Question
Please help with this problem in Python. Below is the starter code, I need help implementing the resize function. I have worked on the append
Please help with this problem in Python. Below is the starter code, I need help implementing the resize function. I have worked on the append method, I need help with methods.
Instructions:
Implement a DynamicArray class by completing the skeleton code provided in the file dynamic_array.py. The DynamicArray class will use a StaticArray object as its underlying data storage container, and will provide many methods similar to the functionality of Python lists. Once completed, your implementation will include the following methods: resize() append() insert_at_index() remove_at_index() slice() merge() map() filter() reduce() * Several class methods, like is_empty(), length(), get_at_index(), and set_at_index() have been pre-written for you. * The dynamic_array.py file also contains the find_mode() function, but this is a separate function outside the class that you will need to implement.
RESTRICTIONS: You are NOT allowed to use ANY built-in Python data structures and/or their methods in any of your solutions. This includes built-in Python lists, dictionaries, or anything else. You must solve this portion of the assignment by importing and using objects of the StaticArray class (prewritten for you), and using class methods to write your solution. You are also not allowed to directly access any variables of the StaticArray class (e.g. self._size or self._data). Access to StaticArray variables must only be done by using the StaticArray class methods.
#starter code
class DynamicArray: def __init__(self, start_array=None): """ Initialize new dynamic array DO NOT CHANGE THIS METHOD IN ANY WAY """ self._size = 0 self._capacity = 4 self._data = StaticArray(self._capacity) # populate dynamic array with initial values (if provided) # before using this feature, implement append() method if start_array is not None: for value in start_array: self.append(value) def __str__(self) -> str: """ Return content of dynamic array in human-readable form DO NOT CHANGE THIS METHOD IN ANY WAY """ out = "DYN_ARR Size/Cap: " out += str(self._size) + "/" + str(self._capacity) + ' [' out += ', '.join([str(self._data[_]) for _ in range(self._size)]) return out + ']' def __iter__(self): """ Create iterator for loop DO NOT CHANGE THIS METHOD IN ANY WAY """ self._index = 0 return self def __next__(self): """ Obtain next value and advance iterator DO NOT CHANGE THIS METHOD IN ANY WAY """ try: value = self[self._index] except DynamicArrayException: raise StopIteration self._index += 1 return value def get_at_index(self, index: int) -> object: """ Return value from given index position Invalid index raises DynamicArrayException DO NOT CHANGE THIS METHOD IN ANY WAY """ if index < 0 or index >= self._size: raise DynamicArrayException return self._data[index] def set_at_index(self, index: int, value: object) -> None: """ Store value at given index in the array Invalid index raises DynamicArrayException DO NOT CHANGE THIS METHOD IN ANY WAY """ if index < 0 or index >= self._size: raise DynamicArrayException self._data[index] = value def __getitem__(self, index) -> object: """ Same functionality as get_at_index() method above, but called using array[index] syntax DO NOT CHANGE THIS METHOD IN ANY WAY """ return self.get_at_index(index) def __setitem__(self, index, value) -> None: """ Same functionality as set_at_index() method above, but called using array[index] syntax DO NOT CHANGE THIS METHOD IN ANY WAY """ self.set_at_index(index, value) def is_empty(self) -> bool: """ Return True is array is empty / False otherwise DO NOT CHANGE THIS METHOD IN ANY WAY """ return self._size == 0 def length(self) -> int: """ Return number of elements stored in array DO NOT CHANGE THIS METHOD IN ANY WAY """ return self._size def get_capacity(self) -> int: """ Return the capacity of the array DO NOT CHANGE THIS METHOD IN ANY WAY """ return self._capacity def print_da_variables(self) -> None: """ Print information contained in the dynamic array. Used for testing purposes. DO NOT CHANGE THIS METHOD IN ANY WAY """ print(f"Length: {self._size}, Capacity: {self._capacity}, {self._data}")
#skeleton code
def map(self, map_func) -> "DynamicArray": """ TODO: Write this implementation """ pass def filter(self, filter_func) -> "DynamicArray": """ TODO: Write this implementation """ pass def reduce(self, reduce_func, initializer=None) -> object: """ TODO: Write this implementation """ pass def find_mode(arr: DynamicArray) -> (DynamicArray, int): """ TODO: Write this implementation """ pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
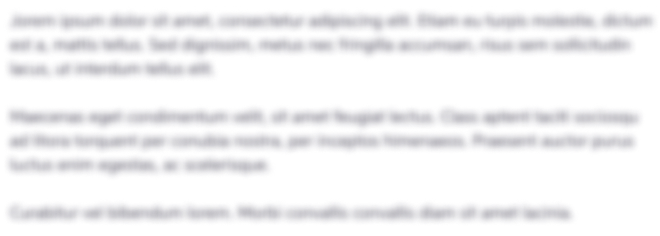
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started