Question
Please help with comlier error driving me crazy. /** * AWT Sample application * * @author * @version 1.00 17/05/19 */ import java.io.*; import java.util.*;
Please help with comlier error driving me crazy.
/** * AWT Sample application * * @author * @version 1.00 17/05/19 */ import java.io.*; import java.util.*;
//declare paerson class with implementung a conable interface public class Person implements Cloneable { //we can still use the same variables inside the private class private String name; private Date born; private Date died; //immplement the private date class withing the person class private class Date { private String month; private int day; private int year; //a four digit number. public Date( ) { month = "January"; day = 1; year = 1000; }
public Date(int monthInt, int day, int year) { setDate(monthInt, day, year); }
public Date(String monthString, int day, int year) { setDate(monthString, day, year); }
public Date(int year) { setDate(1, 1, year); }
public Date(Date aDate) { if (aDate == null)//Not a real date. { System.out.println("Fatal Error."); System.exit(0); }
month = aDate.month; day = aDate.day; year = aDate.year; }
public void setDate(int monthInt, int day, int year) { if (dateOK(monthInt, day, year)) { this.month = monthString(monthInt); this.day = day; this.year = year; } else { System.out.println("Fatal Error"); System.exit(0); } }
public void setDate(String monthString, int day, int year) { if (dateOK(monthString, day, year)) { this.month = monthString; this.day = day; this.year = year; } else { System.out.println("Fatal Error"); System.exit(0); } }
public void setDate(int year) { setDate(1, 1, year); }
public void setYear(int year) { if ( (year < 1000) || (year > 9999) ) { System.out.println("Fatal Error"); System.exit(0); } else this.year = year; } public void setMonth(int monthNumber) { if ((monthNumber <= 0) || (monthNumber > 12)) { System.out.println("Fatal Error"); System.exit(0); } else month = monthString(monthNumber); }
public void setDay(int day) { if ((day <= 0) || (day > 31)) { System.out.println("Fatal Error"); System.exit(0); } else this.day = day; }
public int getMonth( ) { if (month.equals("January")) return 1; else if (month.equals("February")) return 2; else if (month.equalsIgnoreCase("March")) return 3; else if (month.equalsIgnoreCase("April")) return 4; else if (month.equalsIgnoreCase("May")) return 5; else if (month.equals("June")) return 6; else if (month.equalsIgnoreCase("July")) return 7; else if (month.equalsIgnoreCase("August")) return 8; else if (month.equalsIgnoreCase("September")) return 9; else if (month.equalsIgnoreCase("October")) return 10; else if (month.equals("November")) return 11; else if (month.equals("December")) return 12; else { System.out.println("Fatal Error"); System.exit(0); return 0; //Needed to keep the compiler happy } }
public int getDay( ) { return day; }
public int getYear( ) { return year; }
public String toString( ) { return (month + " " + day + ", " + year); }
public boolean equals(Date otherDate) { if (otherDate == null) return false; else return ( (month.equals(otherDate.month)) && (day == otherDate.day) && (year == otherDate.year) ); }
public boolean precedes(Date otherDate) { return ( (year < otherDate.year) || (year == otherDate.year && getMonth( ) < otherDate.getMonth( )) || (year == otherDate.year && month.equals(otherDate.month) && day < otherDate.day) ); }
public void readInput( ) { boolean tryAgain = true; Scanner keyboard = new Scanner(System.in); while (tryAgain) { System.out.println("Enter month, day, and year."); System.out.println("Do not use a comma."); String monthInput = keyboard.next( ); int dayInput = keyboard.nextInt( ); int yearInput = keyboard.nextInt( ); if (dateOK(monthInput, dayInput, yearInput) ) { setDate(monthInput, dayInput, yearInput); tryAgain = false; } else System.out.println("Illegal date. Reenter input."); } }
private boolean dateOK(int monthInt, int dayInt, int yearInt) { return ( (monthInt >= 1) && (monthInt <= 12) && (dayInt >= 1) && (dayInt <= 31) && (yearInt >= 1000) && (yearInt <= 9999) ); }
private boolean dateOK(String monthString, int dayInt, int yearInt) { return ( monthOK(monthString) && (dayInt >= 1) && (dayInt <= 31) && (yearInt >= 1000) && (yearInt <= 9999) ); }
private boolean monthOK(String month) { return (month.equals("January") || month.equals("February") || month.equals("March") || month.equals("April") || month.equals("May") || month.equals("June") || month.equals("July") || month.equals("August") || month.equals("September") || month.equals("October") || month.equals("November") || month.equals("December") ); }
