Question
Please help me with this question. the question asks for implementation of the file stats.h which is already given and I am attaching it with
Please help me with this question. the question asks for implementation of the file stats.h which is already given and I am attaching it with the question. The implementation file called stats.cpp is required. In the implementation file we should use private member function, example the const length() function returns private member function count. all the implementation instruction is written in the beginning of stats.h. Couple of times which I requested help, I received a copy paste of a program and codes which are already available in Chegg and the are not relevant to this question. Thanks in advance.
PRECODE FILE (stats.h) COPY PASTE CODE.
#ifndef STATS_H // Prevent duplicate definition
#define STATS_H
#include
namespace CISP430_A1
{
class statistician
{
public:
// CONSTRUCTOR
statistician( );
// MODIFICATION MEMBER FUNCTIONS
void next(double r);
void reset( );
// CONSTANT MEMBER FUNCTIONS
int length( ) const { return count; }
double sum( ) const { return total; }
double mean( ) const;
double minimum( ) const;
double maximum( ) const;
// FRIEND FUNCTIONS
friend statistician operator +
(const statistician& s1, const statistician& s2);
friend statistician operator *
(double scale, const statistician& s);
private:
int count; // How many numbers in the sequence
double total; // The sum of all the numbers in the sequence
double tiniest; // The smallest number in the sequence
double largest; // The largest number in the sequence
};
// NON-MEMBER functions for the statistician class
bool operator ==(const statistician& s1, const statistician& s2);
}
You will create, implement and test a small class called statistician, which is similar to some of the small classes in Chapter 2 of the text. The new class is called statistician, using a header file, stats.h (attached).(most of which is written for you) and an implementation file, stats.cpp (which you need to create and implement.). The statistician is a class that is designed to keep track of simple statistics about a sequence of real numbers. There are two member functions that you should understand at an informal level before you proceed any further. The prototypes for these two functions are shown here as part of the statistician class declaration: class statistician { public: void next (double r); double mean const; }; The member function "next" is used to give a sequence of numbers to the statistician one at a time. The member function "mean" is a constant member function that returns the ' arithmetic mean (i.e., the average) of all the numbers that have been given to the statistician. Example: Suppose that you want a statistician to compute the mean of the sequence 1.1, 2.8,-0.9. Then you could write these statements: // Declares a statistician object called s statistician si // Give the three numbers 1.1, 2.8 and -0.9 to the statistician S.next(1.1); S.next.(2.8); s.next(-0.9); // Call the mean function, and print the result followed by a carriage return cout 0. You cannot use these three member functions unless the statistician has been given at least one number!) A constant member function called sum, which returns the sum of all the numbers that have been given to the statistician. This function does NOT have a precondition. It may be called even if the statistician has NO numbers (in which case it should return 0). An overloaded operator == which tests to see whether two statisticians are "equal". The prototype is: bool operator ==(const statistician& s, const statistician& t); In order for two statisticians to be equal, they must have the same length (i.e., they have been given the same number of numbers). Also, if their length is greater than zero, they must also have the same mean, the same minimum, the same maximum, and the same sum. For example: Suppose that a statistician s has been given four numbers 1, 2, 3, 4. A second statistician t has been given four numbers 1, 1.5, 3.5, 4. Then the test (s==t) must return true since both s and t have equal values for all the member functions, as shown here: s.length() and t.length() are both 4 s.mean() and t.mean(are both 2.5 s.sum() and t.sum() are both 10.0 s.minimum and t.minimum are both 1 s.maximum and t.maximum are both 4 An overloaded + operator which has two statisticians as arguments, and returns a third statistician, as shown in this prototype: statistician operator +(const statisticiana s, const statistician& t); An overloaded * operator which allows you to "multiply" a double number times a statistician. Here is the prototype: 1. 