Question
Please help with the following: how would you answer this question Using the class Robot, write a java program to simulate a robot race. Your
Please help with the following: how would you answer this question
Using the class Robot, write a java program to simulate a robot race. Your program should ask the user the size of the grid, and the number of robots that will race, as well as their names. The robot objects must be stored in an array. The winner is the 1st robot to reach the top right corner of the grid.
Your program must work for any size grid and any number of robots. Make sure the number of robots is at least 1 and that the grid size is at least 2x2. Make use of the static methods you wrote for the driver with the code provided. (Write a static method to read and validate the number of robots.)
With the following code: In Java
public class Robot { //instance variables private String name; //stores name of the robot private int x; //x coordinate of robot position private int y; //y coordinate of robot position private char direction; //E W N S //constructors public Robot(){ name = "noName"; x=0; y=0; direction = 'E'; } public Robot(String name){ this.name = name; x=0; y=0; direction = 'E'; } //copy constructor public Robot(Robot r){ name = r.name; x=r.x; y=r.y; direction = r.direction; } //getters public String getName(){ return name; } public char getDirection(){ return direction; } public int getX(){ return x; } public int getY(){ return y; } public void setName(String name){ this.name = name; } @Override public String toString(){ return name+" is facing "+direction +" and at position ("+x+", "+y+")"; } public boolean equals(Object o){ if(o == null) return false; if(!(o instanceof Robot)) return false; Robot r = (Robot)o; return((direction == r.direction) && (x == r.x) && (y == r.y)); } public void changeDirection(){ if(direction=='E') direction = 'N'; else if(direction=='W') direction = 'S'; else if(direction=='N') direction = 'W'; else if(direction=='S') direction = 'E'; } public void move(int noOfSteps,int gridSize){ switch(direction){ case 'E': if( x+noOfSteps = 0){ x = x-noOfSteps; }else{ int xold = x; x = 0; changeDirection(); move((noOfSteps - xold),gridSize); } break; case 'N': if( y+noOfSteps = 0){ y = y-noOfSteps; }else{ int yold = y; y = 0; changeDirection(); move((noOfSteps - yold),gridSize); } break; } } //end of method move() public boolean won(int gridSize){ return((x==gridSize) && (y == gridSize)); } //end of method won() }
RobotDriver.java
import java.util.Scanner; import java.util.Random; public class RobotDriver { public static void displayHeader(){ System.out.println(" = 0 = 0 = 0 = 0 = 0 = 0 = 0 ="); System.out.println(" = 0 0 ="); System.out.println(" = 0 Robot Walk 0 ="); System.out.println(" = 0 0 ="); System.out.println(" = 0 = 0 = 0 = 0 = 0 = 0 = 0 ="); } public static int readGridSize(Scanner sc){ int s; System.out.print("What is the size of your grid ? "); s = sc.nextInt(); sc.nextLine(); return s; } public static void displayClosingMessage(Robot r,int moves){ System.out.println(r.getName()+" reached its final destination in "+moves+" moves."); } public static void main(String[] args) { displayHeader(); Scanner sc = new Scanner(System.in); Random rnd = new Random(); String name; int gridSize; int moves=0; System.out.print("What is name of your Robot ? "); name = sc.nextLine(); gridSize = readGridSize(sc); Robot myRobot = new Robot(name); System.out.println("Time for "+name+" to start walking !!!"); System.out.println("At start "+name+" is facing E and at position (0,0)"); while (!myRobot.won(gridSize)) { moves++; int noOfSteps = rnd.nextInt(gridSize)+1; System.out.println("==> Number of steps to take: "+noOfSteps+"."); myRobot.move(noOfSteps, gridSize); System.out.println(" Result: "+name+" is facing "+myRobot.getDirection()+" and at position ("+myRobot.getX()+", "+myRobot.getY()+")."); } displayClosingMessage(myRobot,moves); } }
It should look like this
Step by Step Solution
There are 3 Steps involved in it
Step: 1
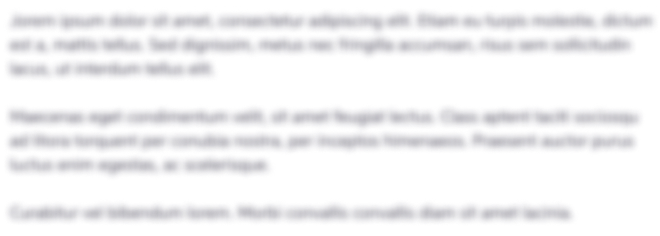
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started