please I need help
C++ Data Structures
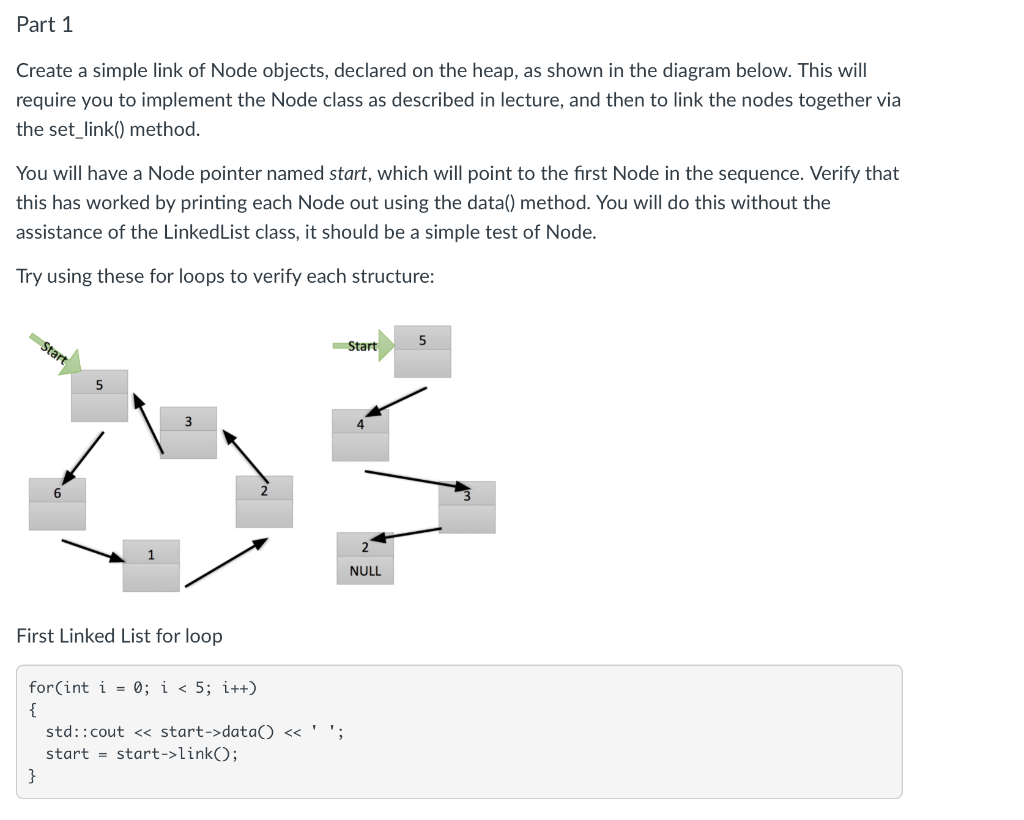
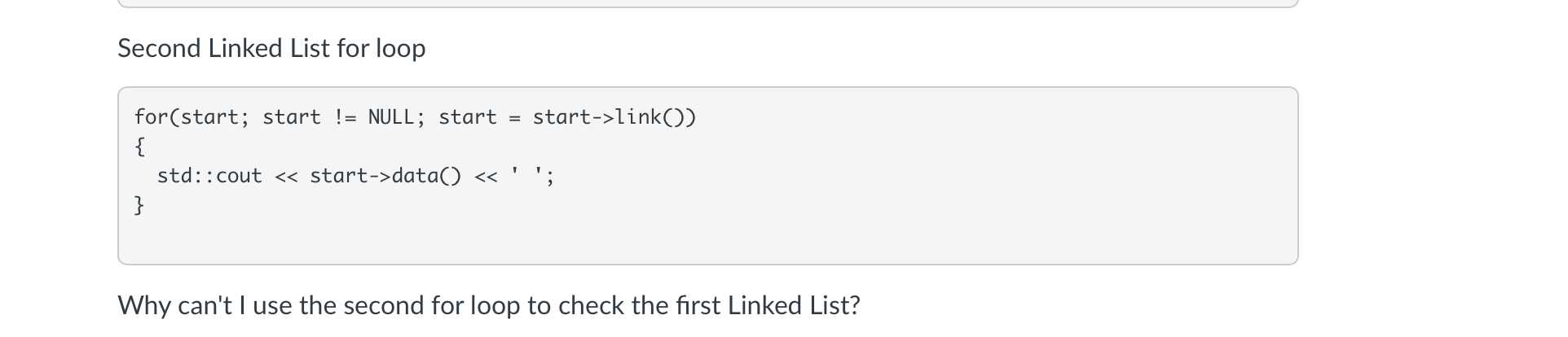
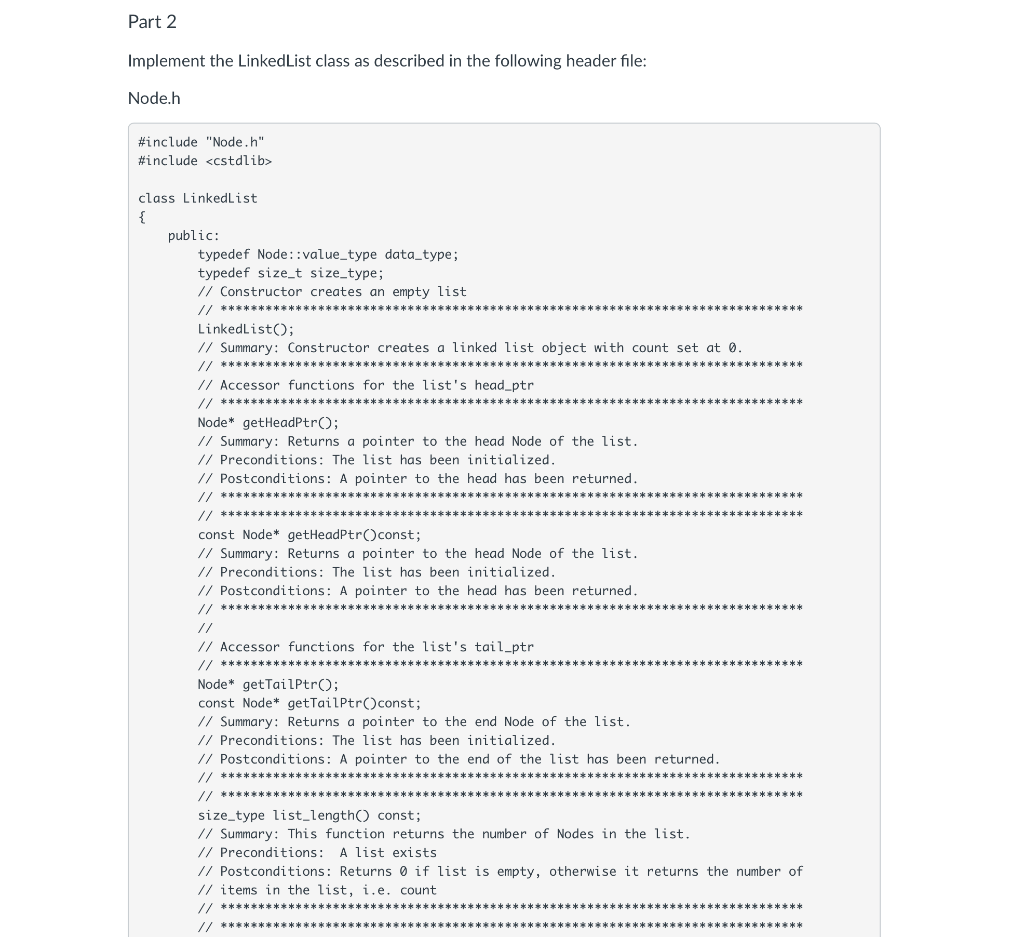
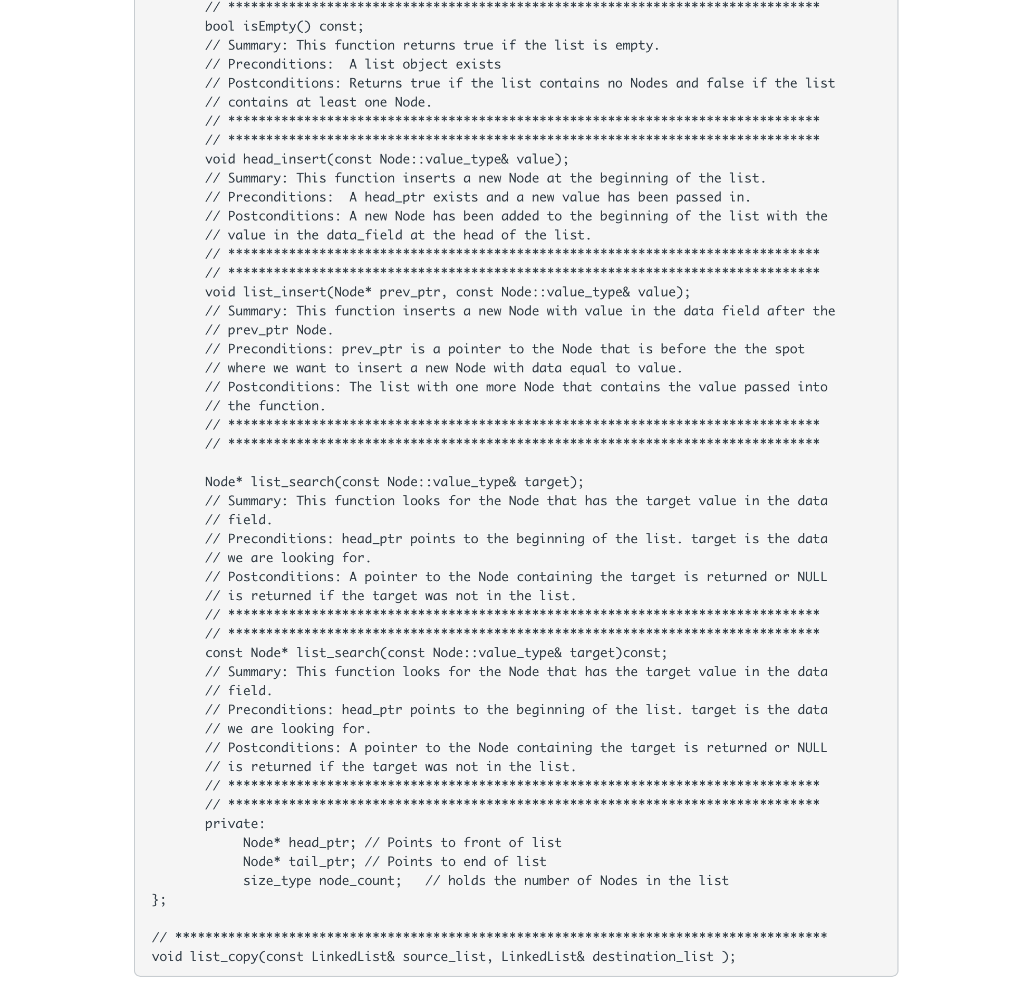
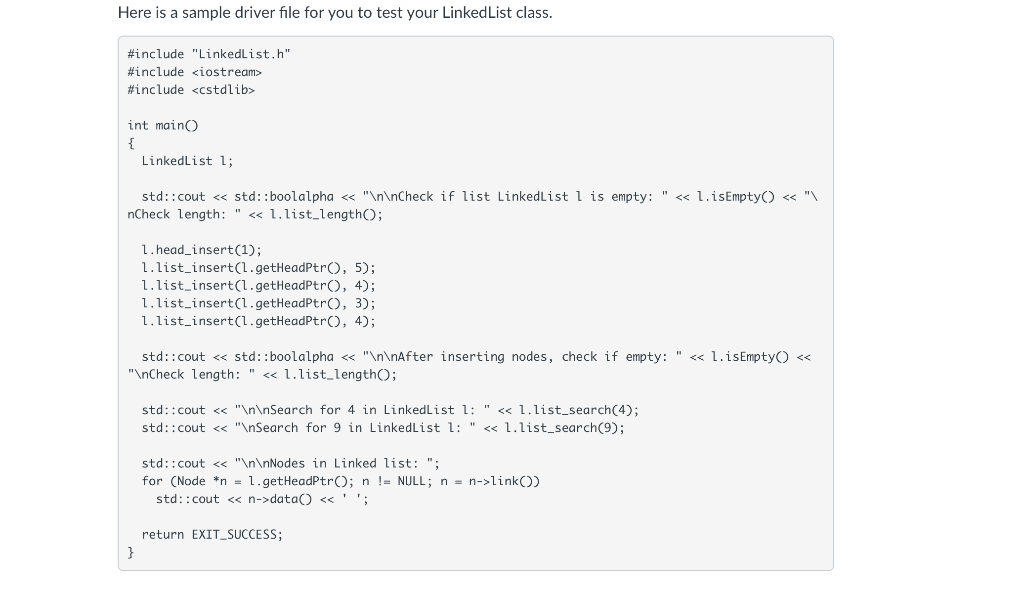
Part 1 Create a simple link of Node objects, declared on the heap, as shown in the diagram below. This will require you to implement the Node class as described in lecture, and then to link the nodes together via the set_link() method. You will have a Node pointer named start, which will point to the first Node in the sequence. Verify that this has worked by printing each Node out using the data() method. You will do this without the assistance of the LinkedList class, it should be a simple test of Node. Try using these for loops to verify each structure: Start 5 Start 3 1 NULL First Linked List for loop for(int i = 0; i data ' '; start = start->link; } Second Linked List for loop start->link()) for(start; start != NULL; start = { std::cout data() class LinkedList { public: typedef