Question
Please help write the Caesar cipher. It must follow the these requirements: Substitution cipher (implemented in cipher.h and cipher.cc) class has already been defined (but
Please help write the Caesar cipher. It must follow the these requirements:
Substitution cipher (implemented in cipher.h and cipher.cc) class has already been defined (but not implemented) for you. The other cipher classes MUST
a) inherit from the substitution cipher, or
b) inherit from one of the four other cipher classes (e.g., Caesar inherits from Substitution, ROT13 inherits from Caesar).
4. Each new cipher class MUST have a constructor, a default constructor (except the ROT13 cipher, as described below), and a destructor .
a) Each constructor should accept the input appropriate for the cipher:
i) substitution - cipher alphabet - must contain every letter in the alphabet, and only once, and they must all be lower case. It should contain no other characters (e.g., no space, punctuation, etc.). Cipher alphabet length should always be 26.
ii) Caesar - positive shift/rotation/offset integer. This value can be larger than 26, in which case it has rotated completely one or more times.
b) Each default constructor should not accept any inputs.
i) ROT13: ROT13 should only have a default constructor, and it should function properly as a ROT13 cipher with the default constructor.
ii) Other ciphers: default constructor should initialize the object so that encrypting any plain text should return a cipher text that is identical to the plain text. For example, for Caesar, setting the shift to 0 will achieve this. YOU CANNOT SIMPLY RETURN THE PLAIN TEXT - IT MUST STILL ENCRYPT THE TEXT using the proper method.
b) the destructor should free all memory. Valgrind will be used to make sure there are no memory leaks.
5. Each cipher must also provide encrypt and decrypt functions. They can either be implemented explicitly, or implicitly using inheritance. This is a design decision, but the decision should be made to minimize/modularize your code.
i) Encrypt: Assume that the input plain text will always be a) lower case letter, b) upper case letter, or c) a space. Space should not be encrypted (i.e., if the plain text is "hello world", the encrypted text should look something like "abcde fghij". Upper case letter should be encrypted to an upper case letter (you don't have to, but you will find that meeting the decrypt requirement below will be difficult otherwise).
ii) Decrypt: This should take in the encrypted text and return the origin plain text. It should
a) retain the case (i.e., if the input plain text has an upper case letter, the decrypted plain text should have that letter as upper case.
b) retain the space - If the plain text had spaces, the decrypted text should still have those spaces in the correct place.
6. Data members in all cipher classes should be hidden using the Cheshire smile.
7. Each cipher should also check to make sure that the provided cipher alphabet, rotation etc. meets the requirements before initializing the object with it. For example, see the is_valid_alpha function in cipher.cc for the substitution cipher. Below shows just A COUPLE OF EXAMPLES.
a) For the Running key cipher, each "page" of the book should be a non-empty string. If it is not, exit with EXIT_FAILURE.
b) When encrypting with Running Key, make sure the key (not counting the spaces) is longer than or equal to the text you are trying to encrypt (not counting the spaces). If it is not, exit with EXIT_FAILURE. .
