointer to string you got in step 3 here. - Print it in lowercase excluding non-alpha using a for loop. - Use tolower() to convert and isalpha() built-in functions. - Don't make a copy of the string. - Dont convert the string itself to a lowercase string, but use an array indexing to access each character.
Step 6 modify main() in main.c to free resources allocated in argscat()
Add some code in main() to free the memory allocated in argscat(). Since there were two calls of malloc(), two calls of free() are expected to free all resources allocated. Remember that the number of malloc() calls should match with that of free() calls.
(this is a skeleton file)
/** * Week3: Homework 1 - Implement argscat() function * * argscat() accepts an array of pointers to strings and * returns a concatenated string separated by a delimiter * passed by the user. */
#include
int main(int argc, char *argv[]) { printf("Happy Coding! ");
// step 1: print all command-line arguments for (int i = 0; i < argc; i++) printf("%s ", argv[i]);
// step 2: invoke argscat() with argc and argv.
// step 3: invoke argscat() such that it excludes the first argument.
// step 4: Use the pointer to string you got in step 3 here. // print it in uppercase excluding non-alpha using a while loop. // don't copy the string, but use a pointer and pointer arithmetic
// step 5: Use the pointer to string you got in step 3 here. // print it in lowercase excluding non-alpha using a for loop. // don't copy the string, but use a pointer and pointer arithmetic
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
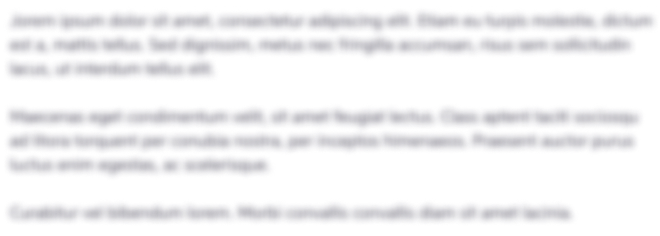
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started