Question
Please help me write these methods in java. * Given an array of doubles, return the product of all * the numbers in the array.
Please help me write these methods in java.
* Given an array of doubles, return the product of all
* the numbers in the array.
* (if the array has length 0, a value of 1 will be returned)
*
* @param array of doubles
* @return product of all array values
*/
public static double product(double[] array) {}
* Takes two arrays and multiplies pairs of doubles from
* each array together to create a new array containing
* the products. Specifically, the i-th index of the
* result array contains a1[i]*a2[i]. The result array
* length equals that of the smaller input array. Extra
* elements in the larger input array (if sizes differ)
* are ignored.
*
* @param a1 array of doubles
* @param a2 array of doubles
* @return array of products of doubles from two input arrays
*/
public static double[] zipMultiply(double[] a1, double[] a2) {
// result has length of shorter input array
double[] result = new double[(int) Math.min(a1.length, a2.length)];
// TODO
for(int i = 0 ; i < result.length; i++)
return result;
}
* If a given character x is in a given string, then
* return the substring of characters after
* the first occurrence of x. Otherwise return the
* empty string (which is just "").
* This can be accomplished without any loops at
* all if you use methods of the String class.
* Check the Java API for String.
*
* @param x character to search for
* @param s string to get a substring from (not null)
* @return substring after first x, if it exists, else ""
*/
public static String subStringAfter(char x, String s) {
return null; // TODO
}
* Indicate if two ArrayLists of Integers start with the same
* sequence of elements. Specifically, the length of the shorter
* list is used, and only those elements in the two lists are
* compared. In other words, check if the beginning portion of
* one list matches the entirety of another list. If either list
* is empty, then a result of true is returned. Note that you cannot
* know that you should return a result of true until you have looped
* as many iterations as the length of the shorter list. Also be aware
* that non-primitive Integers should be compared using the .equals method.
*
* IMPORTANT: Do not modify the contents of either parameter list.
*
* @param list1 First ArrayList of Integers
* @param list2 Second ArrayList of Integers
* @return Whether elements in shorter list match the
* first elements of the longer list in the same order.
*/
public static boolean sameStart(ArrayList
int shorterLength = Math.min(list1.size(), list2.size());
return false; // TODO
}
* Given a (potentially jagged) 2D array, treat each sub-array
* as a collection of numbers, and determine the average across
* those numbers. The final result is an array with one element for
* each sub-array in the input, which equals the average of the
* values in that sub-array. Simply put, given an array of arrays,
* return an array of averages.
*
* Note: arrayOfArrays[i][j] represents the j-th element of the i-th array.
* If you want to know the length of the i-th array, use arrayOfArrays[i].length.
*
* @param arrayOfArrays array of arrays of numbers, where each sub-array has a length of at least 1
* @return array of averages of the sub-arrays of the input
*/
public static double[] averages(double[][] arrayOfArrays) {
double[] result = new double[arrayOfArrays.length]; // arrayOfArrays.length is the number of sub-arrays
// TODO (Hint: loop through all of the arrays with an outer loop, but have an inner loop through each sub-array that adds up values)
return result;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
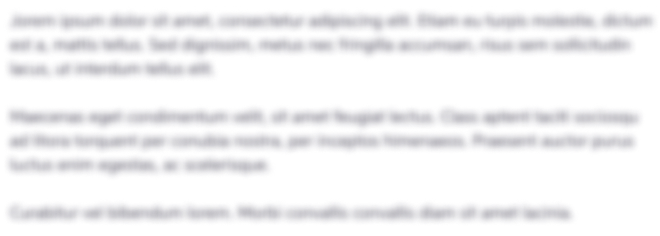
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started