private String monthString(int monthNumber) { switch (monthNumber) { case 1: return "January"; case 2: return "February"; case 3: return "March"; case 4: return "April"; case 5: return "May"; case 6: return "June"; case 7: return "July"; case 8: return "August"; case 9: return "September"; case 10: return "October"; case 11: return "November"; case 12: return "December"; default: System.out.println("Fatal Error"); System.exit(0); return "Error"; //to keep the compiler happy } }
public Object clone( ) { try { return super.clone( );//Invocation of //clone in the base class Object } catch(CloneNotSupportedException e) {//This should not happen. return null; //To keep compiler happy. } } /* //constructors public Date(); public Date(int monthInt, int day, int year); public Date(int year); public Date(Date aDate); //to catch all diiferent inputs //methods to set public void setDate(int monthInt, int day, int year); public void setDate(String monthString, int day, int year); public void setDate(int year); //set date/year/month/day allos suer to set date sepeding //on differnet paramenter public void setYear(int year); public void setMonth(int monthNumber); public void setDay(int day); //methods to get month day and year of date class public int getMonth(); public int getDay(); public int getYear(); //getters allow the user to acces the private mems of the date object public String toString(); //changes outute of date object from memory address to a string defined //in the method public boolean equals(Date otherDate); //equals methods returns true if the ars other date contains the //same month day and year as the date object false otherwise public boolean precedes(Date otherDate); //precedes method rturns ture if the arg other date contains a date that //comes before the date of the Date object public void readInput(); //allos the user to input specific day month and year values private boolean dateOK(int monthString, int dayInt, int yearInt); //date ok method checks if date is valid private boolean dateOK(String monthString, int dayInt, int yearInt); private boolean monthOK(String month); // month ok checks to see if string contains the proper speling //of the month private String monthString(int monthNumber); //takes in a month as an int and returns its repective month //since date objecr now cannot be directly created out of person class //the brth dates and death dates for the new person class must be //doine in the constructor */ } public Person(String initalName) { Date birthDate; Date deathDate; //scanner Scanner scan = new Scanner(System.in); System.out.println("Enter birth date information: "); System.out.print("month (number):"); int month = scan.nextInt(); System.out.print("Day:"); int day = scan.nextInt(); System.out.print("Year:"); int year = scan.nextInt(); birthDate = new Date(month, day, year); scan.nextLine(); //must ask the person if they are dead or not System.out.println("Is person still alive? (Y/N)"); String alive = scan.nextLive(); if(!alive.equalsIgnoreCase("y")) { System.out.println("Enter Death Date information: "); System.out.print("Month (number):"); month = scan.nextInt(); System.out.print("Day:"); day = scan.nextInt(); System.out.print("Year:"); year = scan.nextInt(); deathDate = new Date(month, day, year); scan.nextLine(); } else deathDate = null; //check if the date object are consisnet death year> that birth year if(consistent(birthDate, deathDate)) { name = initialName; born = new Date(birthDate); if(deathDate == null) died = null; else died = new Date(deathDate); } else { System.out.println("Inconsistent dates. Aborting. "); System.exit(0); } }//end of paramentrized contructor person calss //the copy constructor for the calss should not change with the implementation //of the new private calss since date calss is defined along with person //object public Person(Person original) { if(original == null) { System.out.println("Fatal error."); System.exit(0); } name = original.name; born = new Date(orginal.born); if(original.died == null) died = null; else died = new Date(original.died); }//end of person class //set method shoudl now also take no date parameter for implemt public void set(String newName) { name = newName; //input values for a new birh date object Scanner scan = new Scanner(System.in); System.out.println("Enter birth date info: "); System.out.print("Month (number):"); int