Question
Please help to fix the code 1. import javax.swing.ImageIcon; // 1. Replace the ??? with the appropriate code import ???.NumberFormat; public class Item { private
Please help to fix the code
1.
import javax.swing.ImageIcon;
// 1. Replace the ??? with the appropriate code
import ???.NumberFormat;
public class Item {
private String number;
private String description;
private double price;
private boolean taxable;
private int quantity;
private String imageFile;
private ImageIcon image;
// Constructors:
public Item() {
setNumber("");
setDescription("");
setPrice(0.0);
setTaxable(false);
setQuantity(0);
setImageFile("");
}
// 2. Replace the ??? with the appropriate code
public Item(???) {
setNumber(number);
setDescription(desc);
setPrice(pr);
setTaxable(tx);
setQuantity(q);
setImageFile(imfn);
}
// Mutators:
public void setNumber(String number) {
this.number = number;
}
public void setDescription(String desc) {
this.description = desc;
}
// 3. Replace the ??? with the appropriate code
public void setPrice(???) {
this.price = prc;
}
public void setTaxable(boolean tax) {
this.taxable = tax;
}
public void setQuantity(int quant) {
this.quantity = quant;
}
public void setImageFile(String imfn) {
this.imageFile = imfn;
// 4. Replace the ??? with the appropriate code
this.image = ???;
}
// Accessors:
public String getNumber() {
return this.number;
}
public String getDescription() {
return this.description;
}
public double getPrice() {
return this.price;
}
public boolean getTaxable() {
return this.taxable;
}
public int getQuantity() {
return this.quantity;
}
public String getImageFile() {
return this.imageFile;
}
public ImageIcon getImage() {
return this.image;
}
public String toString() {
NumberFormat fmt = NumberFormat.getCurrencyInstance();
// 5. Replace the ??? with the appropriate code
???;
retVal += "Item Number: "+getNumber()+" ";
retVal += "Description: "+getDescription()+" ";
retVal += "Price: "+fmt.format(getPrice())+" ";
retVal += (getTaxable()?"Taxable":"Non-taxable")+" ";
retVal += "Quantity: "+getQuantity();
// 6. Replace the ??? with the appropriate code
???;
}
}
2.
public class MenuItem ??? Item {
private String prepirationDate;
private boolean glutenFree;
public MenuItem(String number, String desc, double pr, boolean tx, int qty, String prep, boolean gf, String imfn) {
// 2. Replace the ??? with the appropriate code
???;
setPrepirationDate(prep);
setGlutenFree(gf);
}
public MenuItem() {
super("", "", 0.0, false, 0, "");
setPrepirationDate("");
// 3. Replace the ??? with the appropriate code to call the method to set gluten free to true
???;
}
// 4. Replace the ??? with the appropriate code
public void setPrepirationDate(???) {
prepirationDate = prep;
}
public void setGlutenFree(boolean gf) {
glutenFree = gf;
}
public String getPrepirationDate() {
return prepirationDate;
}
// 5. Replace the ??? with the appropriate code
??? getGlutenFree() {
return glutenFree;
}
public String toString() {
String retVal = "";
retVal += super.toString()+" ";
retVal += "Prepiration Date: "+getPrepirationDate()+" ";
// 6. Replace the ??? with the appropriate code
retVal += (getGlutenFree() ??? "GlutenFree" ??? "Non-glutenFree");
return retVal;
}
}
3.
