Question
Please help write methods specifically (count, find, and slice) methods with tested examples below below Implement a Singly Linked List data structure by completing the
Please help write methods specifically (count, find, and slice) methods with tested examples below
below
Implement a Singly Linked List data structure by completing the skeleton code provided in the file sll.py. Once completed, your implementation will include the following methods: insert_front(), insert_back() insert_at_index(), remove_at_index() remove() count() find() slice() 2. We will test your implementation with different types of objects, not just integers. We guarantee that all such objects will have correct implementations of methods __eq__(), __lt__(), __gt__(), __ge__(), __le__(), and __str__(). 3. The number of objects stored in the list at any given time will be between 0 and 900 inclusive.
The variable in the LinkedList class (_head) is marked as private, so it may only be accessed/changed directly inside the class. Variables in the SLNode class are not marked as private. You are allowed to access/change their values directly wherever the SLNode class is used, and are not required to write getter or setter methods for them. 6. RESTRICTIONS: You are not allowed to use ANY built-in Python data structures or their methods. 7. This implementation must be done with the use of a front sentinel node.
skeleton code below
from SLNode import * class SLLException(Exception): """ Custom exception class to be used by Singly Linked List DO NOT CHANGE THIS CLASS IN ANY WAY """ pass class LinkedList: def __init__(self, start_list=None) -> None: """ Initialize new linked list DO NOT CHANGE THIS METHOD IN ANY WAY """ self._head = SLNode(None) # populate SLL with initial values (if provided) # before using this feature, implement insert_back() method if start_list is not None: for value in start_list: self.insert_back(value) def __str__(self) -> str: """ Return content of singly linked list in human-readable form DO NOT CHANGE THIS METHOD IN ANY WAY """ out = 'SLL [' node = self._head.next while node: out += str(node.value) if node.next: out += ' -> ' node = node.next out += ']' return out def length(self) -> int: """ Return the length of the linked list DO NOT CHANGE THIS METHOD IN ANY WAY """ length = 0 node = self._head.next while node: length += 1 node = node.next return length
def is_empty(self) -> bool: """ Return True is list is empty, False otherwise DO NOT CHANGE THIS METHOD IN ANY WAY """ return not self._head.next # ------------------------------------------------------------------ #
def insert_front(self, value: object) -> None: """ TODO: Write this implementation """ pass def insert_back(self, value: object) -> None: """ TODO: Write this implementation """ pass def insert_at_index(self, index: int, value: object) -> None: """ TODO: Write this implementation """ pass def remove_at_index(self, index: int) -> None: """ TODO: Write this implementation """ pass def remove(self, value: object) -> bool: """ TODO: Write this implementation """ pass def count(self, value: object) -> int: """ TODO: Write this implementation """ pass def find(self, value: object) -> bool: """ TODO: Write this implementation """ pass def slice(self, start_index: int, size: int) -> "LinkedList": """ TODO: Write this implementation """ pass
