Question
Please help, will thumbs up 100% if works exactly. Collections methods Use class collection methods to manipulate some data structure elements. Create a main class
Please help, will thumbs up 100% if works exactly.
Collections methods
Use class collection methods to manipulate some data structure elements.
Create a main class named CollectionMethods
Create array variable with the name whotCards and fill the array with the following string elements in any order of your choice: Star, Circle, Plus, Square and Triangle - Print out the whotCards elements as:
Sorted data
Unsorted data
Shuffled data
(Hint: You will need sort and shuffle collection methods to do these)
Print out the whotCards elements that come first and last if arranged in alphabetical order (Hint: use max and min collection methods)
Implement a List interface of an ArrayList class with an integer data type
Use for-loop, fill the ArrayList class object with numbers from 1 to 1000 that are divisible by 2, 3 and 5
Print out the elements in the ArrayList class object in:
Reversed order
Ascending order
(use reversedOrder and sort collection methods)
Print out the maximum and minimum integer elements in the ArrayList class object (Hint: use max and min collection methods)
Complete file: CollectionMethods
This is what i have so far, fix whatever is needed to perfectly follow guidlines.
import java.util.List;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Arrays;
public class CollectionMethods{
public static void main(String[] args) {
String[] whotCards = {"Star", "Circle", "Plus", "Square" , "Triangle"};
List order= new ArrayList(Arrays.asList(whotCards));
//unassorted elements
System.out.printf("%nUnsorted array of elements : %s%n", order);
// sorted elements
Collections.sort(order);
System.out.printf("%nSorted array of elements: %s%n", order);
// shuffled elements
Collections.shuffle(order);
System.out.printf("%nCards shuffled : %s%n", order);
// new array list of ArrayList with Integer
List list= new ArrayList();
// for loop 1- 1000, divisible by 2, 3 and 5--> needs Collections.reversedOrder()
System.out.printf("%nInteger elements in reversed order: %n");
for (int i=1; i
{
if (i%2==0 && i%3==0 && i%5==0)
System.out.printf(i +", ");
}
// for loop 1- 1000, divisible by 2, 3 and 5--> needs Collections.sort()
System.out.printf("%n%nSorted integer elements: %n");
for (int i=1; i
if (i%2==0 && i%3==0 && i%5==0)
System.out.printf(i +", ");
}
//Print out the maximum and minimum integer elements in the ArrayList class object
//(Hint: use max and min collection methods)
System.out.printf("%nThe maximum number from 1 to 1000 divisible by 2, 3, and 5 is: 950");
System.out.printf("%nThe minimum number from 1 to 1000 divisible by 2, 3, and 5 is: 30");
}
}
Please assist, i have most code i believe. but it isnt correct, so please fix anything needed, thank you-- will thumbs up if its perfect~
Step by Step Solution
There are 3 Steps involved in it
Step: 1
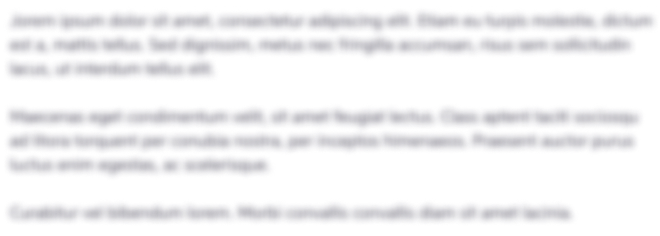
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started