Question
Please help with converting below code to python language CODE: package com.src; import java.util.Locale; import java.util.Objects; import java.util.Random; class RandomString { /** * Generate a
Please help with converting below code to python language
CODE:
package com.src;
import java.util.Locale;
import java.util.Objects;
import java.util.Random;
class RandomString {
/**
* Generate a random string.
*/
public String nextString() {
for (int idx = 0; idx < buf.length; ++idx)
buf[idx] = symbols[random.nextInt(symbols.length)];
return new String(buf);
}
public static final String upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
public static final String lower = upper.toLowerCase(Locale.ROOT);
public static final String space = " ";
public static final String alphanum = upper + lower + space;
private final Random random;
private final char[] symbols;
private final char[] buf;
public RandomString(int length, Random random, String symbols) {
if (length < 1) throw new IllegalArgumentException();
if (symbols.length() < 2) throw new IllegalArgumentException();
this.random = Objects.requireNonNull(random);
this.symbols = symbols.toCharArray();
this.buf = new char[length];
}
/**
* Create an alphanumeric string generator.
*/
public RandomString(int length, Random random) {
this(length, random, alphanum);
}
/**
* Create an alphanumeric strings from a secure generator.
*/
public RandomString(int length) {
this(length, new Random());
}
/**
* Create session identifiers.
*/
public RandomString() {
this(21);
}
}
public class Assignment1Demo {
private static String msg;
private static String msgE;
private static String msgD;
private static int key;
public static void main(String[] args){
//TODO: You can only call methods in main method
key = generateKey();
msg = generateMsg();
msgE = encryption(key,msg);
bruteForce(msgE);
}
private static int generateKey() {
//TODO: implement step a (randomly generate 16-bit key)
//16 bit digit means 2^16 -1 in decimal
Random rand = new Random();
return rand.nextInt((int) (Math.pow(2, 16)-1));
}
private static String generateMsg() {
//TODO: implement step b (randonly generate a string with an even number of characters)
RandomString str = new RandomString();
String randString = str.nextString();
if(randString.length() %2 != 0)
randString += " ";
return randString;
}
private static String encryption(int key, String msg) {
//TODO: implement step c (encrypt the message)
String Key = Integer.toString(key);
for(int i=0;i<=msg.length()/2;i++){
Key += Key;
}
return (msg + key);
}
private static void decryption(int key, String msgE) {
//TODO: implement step d (decryption)
String Key = Integer.toString(key);
for(int i=0;i<=msg.length()/2;i++){
Key += Key;
}
msgD = msgE + Key;
}
private static void bruteForce(String msgE) {
//TODO: implement bruteForce algorithm, you may need the above decryption(key,msgE) method
boolean isEnglisString = msgE.matches("[a-zA-Z]+");
if(isEnglisString)
System.out.println("Yes encrypted message is Randomly English generated message " + msgE);
else
System.out.println("encrypted message is Not Randomly english generated message "+msgE);
decryption(key, msgE);
isEnglisString = msgD.matches("[a-zA-Z]+");
if(isEnglisString)
System.out.println("Yes decrypted message is Randomly english generated message "+ msgD);
else
System.out.println("decrypted message is not Randomly english generated message "+ msgD);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
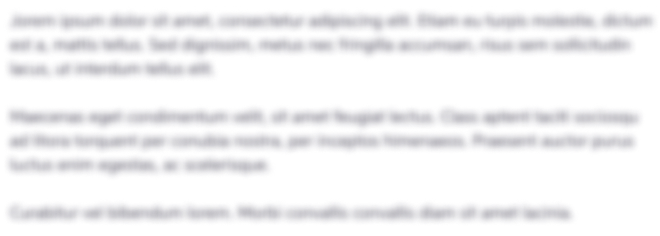
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started