Question
Please help with the following. I am not sure if i am to convert my previous code to match these directions or just use these
Please help with the following. I am not sure if i am to convert my previous code to match these directions or just use these directions and make adjustments to the code being provided in the prompt. Here is what is being asked of me to do:
whether the shape of steel is a Plate or a Rod. For example:
Enter shape and dimensions: P 1/2 2 5 4 8
Weight: 229.78471
Enter shape and dimensions: R 3 Goals
Learn test-driven development.
Learn how to parse input line of text into multiple values.
Learn how to use single dimensional arrays.
Learn how to write and use methods to simplify and reduce the code.
Learn how to use constants and the pre-defined Java constant PI.
Problem
The Weight program is useful, but but the Acme company is expanding their offerings to plates of any
fractional thickness (e.g. 1/8, 3/16...).
In addition, Acme is expanding into more than just plates. They are now adding steel rods to their inventory
in addition to the plates. Rods are just cylinders of steel. To calculate their weight, use the following
formula:
weight = ?r^2 ? length ? 489lb/ft^3
Where r is the radius of the rod in feet (ft) and length is also in feet.
User Story
Users want essentially the same interface as the current Weight program but the input now has to indicate
/4 5 7
Weight: 26.313377
Enter shape and dimensions: Enter nothing and program terminates
Tasks
1. Create a new project called Weight3.
2. Copy and paste this into Weight3.java file in the project.
package weight3;
import java.util.Scanner;
public class Weight3 {
static boolean TESTIT = true;
final static int PLATE = 0;
final static int ROD = 1;
private static double thickness(String thickFrac) {
double thickness = 0;
// Turn *any* string fraction (e.g. "1/4") into its
// double value (e.g. .25). This takes in any fraction so no
// switch statement will work.
// Hints: Split on "/", used Integer.parseInt() on numerator and
// denominator. Divide the two and then divide by 12.
return thickness;
}
private static double[] parseInput(String line) {
String[] input_values;
double[] values;
int feet;
int inches;
// The given parameter "line" string will look like this:
// "P 1/4 5 6 4 5" or
// "R 1/4 8 2"
// You will return an array of doubles that will look something
// like this:
// {0, .0208, 5.5, 4.4166} for "P 1/4 5 6 4 5"
// {1, .0208, 8.1666} for "R 1/4 8 2"
input_values = line.split(" ");
if (input_values[0].equals("P")) {
values = new double[4];
values[0] = PLATE;
values[1] = .0208; // Replace this by using thickness() above for this.
feet = Integer.parseInt(input_values[2]);
inches = Integer.parseInt(input_values[3]);
values[2] = 5.0; // Replace this by using feet and inches.
feet = Integer.parseInt(input_values[3]);
inches = Integer.parseInt(input_values[4]);
values[3] = 6.0; // Replace this by using feet and inches.
} else if (input_values[0].equals("R")){
values = new double[3];
values[0] = ROD;
values[1] = .0208; // Use logic similar to the above to set this
values[2] = 5.0; // Use logic similar to the above to set this
}
else {
values = new double[0];
}
return values;
}
private static double weight(double thick, double width, double length) {
double weight = 0;
// (Plate) Thick x Width x Length weight calculation here.
// System.out.println(thick + " " + width + " " + length);
return weight;
}
private static double weight(double diameter, double length) {
double weight = 0;
// (Rod) Area of circle x Length weight calculation here.
return weight;
}
private static int test() {
int errors = 0;
double thick;
thick = thickness("1/2");
if (thick != .5/12) {
System.out.println("thickess FAILED thick=" + thick);
++errors;
}
double[] values;
values = parseInput("P 1/4 5 6 4 0");
if (values[0] != 0 || values[1] != .25 || values[2] != 5.5 || values[3] != 4.0) {
System.out.println("parseInput FAILED thick=" + values[1] +
" width=" + values[2] + " length=" + values[3]);
++errors;
}
return errors;
}
public static void main(String[] args) {
if (TESTIT) {
int errors = test();
System.exit(errors);
}
Scanner input = new Scanner(System.in);
String line;
double[] values;
double weight;
while (true) {
System.out.print("Enter shape and dimensions: ");
line = input.nextLine();
values = parseInput(line);
if (values.length == 0) {
break;
}
weight = 0;
switch ((int) values[0]) {
case PLATE:
weight = weight(values[1], values[2], values[3]);
break;
case ROD:
// Put rod code here. Note that rods do not need a width
// so copy the above code but make the appropriate changes
// to exclude width.
break;
default:
System.out.println("Unknown shape");
break;
}
if (weight != 0) {
System.out.print("Weight: ");
System.out.println(weight);
}
}
}
}
3. Run it immediately and you should see the output.
run:
thickess FAILED thick=0.0
parseInput FAILED thick=0.0208 width=5.0 length=6.0
some-path\AppData\Local\NetBeans\Cache\8.2\executor-snippets un.xml:53: Java
returned: 2
BUILD FAILED (total time: 0 seconds)
4. First get rid of these test failures by implementing:
a. thickness()
b. parseInput()
5. Implement the remaining code indicated by the bold comments in the above code.
6. Change TESTIT to false and test with the test items below.
Checklist
Use the following as guidance for getting a 10 on this project:
Working thickness(). (2 points)
Working parseInput(). (3 points)
Working weight() for plates. (1 points)
Working weight() for rods. (2 points)
Follows conventions, has correct prompt, has correct output. (2 points)
Shape Thick. Or Diameter WidthFt. Width In. LengthFt. LengtIn. Weight
P 1/4 5 6 2 3 126.07
R 3/4 5 7 8 .376302
This is my previous code I made(please be aware I am not sure if i am to use this code or the above):
package weight;
import java.util.Scanner;
public class Weight {
public static void main(String[] args) { double thickness_in; double thickness_ft;
/** * Start of loop */ while (true) {
System.out.print("Enter Dimensions: "); Scanner input = new Scanner(System.in);
thickness_in = 0; //getting thickness fraction as string String thickness_frac = input.next();
if (thickness_frac.equals("end")) { //when "end" entered end loop break;
} /** * switching thickness fraction to identify thickness_in */ switch (thickness_frac) {
case "1/8": thickness_in = (float) 0.125; break;
case "1/4": thickness_in = (float) 0.25; break;
case "1/2": thickness_in = (float) 0.5; break; } /** * If thickness is == 0 then print out error message */ if (thickness_in == 0) {
System.out.println("Error: The plate thickness is not an allowed thickness"); } else { //valid fraction, calculating other values thickness_ft = thickness_in / 12.0; int width_in_ft = input.nextInt(); int width_in_in = input.nextInt();
double width_ft = width_in_ft + width_in_in / 12.0; int length_in_ft = input.nextInt(); int length_in_in = input.nextInt();
double length_ft = length_in_ft + length_in_in / 12.0;
System.out.println("Weight: " + (thickness_ft * width_ft * length_ft * 489)); }
}
}
}
Thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
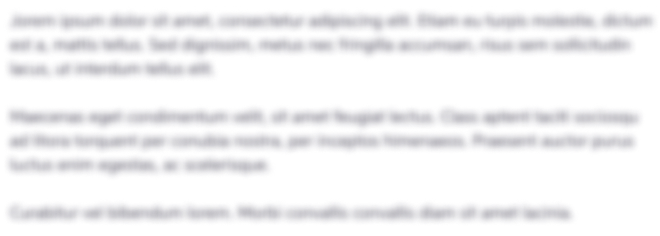
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started