Question
PLEASE HELP USING C HERE IS THE CODE GIVEN int main() { int ch; char *pos; char s[MAX_BUF+2]; // 2 extra for the newline and
PLEASE HELP USING C
HERE IS THE CODE GIVEN
int main() { int ch; char *pos; char s[MAX_BUF+2]; // 2 extra for the newline and ending '\0' static const char prompt[] = "msh> "; char *toks[MAX_TOKS];
// // YOUR CODE HERE (add declarations as needed) //
while (1) { // prompt for input if input from terminal if (isatty(fileno(stdin))) { printf(prompt); }
// read input char *status = fgets(s, MAX_BUF+2, stdin);
// exit if ^d entered if (status == NULL) { printf(" "); break; }
// input is too long if last character is not newline if ((pos = strchr(s, ' ')) == NULL) { printf("error: input too long "); // clear the input buffer while ((ch = getchar()) != ' ' && ch != EOF) ; continue; }
// remove trailing newline *pos = '\0';
// // YOUR CODE HERE //
} exit(EXIT_SUCCESS); }
Your job is to extend the code in the following ways: split the input string s into an array of strings (array toks[]) if there are no tokens, just prompt for more input o if the first token is 'exit', exit the program if the first token is 'help', print some help information if the first token is 'today', print today's date otherwise, print the tokens that were input 0 Here is an example of what your msh code should do and how to format outputs: ($ is the bash prompt) $ ./msh1 msh> help enter 'help', 'today', or 'exit' to quit msh> today 02/01/2020 msh> exit $ $ ./msh1 msh> wc -1 token: 'We' token: '-1' msh> lst token: 'ls' token: '-t' msh> Implementing the 'exit' and 'help' commands is easy. There are two parts to this assignment that will take a little thinking: 1. splitting the input string s into tokens array toks[] You must use function strtok() or strtok_r() for this. The man page for strtok() is scary, but using strtok() is not hard. To get the first token in string s, you call strtok(s," "). The second argument means that s should be split into tokens based on space characters. To get the second token in s, you call strtok(NULL, ""). If will return NULL if there is no second token. To get the third token in s you call strtok(NULL, "") again. In summary, you provide the string s in the first call but NULL in later calls, and keep calling until NULL is returned. 2. implementing the 'today' command You must use Linux commands time() and localtime() for this. Try 'man 2 time', and 'man localtime'. You will first call time() and then call localtime(). It's a little tricky. You must use system calls or C library functions to get the time, not user commands (look at the man page for 'man' if you don't know the difference). For these two parts, I recommend you write separate small test programs to make sure you understand how to use the calls. Your job is to extend the code in the following ways: split the input string s into an array of strings (array toks[]) if there are no tokens, just prompt for more input o if the first token is 'exit', exit the program if the first token is 'help', print some help information if the first token is 'today', print today's date otherwise, print the tokens that were input 0 Here is an example of what your msh code should do and how to format outputs: ($ is the bash prompt) $ ./msh1 msh> help enter 'help', 'today', or 'exit' to quit msh> today 02/01/2020 msh> exit $ $ ./msh1 msh> wc -1 token: 'We' token: '-1' msh> lst token: 'ls' token: '-t' msh> Implementing the 'exit' and 'help' commands is easy. There are two parts to this assignment that will take a little thinking: 1. splitting the input string s into tokens array toks[] You must use function strtok() or strtok_r() for this. The man page for strtok() is scary, but using strtok() is not hard. To get the first token in string s, you call strtok(s," "). The second argument means that s should be split into tokens based on space characters. To get the second token in s, you call strtok(NULL, ""). If will return NULL if there is no second token. To get the third token in s you call strtok(NULL, "") again. In summary, you provide the string s in the first call but NULL in later calls, and keep calling until NULL is returned. 2. implementing the 'today' command You must use Linux commands time() and localtime() for this. Try 'man 2 time', and 'man localtime'. You will first call time() and then call localtime(). It's a little tricky. You must use system calls or C library functions to get the time, not user commands (look at the man page for 'man' if you don't know the difference). For these two parts, I recommend you write separate small test programs to make sure you understand how to use the callsStep by Step Solution
There are 3 Steps involved in it
Step: 1
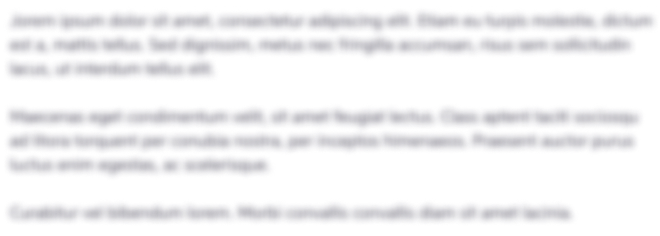
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started