import javax.swing.JOptionPane;
import java.util.Scanner;
import java.util.ArrayList;
import java.io.*;
import java.text.NumberFormat;
public class MenuSelect {
public static final double TAX_RATE = 0.06;
public static void main(String[] args) throws IOException {
// Load ArrayList from Inventory File
ArrayList
// 1. Replace the ??? with the appropriate code
ArrayList
File inFile = new File("oldMenu.txt");
Scanner in = new Scanner(inFile);
MenuItem mi = null;
while (in.hasNext()) {
mi = new MenuItem();
mi.setNumber(in.nextLine());
mi.setDescription(in.nextLine());
mi.setPrice(Double.parseDouble(in.nextLine()));
mi.setTaxable(in.nextLine().charAt(0)=='t');
mi.setQuantity(Integer.parseInt(in.nextLine()));
mi.setPrepirationDate(in.nextLine());
mi.setGlutenFree(in.nextLine().charAt(0)=='t');
// 2. Replace the ??? with the appropriate code
mi.setImageFile(???);
mal.add(mi);
}
in.close();
String[] fiNumbernDesc = new String[mal.size()];
for (int i=0; i fiNumbernDesc[i] = "["+(i+1)+"] "+mal.get(i).getNumber()+": "+ mal.get(i).getDescription(); } boolean invalid; String input = ""; int result=0; String menuChoice; int qtyPurchased; int index; MenuItem mp; // Purchase MenuItems do { // Select item menuChoice = (String) JOptionPane.showInputDialog(null, "Select menu item:", "Menu System", JOptionPane.PLAIN_MESSAGE, null, fiNumbernDesc, fiNumbernDesc[0]); index = Integer.parseInt(menuChoice.substring(1,menuChoice.indexOf("]")))-1; qtyPurchased = 0; // Input Quantity mi = mal.get(index); do { invalid = false; try { input = JOptionPane.showInputDialog(null, mi.toString()+" How many would you like to purchase? ", "Quantity?", JOptionPane.QUESTION_MESSAGE); if (input.length() == 0) throw new Exception("Input is blank."); qtyPurchased = Integer.parseInt(input); if (qtyPurchased < 0) throw new Exception("Cannot purchase a negative quantity."); if (qtyPurchased > mi.getQuantity()) throw new Exception("Menu Item Shortage Cannot purchase more that "+mi.getQuantity()+"."); } // 3. Replace the ??? with the appropriate code ??? (??? e) { invalid = true; JOptionPane.showMessageDialog(null, e.getMessage()+" Please enter the quantity to purchase.", "ERROR", JOptionPane.ERROR_MESSAGE); } } while (invalid); // Add item to mealsPurchased list mp = new MenuItem(); mp.setNumber(mi.getNumber()); mp.setDescription(mi.getDescription()); mp.setPrice(mi.getPrice()); mp.setTaxable(mi.getTaxable()); mp.setQuantity(qtyPurchased); // 4. Replace the ??? with the appropriate code ???.???(mi.getImageFile()); mp.setPrepirationDate(mi.getPrepirationDate()); mp.setGlutenFree(mi.getGlutenFree()); mealsPurchased.add(mp); // Subtract Quantity from mal ArrayList mi.setQuantity(mi.getQuantity()-qtyPurchased); mal.set(index, mi); // 5. Replace the ??? with the appropriate code } while (JOptionPane.???(null, "Would you like to select another item?", "Another?", JOptionPane.YES_NO_OPTION) == ???); // Display Items Purchased and Subtotal, Tax and Total Sale double subtotal = 0.0; double lineItem = 0.0; double tax = 0.0; double total; String purchasedItem; // 6. Replace the ??? with the appropriate code NumberFormat ??? = ???.getCurrencyInstance(); for (MenuItem p:mealsPurchased) { lineItem = p.getPrice() * p.getQuantity(); // 7. Replace the ??? with the appropriate code JOptionPane.showMessageDialog(???, p.toString()+" Total Cost: "+fmt.format(lineItem), p.getDescription(), JOptionPane.PLAIN_MESSAGE, p.???()); subtotal += lineItem; if (p.getTaxable()) tax += lineItem * TAX_RATE; } // 8. Replace the ??? with the appropriate code total = ???; JOptionPane.showMessageDialog(null, "Meal Reciept ================== Subtotal: "+ fmt.format(subtotal)+" Taxes: "+fmt.format(tax)+" Total: "+fmt.format(total), "Meal Total", JOptionPane.INFORMATION_MESSAGE); // Save ArrayList to new Inventory File File outFile = new File("newMenu.txt"); PrintWriter out = new PrintWriter(outFile); // Write all of the items in the arraylist to the new inventory file. for (int i=0; i out.println(mal.get(i).getNumber()); out.println(mal.get(i).getDescription()); out.println(mal.get(i).getPrice()); out.println(mal.get(i).getTaxable()); out.println(mal.get(i).getQuantity()); out.println(mal.get(i).getPrepirationDate()); out.println(mal.get(i).getGlutenFree()); out.println(mal.get(i).getImageFile()); } out.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
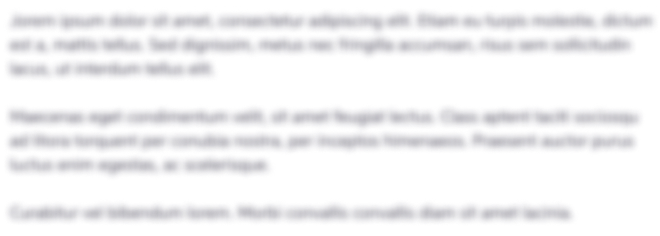
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started