2. 3. 4. 5. . statistician operator +(const statistician& s, const statistician& t); An overloaded * operator which allows you to "multiply" a double number times a statistician. Here is the prototype: statistician operator *(double scale, const statisticiana si This is not a member function. The result of a multiplication such as 2*s is a new statistician that looks as if it had been given all the numbers of s, multiplied by the constant 2. Examples: Suppose that s is a statistician that has been given 1, 2, 3, and u is another statistician. Then the assignment statement u=2*s will result in u behaving as if it had been given the numbers 2, 4, 6. As another example, the assignment statement u=- 3*s will result in u behaving as if it had been given the numbers -3, -6, -9. Notice that neither + nor == are member functions. (See Section 2.5 in the class notes). The result of stt is a new statistician that looks as if it had been given all the numbers of the sequence for s, followed by all the numbers of the sequence for t. For example: Suppose that we have three statisticians s, t, and u. The statisticians has been given the numbers 1, 2, 3; the statistician t has been given the numbers 4, 5. Then the assignment statement u=s+t will result in u behaving as if it had been given the five numbers 1, 2, 3, 4, 5. n stats.h) No Selection 1 // FILE: stats.h 2 // CLASS PROVIDED: statistician 3 // (a class to keep track of statistics on a sequence of real numbers) 4 // This class is part of the namespace CISP430_A1. 5 // 6 // CONSTRUCTOR for the statistician class: 7 // statistician(); 8 // Postcondition: The object has been initialized, and is ready to accept 9 // a sequence of numbers. Various statistics will be calculated about the 10 // sequence. 11 // 12 // PUBLIC MODIFICATION member functions for the statistician class: 13 // void next(double r) 14 // The number r has been given to the statistician as the next number in 15 // its sequence of numbers. 16 // void reset(); 17 // Postcondition: The statistician has been cleared, as if no numbers had 18 // yet been given to it. 19 // 20 // PUBLIC CONSTANT member functions for the statistician class: 21 // int length( ) const 22 // Postcondition: The return value is the length of the sequence that has 23 // been given to the statistician (i.e., the number of times that the 24 // next(r) function has been activated). 25 // double sum() const 26 // Postcondition: The return value is the sum of all the numbers in the 27 // statistician's sequence. 28 // double mean() const 29 // Precondition: length() > 0 30 // Postcondition: The return value is the arithmetic mean (i.e., the 31 // average of all the numbers in the statistician's sequence). 32 // double minimum() const 33 // Precondition: length() > 0 34 // Postcondition: The return value is the tiniest number in the 35 // statistician's sequence. 36 // double maximum() const 37 // Precondition: length() > 0 38 // Postcondition: The return value is the largest number in the 39 // statistician's sequence. 40 // 41 // NON-MEMBER functions for the statistician class: 42 // statistician operator +(const statistician& si, const statistician& s2) 43 // Postcondition: The statistician that is returned contains all the 44 // numbers of the sequences of si and s2. 45 // statistician operator *(double scale, const statistician& s) 46 // Postcondition: The statistician that is returned contains the same 47 // numbers that s does, but each number has been multiplied by the 48 // scale number. 49 // bool operator ==(const statistician& si, const statistician& s2) 49 // bool operator ==(const statistician& si, const statistician& s2) 50 // Postcondition: The return value is true if s1 and s2 have the zero 51 // length. Also, if the length is greater than zero, then s1 and s2 must 52 // have the same length, the same mean, the same minimum, 53 // the same maximum, and the same sum. 54 // 55 // VALUE SEMANTICS for the statistician class: 56 // Assignments and the copy constructor may be used with statistician objects. 57 n stats.h) No Selection 66 68 69 73 74 76 58 #ifndef STATS_H // Prevent duplicate definition 59 #define STATS_H 60 #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
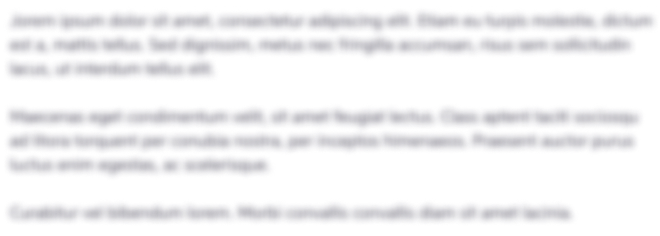
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started