Question
Please help me with this entire C++ lab example. I know it looks long but i promise most of it are just explanations. I will
Please help me with this entire C++ lab example. I know it looks long but i promise most of it are just explanations. I will provide the given codes below the pictured instructions. thank you so much
-------------------------------------------------------------------------------------------------------------------------
// iadrv.h
#ifndef _IADRV_H
#define _IADRV_H
#include "intarray.h"
int main();
void test1();
void test2();
void test3();
void test4();
void test5();
void test6();
void test7();
void test8();
void test9();
void test10();
void test11();
void test12();
void test13();
void test14();
void test15();
void test16();
void test17();
void test18();
void test19();
void test20();
void wait();
#endif
-------------------------------------------------------------------------------------------------------------------------
// iadrv.cpp - driver program for testing IntArray class
#include
#include
#include
#include
#include "iadrv.h"
using namespace std;
ofstream csis;
int main() {
csis.open("csis.txt");
test1();
test2();
test3();
test4();
test5();
test6();
test7();
test8();
test9();
test10();
test11();
test12();
test13();
test14();
test15();
test16();
test17();
test18();
test19();
test20();
csis.close();
}
void test1() {
cout
csis
IntArray a(10);
for(int i = a.low(); i
a[i] = i * 10;
a.setName("a");
cout
csis
wait();
}
void test2() {
cout
csis
IntArray b(-3, 6);
for(int i = b.low(); i
b[i] = i * 10;
b.setName("b");
cout
csis
wait();
}
void test3() {
cout
csis
IntArray c(6, 8);
for(int i = c.low(); i
c[i] = i * 10;
c.setName("c");
cout
csis
wait();
}
void test4() {
cout
csis
IntArray d(5, 5);
for(int i = d.low(); i
d[i] = i * 10;
d.setName("d");
cout
csis
wait();
}
void test5() {
cout
csis
IntArray z;
for(int i = z.low(); i
z[i] = i * 10;
z.setName("z");
cout
csis
wait();
}
void test6() {
cout
cout
csis
csis
IntArray c(6, 8);
for(int i = c.low(); i
c[i] = i * 10;
c.setName("c");
cout
csis
IntArray e(c);
e.setName("e");
cout
csis
wait();
}
void test7() {
cout
cout
cout
csis
csis
csis
IntArray f(1, 4);
for(int i = f.low(); i
f[i] = i * 10;
f.setName("f");
cout
csis
IntArray g(5, 8);
for(int i = g.low(); i
g[i] = i * 10;
g.setName("g");
cout
csis
wait();
f = g;
cout
cout
csis
csis
wait();
}
void test8() {
cout
cout
cout
cout
csis
csis
csis
csis
IntArray j(3, 6);
for(int i = j.low(); i
j[i] = i * 10;
j.setName("j");
cout
csis
IntArray k(6, 9);
for(int i = k.low(); i
k[i] = i * 10;
k.setName("k");
cout
csis
IntArray l(1, 4);
for(int i = l.low(); i
l[i] = i * 10;
l.setName("l");
cout
csis
wait();
j = k = l;
cout
cout
cout
csis
csis
csis
wait();
}
void test9() {
cout
cout
cout
csis
csis
csis
IntArray m(3, 7);
for(int i = m.low(); i
m[i] = i * 10;
m.setName("m");
cout
csis
IntArray n(1, 5);
for(int i = n.low(); i
n[i] = i * 10;
n.setName("n");
cout
csis
wait();
m = n;
cout
cout
cout
csis
csis
csis
wait();
}
void test10() {
cout
cout
cout
csis
csis
csis
IntArray o(3, 7);
for(int i = o.low(); i
o[i] = i * 10;
o.setName("o");
cout
csis
IntArray p(1, 5);
for(int i = p.low(); i
p[i] = i * 10;
p.setName("p");
cout
cout
csis
csis
wait();
}
void test11() {
cout
cout
cout
csis
csis
csis
IntArray q(1, 3);
for(int i = q.low(); i
q[i] = i * 10;
q.setName("q");
cout
csis
IntArray r(1, 4);
for(int i = r.low(); i
r[i] = i * 10;
r.setName("r");
cout
cout
csis
csis
wait();
}
void test12() {
cout
cout
cout
csis
csis
csis
IntArray s(3, 7);
