Question
Please help, modify the given code blocks (both HTML and JS) below to add in the following: Modify provided template from chapter 5 example (photo
Please help, modify the given code blocks (both HTML and JS) below to add in the following:
Modify provided template from chapter 5 example (photo viewer) to include the following features:
When the user clicks on a picture and it opens a window containing a zoomed version of the image, give her a hyperlink (button) to add the pic to a favorites area at the bottom of the first page (add img nodes to the DOM).
A maximum of 5 pics can be added to the favorites. After that a message is displayed to remove at least one favorite first.
If the user clicks on a favorite, a Remove link (button) shows up next to that picture. If the user clicks it, the pic is removed from the favorites.
Use good web design practices to enhance visually your html page. Add your name and student number, colors, copyright line, etc.
HTML CODE
Interactive Slideshow
To view your slides:
- Click the Right or Left arrow buttons to move forward or backward through the image list.
- Click the Play/Pause button to automatically move forward through the image list.
- Click an image to view it in full screen mode.
JAVA CODE
"use strict";
/* JavaScript 7th Edition
Chapter 5 - A2 COMP125 Section 402,403
Chapter Case
Application to generate a slide show
Author:
Date:
Filename: js05.js
*/
window.addEventListener("load", createLightbox);
function createLightbox() {
// Lightbox Container
let lightBox = document.getElementById("lightbox");
// Parts of the lightbox
let lbTitle = document.createElement("h1");
let lbCounter = document.createElement("div");
let lbPrev = document.createElement("div");
let lbNext = document.createElement("div");
let lbPlay = document.createElement("div");
let lbImages = document.createElement("div");
// Design the lightbox title
lightBox.appendChild(lbTitle);
lbTitle.id = "lbTitle";
lbTitle.textContent = lightboxTitle;
// Design the lightbox slide counter
lightBox.appendChild(lbCounter);
lbCounter.id = "lbCounter";
let currentImg = 1;
lbCounter.textContent = currentImg + " / " + imgCount;
// Design the lightbox previous slide button
lightBox.appendChild(lbPrev);
lbPrev.id = "lbPrev";
lbPrev.innerHTML = "◀";
lbPrev.onclick = showPrev;
// Design the lightbox next slide button
lightBox.appendChild(lbNext);
lbNext.id = "lbNext";
lbNext.innerHTML = "▶";
lbNext.onclick = showNext;
// Design the lightbox Play-Pause button
lightBox.appendChild(lbPlay);
lbPlay.id = "lbPlay";
lbPlay.innerHTML = "⏯";
let timeID;
lbPlay.onclick = function() {
if (timeID) {
// Stop the slideshow
window.clearInterval(timeID);
timeID = undefined;
} else {
// Start the slideshow
showNext();
timeID = window.setInterval(showNext, 1500);
}
}
// Design the lightbox images container
lightBox.appendChild(lbImages);
lbImages.id = "lbImages";
// Add images from the imgFiles array to the container
for (let i = 0; i < imgCount; i++) {
let image = document.createElement("img");
image.src = imgFiles[i];
image.alt = imgCaptions[i];
image.onclick = createOverlay;
lbImages.appendChild(image);
}
// Function to move forward through the image list
function showNext() {
lbImages.appendChild(lbImages.firstElementChild);
(currentImg < imgCount) ? currentImg++ : currentImg = 1;
lbCounter.textContent = currentImg + " / " + imgCount;
}
// Function to move backward through the image list
function showPrev() {
lbImages.insertBefore(lbImages.lastElementChild, lbImages.firstElementChild);
(currentImg > 1) ? currentImg-- : currentImg = imgCount;
lbCounter.textContent = currentImg + " / " + imgCount;
}
function createOverlay() {
let overlay = document.createElement("div");
overlay.id = "lbOverlay";
// Add the figure box to the overlay
let figureBox = document.createElement("figure");
overlay.appendChild(figureBox);
// Add the image to the figure box
let overlayImage = this.cloneNode("true");
figureBox.appendChild(overlayImage);
// Add the caption to the figure box
let overlayCaption = document.createElement("figcaption");
overlayCaption.textContent = this.alt;
figureBox.appendChild(overlayCaption);
// Add a close button to the overlay
let closeBox = document.createElement("div");
closeBox.id = "lbOverlayClose";
closeBox.innerHTML = "×";
closeBox.onclick = function() {
document.body.removeChild(overlay);
}
overlay.appendChild(closeBox);
document.body.appendChild(overlay);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
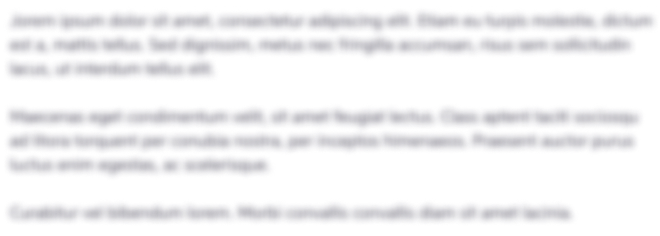
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started