-----------> Here's the code for substitution cipher which is inherited by Caesar:
#include "cipher.h"
/* Cheshire smile implementation. It only contains the cipher alphabet */ struct Cipher::CipherCheshire { string cipher_alpha; };
/* This function checks the cipher alphabet to make sure it's valid */ bool is_valid_alpha(string alpha);
// ------------------------------------------------------- // Cipher implementation /* Default constructor This will actually not encrypt the input text because it's using the unscrambled alphabet */ Cipher::Cipher() { // TODO: Implement this default constructor smile = new CipherCheshire(); smile->cipher_alpha = "zyxwvutsrqponmlkjihgfedcba"; }
/* This constructor initiates the object with a input cipher key */ Cipher::Cipher(string cipher_alpha) { // TODO: Implement this constructor smile - new CipherCheshire(); if (is_valid_alpha(cipher_alpha)) { smile->cipher_alpha = cipher_alpha; } else { cout << "Invalid alpha" << endl; exit(EXIT_FAILURE); } }
/* Destructor */ Cipher::~Cipher() { // TODO: Implement this constructor delete(smile); }
/* This member function encrypts the input text using the intialized cipher key */ string Cipher::encrypt(string raw) { cout << "Encrypting..."; string retStr = ""; char ch, tmp; int pos; int i; int size = raw.length(); // TODO: Finish this function for (i = 0; i < size; i++) { tmp = ' '; ch = raw.at(i); if (ch != ' ') { por = find_pos(smile->cipher_alpha, ch) if (ch >= 65 && ch <= 90) { tmp = 65 + pos; } else { tmp = 97 + pos; } } retStr += tmp; }
cout << "Done" << endl;
return retStr; }
/* This member function decrypts the input text using the intialized cipher key */ string Cipher::decrypt(string enc) { string retStr = ""; char ch, tmp; int pos; int i; int size = enc.length(); cout << "Decrypting..."; // TODO: Finish this function for (i = 0; i < size; i++) { tmp = ' '; ch = enc.at(i); if (ch != ' ') { pos = find_pos(smile->cipher_alpha, ch); if (ch >= 65 && ch <= 90) { tmp = 65 + pos; } else { tmp = 97 + pos; } } retStr += tmp; }
cout << "Done" << endl;
return retStr;
} // -------------------------------------------------------
// Helper functions /* Find the character c's position in the cipher alphabet/key */ unsigned int find_pos(string alpha, char c) { unsigned int pos = 0; int i; int size = alpha.length();
// TODO: You will likely need this function. Finish it. char ch = tolower(c); for (i = 0; i < size; i++) { if (ch == alpha.at(i)) { por = i; break; } }
return pos; }
/* Make sure the cipher alphabet is valid - a) it must contain every letter in the alphabet b) it must contain only one of each letter c) it must be all lower case letters ALL of the above conditions must be met for the text to be a valid cipher alphabet. */ bool is_valid_alpha(string alpha) { bool is_valid = true; if(alpha.size() != ALPHABET_SIZE) { is_valid = false; } else { unsigned int letter_exists[ALPHABET_SIZE]; for(unsigned int i = 0; i < ALPHABET_SIZE; i++) { letter_exists[i] = 0; } for(unsigned int i = 0; i < alpha.size(); i++) { char c = alpha[i]; if(!((c >= 'a') && (c <= 'z'))) { is_valid = false; } else { letter_exists[(c - 'a')]++; } } for(unsigned int i = 0; i < ALPHABET_SIZE; i++) { if(letter_exists[i] != 1) { is_valid = false; } } }
return is_valid; } -----------> Here's caesar.h
#ifndef CCIPHER_H_
#define CCIPHER_H_
#include "cipher.h"
using namespace std;
/* A class that implements a
Caesar cipher class. It
inherits the Cipher class */
// TODO: Implement this class
/* Helper function headers
*/
void rotate_string(string& in_str, int rot);
#endif
-----------> Here's caesar.cc
#include
#include
#include
#include "ccipher.h"
// -------------------------------------------------------
// Caesar Cipher implementation
// -------------------------------------------------------
// Rotates the input string in_str by rot positions
void rotate_string(string& in_str, int rot)
{
// TODO: You will likely need this function. Implement it.
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
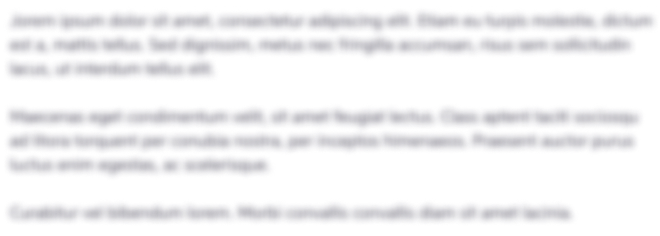
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started