for(int i = s.low(); i
s[i] = i * 10;
s.setName("s");
cout
csis
IntArray t(1, 5);
for(int i = t.low(); i
t[i] = i * 10;
t.setName("t");
cout
csis
wait();
s = t;
cout
cout
cout
csis
csis
csis
wait();
}
void test13() {
cout
cout
cout
csis
csis
csis
IntArray u(3, 7);
for(int i = u.low(); i
u[i] = i * 10;
u.setName("u");
cout
csis
IntArray v(1, 5);
for(int i = v.low(); i
v[i] = i * 10;
v.setName("v");
cout
cout
csis
csis
wait();
}
void test14() {
cout
cout
cout
csis
csis
csis
IntArray w(1, 3);
for(int i = w.low(); i
w[i] = i * 10;
w.setName("w");
cout
csis
IntArray x(1, 4);
for(int i = x.low(); i
x[i] = i * 10;
x.setName("x");
cout
cout
csis
csis
wait();
}
void test15() {
cout
cout
cout
csis
csis
csis
IntArray a(1, 5);
for(int i = a.low(); i
a[i] = i * 10;
a.setName("a");
cout
csis
IntArray b(4, 8);
for(int i = b.low(); i
b[i] = i * 10;
b.setName("b");
cout
csis
wait();
IntArray c = a + b;
c.setName("c");
cout
csis
wait();
}
void test16() {
cout
cout
cout
csis
csis
csis
IntArray d(10, 13);
for(int i = d.low(); i
d[i] = i * 10;
d.setName("d");
cout
csis
IntArray e(30, 33);
for(int i = e.low(); i
e[i] = i * 10;
e.setName("e");
cout
csis
d += e;
cout
csis
wait();
}
void test17() {
cout
csis
IntArray f(5, 2);
for(int i = f.low(); i
f[i] = i * 10;
f.setName("f");
cout
csis
wait();
}
void test18() {
cout
cout
csis
csis
IntArray g(10);
for(int i = g.low(); i
g[i] = i * 10;
g.setName("g");
cout
csis
g[10] = 1;
wait();
}
void test19() {
cout
cout
cout
csis
csis
csis
IntArray m(1, 4);
for(int i = m.low(); i
m[i] = i * 10;
m.setName("m");
cout
csis
IntArray n(2, 4);
for(int i = n.low(); i
n[i] = i * 10;
n.setName("n");
cout
csis
wait();
m = n;
cout
cout
csis
csis
wait();
}
void test20() {
cout
cout
cout
csis
csis
csis
IntArray o(7, 8);
for(int i = o.low(); i
o[i] = i * 10;
o.setName("o");
cout
csis
o[7] = 25;
o[8] = o[7];
cout
csis
wait();
}
void wait() {
char buf;
cout
cin.get(buf);
}
Operator Overloading Lab (IntArray) The array construct in C is very efficient but also very dangerous for the unwary For example, many novice programmers fall into the trap of declaring an array of 100 elements and then try to access the element with index 100. Not only is thie an error in C, but the language won't even alert the user when the mistake is made. C++ allows programmers to define safer and more flexible array constructs if they are willing to sacrifice some of C's runtime efficiency. The purpose of this lab is to see how this is done and to gain some experience in overloading operators called IntArray rray With it, the user will be able to declare integer arrays of any size with automatic range checking of indices. The upper and lower indices can be any integer positive or negative, rather than the fixed limits of o to SIZE-1. It will also be possible to assign entire arrays to each other, compare two arrays for equal and inequality, add two arrays, and output arrays using the overloaded ity operator. For example: tinclude ciostream> include "IntArray.h" using namespace std; int main) f // Ten elements, indexed 0 to 9 // Ten elements, indexed -3 to 6 // Three elements, indexed 6 to 8 // Single element array, indexed at 5 // Ten elements, indexed 0 to 9 IntArray a (10), w(10) IntArray b(-3, 6) IntArray c(6, 8) IntArray d(5, 5); IntArray zi // high() and low() return largest and smallest legal indices for (int i =a.Iow(); i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
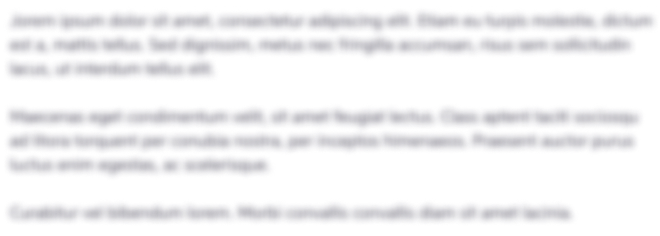
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started