Question
please help with this question and post it with comments of work to undersatnd the program Question 1: Booking System (100 points) For this question,
please help with this question and post it with comments of work to undersatnd the program
Question 1: Booking System (100 points) For this question, you will write several classes, and then create and use instances of those classes in order to simulate a hotel booking system. Note that in addition to the required methods below, you are free to add as many other private methods as you want (no additional public method is allowed).
(a) (20 points) Write a class Room. A Room has the following private attributes:
A String type
A double price
A boolean availability The Room class also contains the following public methods:
A constructor that takes as input the type of the room and uses it to initialize the attributes. Note that there are only 3 type of Rooms supported by the program: double, queen, and king. If the input is not one of these types, then the constructor should throw an IllegalArgumentEx- ception explaining that no room of such type can be created. The price of the room is based on its type as follows: $90 for a double, $110 for a queen, $150 for a king. The constructor should set the availability for a new room to be true.
getType which returns the type of the room.
getPrice which returns the price of the room.
getAvailability which returns the availability of the room.
changeAvailability which takes no input and sets the value stored in the availability attribute to be the opposite of the one currently there.
A findAvailableRoom method which takes as input an array of Rooms as well as a String indicating the room type. The method should return the first available room in the array of the indicated type. If no such room exists (either because all rooms of said type are occupied, or because no room of such type is in the array), the method returns null.
(b) (10 points) Write a class Reservation. A Reservation has the following private attributes:
A String name
A Room roomReserved The Reservation class also contains the following public methods:
A constructor that takes as input a Room and the name under which the reservation is made. The constructor must use its inputs to initialize the attributes.
getName which returns the name under which the reservation was made.
getRoom which returns the Room that has been reserved.
(c) (40 points) Write a class Hotel. This class has three private attributes:
A String name An array of Room An array of Reservation
It also has the following methods:
A public constructor that takes as input the name of the hotel, and an array of rooms. It initializes the Room array and copies the Room references from the input array to the Hotel array.
A private method addReservation that takes a Reservation as input and adds it to the array of Reservation of the hotel.
A private method removeReservation that takes as input a name and a type of room. If no reservation has been made under the given name for the given type of room, then the method should throw a NoSuchElementException with the appropriate message. If a matching reser- vation is found, then the method should remove such reservation from the array of reservations of the hotel, and make sure that the availability of the Room is modified accordingly. Note that you need to import java.util.NoSuchElementException in order to be able to use it.
A public method createReservation that takes as input a name and the type of the room that should be reserved. The method should use findAvailableRoom (from the Room class) to verify whether a room of the given type is available in the hotel. If not, the method should print a message informing the user that the hotel has no available rooms of the requested type. Otherwise, the method should: create a reservation for such room under the given name, change the availability of the room, and add the reservation to the array of reservations of the hotel using the addReservation method. In this case, the method should also print a message letting the user know that the reservation was successfully completed.
ApublicmethodcancelReservationthattakesasinputthenameunderwhichthereservation was made and the room type reserved. This method should use the removeReservation method to cancel the reservation. If no matching reservation was found, the method should inform the user that no reservation under such name has been made for the given type of room. Otherwise, the method should print a message letting the user know that the operation was successful. To receive full marks, a try/catch block should be used.
A public method printInvoice that takes as input a name and prints out a message letting the user know how much the given person owes the hotel based on all the reservations made under such name.
The toString method that returns a String containing the name of the hotel as well as how many available rooms of each type the hotel has.
(d) (30 points) In the provided class BookingSystem write a main method. Here, you will write a main method that creates an hotel where the name of the hotel is chosen by the user via a Scanner object. The program then creates an array of rooms at random and uses it, together with the hotel name, to create a new hotel.
In order to facilitate generating your Room array, we have provided methods to generate a random number of rooms, and to select the room types randomly from a provided array.
Then, the program should print on the screen a menu from which the user can chose one of the following options:
1. Make a reservation 2. Cancel a reservation 3. See an invoice 4. See the hotel info 5. Exit the booking system
Depending on the option chosen, you should use Scanner to obtain from the user the information needed (if any) to carry forward the operation selected. After each operation, the main menu should
be displayed again, unless the user had chosen to exit the booking system. In such case, the program should end. If the user enters a wrong option, then the main menu should also be displayed again. Note that you can assume that the user will always be entering an integer. Sample interaction and output is shown below.
. A pablic constructor that takes as input the name ofhe, and an array of rooms, It be displayed again, unless the user had chosen to exit the booking system In suh esse, the program sbould end. If the user enters a wrong option, then the main menu sbould also be displayed again Note that you cnn ssume that the user will always be entering an integer, Sample isteraction and output is shown below initialiaes the Roos array and copies the Room references from the input array to the Hotel sray A private method addReservation that takes a Reservation as input and adds it to the BErRy of Reservation of the hotel example: A privato method zeoveReservation that takes as input a name and a type of room. If no reservation hes been made under the given name foe the given type of room, then the method should throw a eSuchEl ecentExcoption with the appropriate mesage, If a matching reser- vation is found, then the method should remove such reservation from the array of reservations of the hotel, and mke sure that the svsilability of the Room is modified secoedingly, Note that you need to import java.util.s ion in order to be able to use it. * A public method createReservation that takes 88 i putname and the type of the room that should be rserved. The method should use findAvailabletoon (from the Roon class) to verify whether room of the given type is available in the hotel. If not, the method should print a message informing the ser that the hotel has no available rooms of the requested type Otherwise, the method should: creste a reservation for such room under the given name, change the availability of the room, and add the reservation to the array of reservations of the hotel using the addReservation method. In this case, the method should also print a messsge letting the user know that the reservation was successfully completed A publie method cancelReservation that takes as input the name under which the reservation was made and the rocm type reserved. This method should use the resoveeservation method to canoel the reservation. If no matching reservation was found, the method should inform the user that so reservation under such name has been mnde for the gives type of room. Otherwise, the method should print a message letting the user know that the operation was successful. To receive full marks, a try eatch block should be used A public method printlavoice that takes as input a name and prints out a message letting the user know how much the given person owes the hotel based on all the reservations msde under such namne. . The tostring method that returns a String eontaining the name of the hotel as well ss how many available rooms of esch type the hotel bas (4) (30 points) In the provided class BookingSysten write a main method. Heee, you will write a main metbod that crestes an hotel where the ame of the hotel is chosen by the user via a Scanner object. The program then crestes an array of rooms at randoam and uses it together with the hotel name, to create a pow hotel. Is order to facilitate generating your Room array, we have provided methods to generate a random number of rooms, and to select the room types randomly from a provided array Then, the program should print on the screen a memu from which the user can chose one of the following options: . Make a reservation 2. Canoel a reservation 3. See an invoice See the hotel info . Exit the booking system Depending on the option chosen, you should use Scanner to obtain from the user the information needed (if any) to carry forward the operation selected. After ench operation, the msin mena should Page 4 Page 5Step by Step Solution
There are 3 Steps involved in it
Step: 1
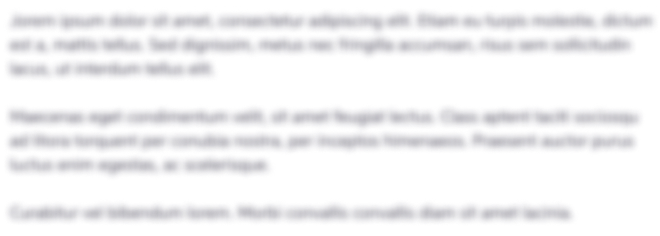
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started