Question
Please help me with only the comparison operators: The comparison operators must compare the decimal values. How can I use the toDecimal() function with the
Please help me with only the comparison operators:
The comparison operators must compare the decimal values.
How can I use the toDecimal() function with the comparison operators?
Here is my program:
#include
#include
#include
using namespace std; // For C standard library
// Start of class Fraction
class Fraction {
private:
// Declare the private variables
int numerator;
int denominator;
public:
// Default Constructor
Fraction() {
// Setting the default fraction of numerator of 1
// and denominator of 1
numerator = 0;
denominator = 1;
}
// Overloaded constructor
Fraction(int numTop, int denBottom) {
numerator = numTop;
denominator = denBottom;
}
// Setter function for numerator
void setNumerator(int n) {
numerator = n;
}
// Getter function for numerator
int getNumerator() const {
return numerator;
}
// Setter function for denominator
void setDenominator(int d) {
denominator = d;
}
// Getter function for denominator
int getDenominator() const {
return denominator;
}
// Fraction to decimal converter "toDecimal"
double toDecimal() {
return static_cast
}
// Convert the fraction to a string using the append and
// to string methods
string toSring() {
string decimalStr = "";
decimalStr.append(to_string((int)numerator));
decimalStr.append("/");
decimalStr.append(to_string((int)denominator));
return decimalStr;
}
// Reduce fraction function using gcd function
int reduce() {
int numer = numerator;
int denom = denominator;
int gcd;
while (numer != denom) {
if (denom > numer)
denom -= numerator;
else
numer -= denom;
}
numerator = numerator / numer;
denominator = denominator / numer;
return numer;
}
// Assign numerator and denominator of fraction two to
// numerator and denominator of fraction one
Fraction &operator=(const Fraction &f2) {
numerator = f2.numerator;
denominator = f2.denominator;
return *this;
}// Operator=
// Increment the numerator operator++
Fraction &operator++() {
++numerator;
return *this;
}// Operator++
// Decrement the numerator operator--
Fraction &operator--() {
--numerator;
return *this;
}// Operator++
};// End of class Fraction
Fraction f1, f2;
// Overloaded operators
// Is fraction one equal to fraction two?
bool operator==(const Fraction &f1, const Fraction &f2) {
if (f1 == f2)
return true;
else
return false;
}// Operator ==
// Is fraction one less than fraction two?
bool operator<(const Fraction &f1, const Fraction &f2) {
return (f1 < f2);
}// Operator <
// Is fraction one less than or equal to fraction two?
bool operator<=(const Fraction &f1, const Fraction &f2) {
return (f1 <= f2);
}// Operator <=
// Is fraction one greater than fraction two?
bool operator>(const Fraction &f1, const Fraction &f2) {
return (f1 > f2);
}// Operator >
// Is fraction one greater than or equal to fraction two?
bool operator>=(const Fraction &f1, const Fraction &f2) {
return (f1 >= f2);
}// Operator >=
// Start of main
int main()
{
Fraction frac; // Declare a Fraction object
// Declare and initialize variables
int numerator = 0; // User input numerator variable
int denominator = 0; // User input denominator variable
// Display the fractions default values that has a
// numerator of 0 and a denominator of 1
cout << "The numerator is: " << frac.getNumerator() << endl;
cout << "The denominator is: " << frac.getDenominator() << endl;
cout << "Setting and displaying a numerator of 2." << endl;
frac.setNumerator(2);
cout << "The numerator is now: " << frac.getNumerator() << endl;
cout << "Setting and displaying a denominator of 3." << endl;
frac.setDenominator(3);
cout << "The denominator is now: " << frac.getDenominator() << endl;
// "Prompt the user for a numerator
cout << "Please enter a numerator: " << endl;
cin >> numerator;
// Set the numerator
frac.setNumerator(numerator);
// "Prompt the user for a denominator and
// "check for division by 0
cout << "Please enter a denominator: " << endl;
cin >> denominator;
// Check for denominator = 0
while (denominator == 0) {
cout << "Please enter a denominator other than 0" << endl;
cin >> denominator;
}
// Set the denominator to valid arguement
frac.setDenominator(denominator);
cout << "The denominator was set to " << "\'" << frac.getDenominator()
<< "\'" << " which is greater than 0." << endl;
// Declaring second fraction object frac1
Fraction frac1;
// Converting default numerator and denominator values to decimal
cout << "Converting default numerator and denominator values to decimal."
