Question
Please help with the following C programming problem: 1. Follow comments instructions in the main() to create a dynamic array of 10 integers, assign them
Please help with the following C programming problem:
1. Follow comments instructions in the main() to create a dynamic array of 10 integers, assign them with random values (0-100, inclusive), sort, and print them. You will implement everything up till Step 2 in the main() as well as the
sort(int *arr, int n)
function.
2. Change the prototype of sort() function from
sort(int *arr, int n);
To
void sort(void** arr, int n, int(*cmp)(void* a, void* b));
Now this function is able to sort an array of a generic type (void*). The new sort() function also needs to take a function pointer as its 3rd argument to determine how to sort its elements. Modify the sort() function using function pointer as well as the code you implemented in Step 1, i.e., the function call.
3. Follow comments instructions in the main() to create a dynamic array of 10 students, assign them with random IDs (0-100, inclusive) and scores (0-100,
4. Free the memory allocated in Step1-3. You will need to implement the free_arr() function.
Given Code:
dynarray.c:
#include
#include
#include
#include
#include
struct student{
int id;
int score;
};
//helper function to compare students by their scores
int compare_student (void* x, void* y){
struct student *s1 = x;
struct student *s2 = y;
return s1->score > s2->score ? 1 : 0;
}
//helper function to compare two ints
int compare_int (void* x, void* y){
int* a = x, *b = y;
return *a > *b ? 1 : 0;
}
/* sort the arr array by using a function pointer to
* compare its elements
* Param:
* void** arr - array of generic type
* int n - array size
* int(*cmp)(void* a, void* b)- function pointer to compare two elements in the array
*/
//void sort(void** arr, int n, int(*cmp)(void* a, void* b))
void sort(int* arr, int n){
/*Sort n integers in arr, ascending order*/
/*
* FIXED ME:
*/
}
/* free the memeory allocated by arr
* Param:
* void** arr - array of generic type
* int n - array size
*/
void free_arr (void** arr, int n){
/* free the memory allocated by arr*/
/*
* FIXED ME:
*/
}
int main(){
srand(time(NULL));
/*Declare an integer n and assign 10 to it.*/
/***Step 1: sort an integer array ***/
/*Allocate memory for n integers using malloc.*/
/*Generate random ints (0-100, inclusive) for the n integers, using rand().*/
/*Print the contents of the array of n students.*/
printf(" Before sorting.... ");
/*call sort() function to sort*/
/*Print the contents of the array of n students.*/
printf(" After sorting.... ");
/***Step 2: After modifying sort() by using function pointer, modify your sort() function call above ****/
/***Step 3: sort an array of students ***/
/*Allocate memory for n students using malloc.*/
/*Generate random IDs and scores for the n students, using rand().*/
/*note:
* 1. no two students should have the same ids
* 2. score and id range: 0-100, inclusive
*/
/*Print the contents of the array of n students.*/
printf(" Before sorting.... ");
/*call sort() function to sort*/
/*Print the contents of the array of n students.*/
printf(" After sorting.... ");
/***Step 4: free the memory allocated in Step 1-3 by calling free_arr() ***/
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
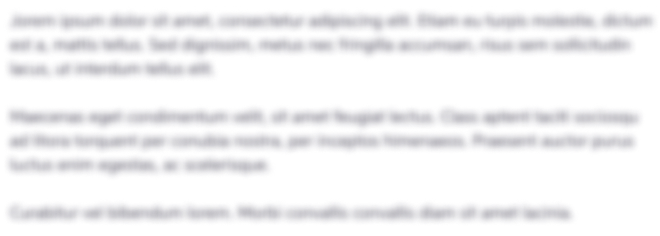
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started