if __name__ == "__main__": print(" # insert_front example 1") test_case = ["A", "B", "C"] lst = LinkedList() for case in test_case: lst.insert_front(case) print(lst) print(" # insert_back example 1") test_case = ["C", "B", "A"] lst = LinkedList() for case in test_case: lst.insert_back(case) print(lst) print(" # insert_at_index example 1") lst = LinkedList() test_cases = [(0, "A"), (0, "B"), (1, "C"), (3, "D"), (-1, "E"), (5, "F")] for index, value in test_cases: print("Inserted", value, "at index", index, ": ", end="") try: lst.insert_at_index(index, value) print(lst) except Exception as e: print(type(e)) print(" # remove_at_index example 1") lst = LinkedList([1, 2, 3, 4, 5, 6]) print(f"Initial LinkedList : {lst}") for index in [0, 2, 0, 2, 2, -2]: print("Removed at index", index, ": ", end="") try: lst.remove_at_index(index) print(lst) except Exception as e: print(type(e)) print(" # remove example 1") lst = LinkedList([1, 2, 3, 1, 2, 3, 1, 2, 3]) print(f"Initial LinkedList, Length: {lst.length()} {lst}") for value in [7, 3, 3, 3, 3]: print(f"remove({value}): {lst.remove(value)}, Length: {lst.length()}" f" {lst}") print(" # remove example 2") lst = LinkedList([1, 2, 3, 1, 2, 3, 1, 2, 3]) print(f"Initial LinkedList, Length: {lst.length()} {lst}") for value in [1, 2, 3, 1, 2, 3, 3, 2, 1]: print(f"remove({value}): {lst.remove(value)}, Length: {lst.length()}" f" {lst}") print(" # count example 1") lst = LinkedList([1, 2, 3, 1, 2, 2]) print(lst, lst.count(1), lst.count(2), lst.count(3), lst.count(4)) print(" # find example 1") lst = LinkedList(["Waldo", "Clark Kent", "Homer", "Santa Claus"]) print(lst)
print(lst.find("Waldo")) print(lst.find("Superman")) print(lst.find("Santa Claus")) print(" # slice example 1") lst = LinkedList([1, 2, 3, 4, 5, 6, 7, 8, 9]) ll_slice = lst.slice(1, 3) print("Source:", lst) print("Start: 1 Size: 3 :", ll_slice) ll_slice.remove_at_index(0) print("Removed at index 0 :", ll_slice) print(" # slice example 2") lst = LinkedList([10, 11, 12, 13, 14, 15, 16]) print("Source:", lst) slices = [(0, 7), (-1, 7), (0, 8), (2, 3), (5, 0), (5, 3), (6, 1)] for index, size in slices: print("Start:", index, "Size:", size, end="") try: print(" :", lst.slice(index, size)) except: print(" : exception occurred.")
#test cases for problems only need count, find and slice
count
Example #1: lst = LinkedList([1, 2, 3, 1, 2, 2]) print(lst, lst.count(1), lst.count(2), lst.count(3), lst.count(4))
Output: SLL [1 -> 2 -> 3 -> 1 -> 2 -> 2] 2 3 1 0
find
Example #1: lst = LinkedList(["Waldo", "Clark Kent", "Homer", "Santa Claus"]) print(lst) print(lst.find("Waldo")) print(lst.find("Superman")) print(lst.find("Santa Claus"))
Output: SLL [Waldo -> Clark Kent -> Homer -> Santa Claus] True False True
slice
Example #1: lst = LinkedList([1, 2, 3, 4, 5, 6, 7, 8, 9]) ll_slice = lst.slice(1, 3) print("Source:", lst) print("Start: 1 Size: 3 :", ll_slice) ll_slice.remove_at_index(0) print("Removed at index 0 :", ll_slice) Output: Source: SLL [1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9] Start: 1 Size: 3 : SLL [2 -> 3 -> 4] Removed at index 0 : SLL [3 -> 4] Example #2: lst = LinkedList([10, 11, 12, 13, 14, 15, 16]) print("Source:", lst) slices = [(0, 7), (-1, 7), (0, 8), (2, 3), (5, 0), (5, 3), (6, 1)] for index, size in slices: print("Start:", index, "Size:", size, end="") try: print(" :", lst.slice(index, size)) except: print(" : exception occurred.") Output: Source: SLL [10 -> 11 -> 12 -> 13 -> 14 -> 15 -> 16] Start: 0 Size: 7 : SLL [10 -> 11 -> 12 -> 13 -> 14 -> 15 -> 16] Start: -1 Size: 7 : exception occurred. Start: 0 Size: 8 : exception occurred. Start: 2 Size: 3 : SLL [12 -> 13 -> 14] Start: 5 Size: 0 : SLL [] Start: 5 Size: 3 : exception occurred. Start: 6 Size: 1 : SLL [16]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
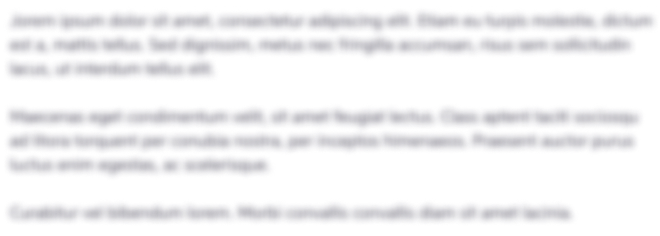
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started