Question
Please help me with this homework assignment I'm struggling GIVEN CODE: from hw5_util import * # a function to print the grid def print_grid(grid): for
Please help me with this homework assignment I'm struggling
GIVEN CODE:
from hw5_util import *
# a function to print the grid
def print_grid(grid):
for row in grid:
for item in row:
print(item, end=' ')
print()
# a function to get neighbours of point marked by (row, col)
def get_neighbors(grid, rows, cols, row, col):
neighbors = []
if row - 1 >= 0:
neighbors.append((row-1, col))
if col -1 >= 0:
neighbors.append((row, col-1))
if row + 1
neighbors.append((row+1, col))
if col + 1
neighbors.append((row, col + 1))
return neighbors
# a function returns true if two points are adjacent
def is_adjacent(point_a, point_b):
if abs(point_a[0] - point_b[0]) == 1 and point_a[1] == point_b[1]:
return True
if abs(point_a[1] - point_b[1]) == 1 and point_a[0] == point_b[0]:
return True
return False
# a function which returns true if a point is valid point
def is_valid_point(point, rows, cols):
if 0
return True
return False
# a function which determines if the path is valid or not
def is_valid_path(path, rows, cols, index):
if not(is_valid_point(path[index], rows, cols)):
return False
if index == len(path) - 1:
return True
if is_adjacent(path[index], path[index + 1]):
return is_valid_path(path, rows, cols, index + 1)
else:
return False
# a function to calculate the total up or down elevation
def calculate_up_down(grid, path):
up = 0
down = 0
curr_loc = path[0]
for index in range(1, len(path)):
loc = path[index]
elevation = grid[loc[0]][loc[1]] - grid[curr_loc[0]][curr_loc[1]]
if elevation > 0:
up += elevation
elif elevation
down += abs(elevation)
curr_loc = path[index]
return up, down
# the main method
def main():
# read grid
read_grids()
grid_no = 0
while True:
try:
grid_no = int(input('Enter a grid index less than or equal to 3 (0 to end): '))
if grid_no == 0:
print('Exitting . . .')
exit(0)
if grid_no 3:
print('Please enter number from 1 to 3 only')
else:
break
except:
print('Invalid number!')
print()
choice = input('Should the grid be printed (Y or N): ')
grid = get_grid(grid_no)
if choice.lower() == 'y':
print('Grid', grid_no)
print_grid(grid)
rows = len(grid)
cols = len(grid[0])
print('Grid has {0} rows and {1} columns'.format(rows, cols))
start_locations = get_start_locations(grid_no)
for loacation in start_locations:
print('Neighbors of {0}: {1}'.format(loacation, get_neighbors(grid, rows, cols, loacation[0], loacation[1])))
path = get_path(grid_no)
if is_valid_path(path, rows, cols, 0):
print('Valid path')
up, down = calculate_up_down(grid, path)
print('Downward', down)
print('Upward', up)
else:
for index in range(1, len(path)):
if (not is_adjacent(path[index], path[index + 1])) or (not is_valid_point(path[index], rows, cols)) or (not is_valid_point(path[index], rows, cols)):
print('Path invalid step from {0} to {1}'.format(path[index], path[index + 1]))
break
main()
HERE'S THE ACTUAL PROBLEM:
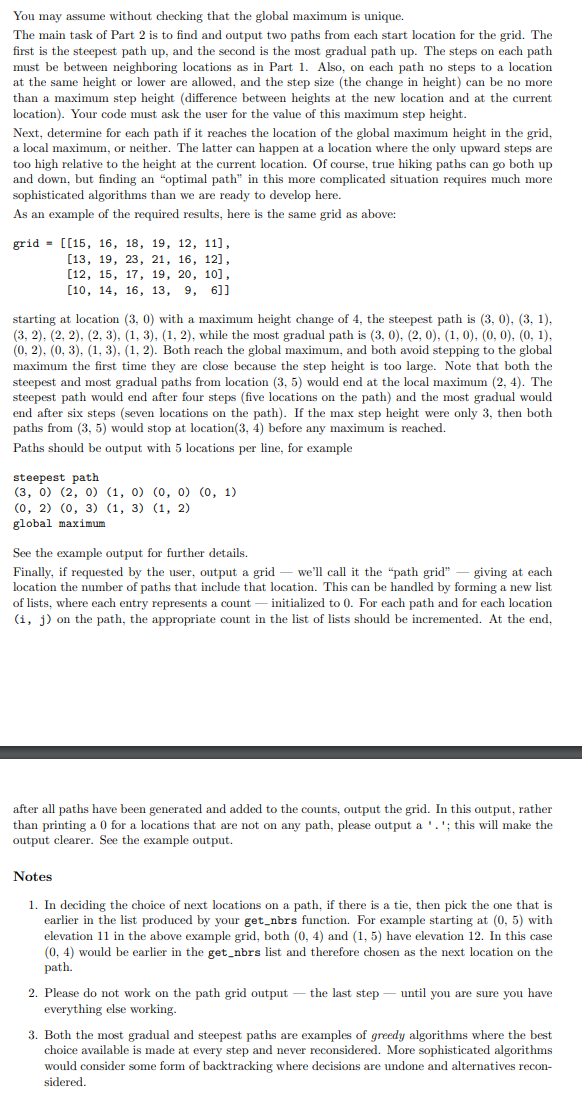
Step by Step Solution
There are 3 Steps involved in it
Step: 1
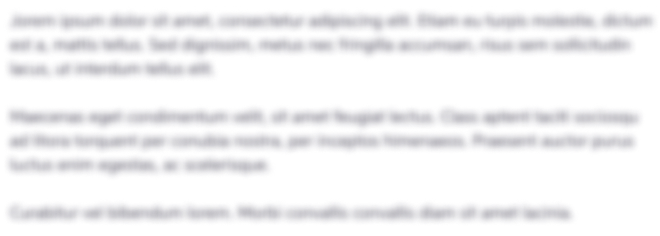
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started