month = scan.nextInt(); System.out.print("Day:"); int day = scan.nextInt(); System.out.print("Year:"); int year = scan.nextInt(); birthDate = new Date(month, day, year); scan.nextLine(); //must ask the person if they are dead or not System.out.println("Is person still alive? (Y/N)"); String alive = scan.nextLive(); if(!alive.equalsIgnoreCase("y")) { System.out.println("Enter Death Date information: "); System.out.print("Month (number):"); month = scan.nextInt(); System.out.print("Day:"); day = scan.nextInt(); System.out.print("Year:"); year = scan.nextInt(); deathDate = new Date(month, day, year); scan.nextLine(); } else deathDate = null; //check if the date object are consisnet death year> that birth year if(!consistent(birthDate, deathDate)) { System.out.println("Inconsistent dates. Aborting. "); System.exit(0); } born = new Date(birthDate); if(deathDate == null) died = null; else died = new Date(deathDate); }//end of set method public String toString() { String diedString; if(died == null) diedString = ""; else diedString = died.toString(); return name + "," + born + "-" + diedString; } public boolean equals(Person otherPerson) { if(otherPerson == null) return false; else return (name.equals(otherPerson.name) && born.equals(otherPerson.born) && datesMatch(died, otherPerson.died)); } //datesMactch method should compare two different date objects to see if they //match private static boolean datesMatch(Date date1, Date date2) { if(date1 == null) return (date2 == null); else if(date2 == null) return false; else return date1.equals(date2); } //setbirth date remove date parameter take inout from user public void setBirthDate() { Scanner scan = new Scanner(System.in); System.out.println("Enter birth date info: "); System.out.print("Month (number):"); int month = scan.nextInt(); System.out.print("Day:"); int day = scan.nextInt(); System.out.print("Year:"); int year = scan.nextInt(); birthDate = new Date(month, day, year); scan.nextLine(); //check to see if the new birth date is constient with death if(!consistent(newDate, died)) { System.out.println("Inconsisten dates, aborting. "); System.exit(0); } else born = new Date(newDate); } //death date as well should be cahnged to user input public void setDeathDate() { Scanner scan = new Scanner(System.in); //check to seee if person is alive System.out.println("Is person still alive? (Y/N)"); String alive = scan.nextLine(); Date newDate; if(!alive.equalsIgnoreCase("y")) { System.out.println("enter death date info: "); System.out.print("Month (number):"); int month = scan.nextInt(); System.out.print("Day:"); int day = scan.nextInt(); System.out.print("Year:"); int year = scan.nextInt(); birthDate = new Date(month, day, year); scan.nextLine(); } else newDate = null; //check to see if the new death date is in live with the birth date if(!consistent(born, newDate)) { System.out.println("Inconsisten dates, aborting. "); System.exit(0); } else died = new Date(newDate); } //setname public void setName(String newName) { name = newName; } //mutator method birth year only modifies the year within the date object public void setBirthYear(int newYear) { if (died == null) { System.out.println("Fatal error, aborting."); System.exit(0); } born.setYear(newYear); if(!consistent(born, died)) { System.out.println("Inconsisten dates, aborting. "); System.exit(0); } } public void setDeathYear(int newYear) { if (died == null) { System.out.println("Fatal error, aborting."); System.exit(0); } died.setYear(newYear); if(!consistent(born, died)) { System.out.println("Inconsisten dates, aborting. "); System.exit(0); } } ////accesors methods public String getName() { return name; } public Date getBirthDate() { return new Date(born); } public Date getDeathDate() { if(died == null) return null; else return new Date(died); } //consistent method checks if the first date object is in line with //the second Date object private static boolean consistent(Date birthDate, Date deathDate) { if(birthDate == null) return false; else if(deathDate == null) return true; else return (birthDate.precedes(deathDate) || birthDate.equals(deathDate)); } //override the clone method of the generic object class this shloud //return a deep copy of person object that calls this method use the copy //contructor of the person object to do so public Person clone() { return new Person(this); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
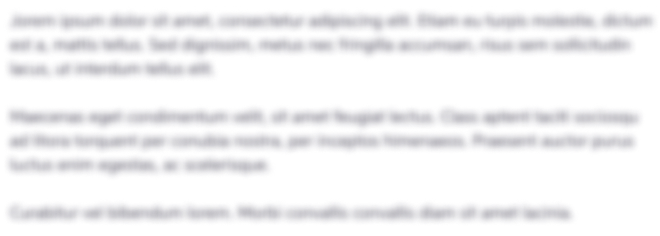
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started