<< endl;
cout << "The numerator is: " << frac1.getNumerator() << endl;
cout << "The denominator is: " << frac1.getDenominator() << endl;
cout << "Converting the numerator and denominator to decimal." << endl;
// Convert default fraction to decimal and store in declared variable
double decimal = frac1.toDecimal();
// Display the converted fraction to decimal value
cout << "The converted fration to it's equivalent decimal value is: "
<< decimal << endl;
// Convert the numerator and denominator of frac to string
string deciString = frac.toSring();
// Display the converted fraction to string
cout << "The fraction frac converted to string is: " << deciString
<< endl;
// Setting different values for numerator and denominator for frac1
cout << "Set the numerator of object frac1 to 4 and "
<< " set the denominator to 12." << endl;
frac1.setNumerator(4); frac1.setDenominator(12);
cout << "The numerator and denominator of object frac1 are "
<< frac1.getNumerator() << ", " << frac1.getDenominator()
<< " respectively." << endl;
// Call the reduce fraction method to reduce the fraction of frac1
int gcd = frac1.reduce();
cout << "The greatest common denominator of frac1 is: " << gcd << endl;
cout << "And the equivalent reduced fraction of frac1 is: "
<< frac1.getNumerator() << "/" << frac1.getDenominator() << endl;
// Set the Fraction object "frac" to 1/2 to compare to "frac1"
cout << "Setting Fraction object \"frac\" to 1/2." << endl;
frac.setNumerator(1); frac.setDenominator(2);
// Use the overloaded comparison operator "==" to compare
// frac and frac1
if (frac == frac1) {
cout << "The two fractions frac and frac1 are equal." << endl;
}
else {
cout << "The two fractions frac and frac1 are not equal." << endl;
}
// Use the overloaded operators "<" to compare frac and frac1
if (frac.toDecimal() < frac1.toDecimal()) {
cout << "Fraction 1 is less than fracton 2." << endl;
}
else{
cout << "Fraction 1 is not less than fraction 2." << endl;
}
// Use the overloaded operators "<=" to compare frac and frac1
if (frac.toDecimal() <= frac1.toDecimal()) {
cout << "Fraction 1 is less than or equal to fracton 2." << endl;
}
else {
cout << "Fraction 1 is not less than or equal to fraction 2." << endl;
}
// Use the overloaded operators ">" to compare frac and frac1
if (frac.toDecimal() > frac1.toDecimal()) {
cout << "Fraction 1 is greater than fracton 2." << endl;
}
else {
cout << "Fraction 1 is not greater than fraction 2." << endl;
}
// Use the overloaded operators ">=" to compare frac and frac1
if (frac.toDecimal() >= frac1.toDecimal()) {
cout << "Fraction 1 is greater than or equal to fracton 2." << endl;
}
else {
cout << "Fraction 1 is not greater than or equal to fraction 2." << endl;
}
// Display fract and frac1's contents
cout << "The numerator and denominator of frac are: "
<< frac.getNumerator() << " and " << frac.getDenominator()
<< " respectively." << endl;
cout << "The numerator and denominator of frac1 are: "
<< frac1.getNumerator() << " and " << frac1.getDenominator()
<< " respectively." << endl;
// Assign the numerator and denominator of frac1 to frac
cout << "Assigning frac1 numerator and denominator to frac" << endl;
frac = frac1;
// Display frac assigned contents
cout << "The numerator and denomenator of frac are now: "
<< frac.getNumerator() << " and " << frac.getDenominator()
<< " respectively." << endl;
// Increment the numerator of frac
cout << "The value of frac is: " << frac.getNumerator()
<< "/" << frac.getDenominator() << ".";
// Increment frac
++frac;
cout << " Incrementing frac by 1 is: " << frac.getNumerator()
<< "/" << frac.getDenominator() << endl;
// Decrement the numerator of frac
cout << "The value of frac is: " << frac.getNumerator()
<< "/" << frac.getDenominator() << ".";
// Decrement frac
--frac;
cout << " Decrementing frac by 1 is: " << frac.getNumerator()
<< "/" << frac.getDenominator() << " ";
system("pause"); // Pause the program
return 0; // Return 0 if all went well
}// End of main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
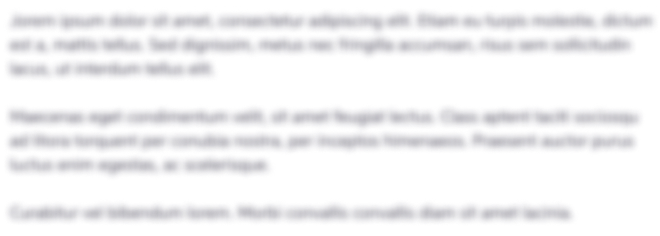
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started