Node::value_type data_type; typedef size_t size_type; // Constructor creates an empty list // *** Linkedlist(); // Summary: Constructor creates a linked list object with count set at 0. // ****** // Accessor functions for the list's head_ptr // **** Node* getHeadPtrO; // Summary: Returns a pointer to the head Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the head has been returned. // *** const Node* getHeadPtr const; // Summary: Returns a pointer to the head Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the head has been returned. // // Accessor functions for the list's tail_ptr // *** Node* getTailPtrO; const Node* getTailPtr(const; // Summary: Returns a pointer to the end Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the end of the list has been returned. // *** // size_type list_length const; // Summary: This function returns the number of Nodes in the list. // Preconditions: A list exists // Postconditions: Returns if list is empty, otherwise it returns the number of // items in the list, i.e. count // *** ******************** bool isEmpty const; 1/ Summary: This function returns true if the list is empty. // Preconditions: A list object exists // Postconditions: Returns true if the list contains no Nodes and false if the list // contains at least one Node. // *** * *************** // **** void head_insert(const Node::value_type& value); // Summary: This function inserts a new Node at the beginning of the list. // Preconditions: A head_ptr exists and a new value has been passed in. // Postconditions: A new Node has been added to the beginning of the list with the // value in the data_field at the head of the list. // *** // *** void list_insert(Node* prev_ptr, const Node::value_type& value); 1/ Summary: This function inserts a new Node with value in the data field after the // prev_ptr Node. // Preconditions: prev_ptr is a pointer to the Node that is before the the spot // where we want to insert a new Node with data equal to value. // Postconditions: The list with one more Node that contains the value passed into // the function. ************* // *** Node* list_search(const Node::value_type& target); // Summary: This function looks for the Node that has the target value in the data // field. // Preconditions: head_ptr points to the beginning of the list. target is the data // we are looking for. // Postconditions: A pointer to the Node containing the target is returned or NULL // is returned if the target was not in the list. // *** const Node* list_search(const Node::value_type& target) const; // Summary: This function looks for the Node that has the target value in the data // field // Preconditions: head_ptr points to the beginning of the list. target is the data // we are looking for. // Postconditions: A pointer to the Node containing the target is returned or NULL // is returned if the target was not in the list. // *** *************** // private: Node* head_ptr; // Points to front of list Node* tail_ptr; // Points to end of list size_type node_count; // holds the number of Nodes in the list }; void list_copy(const LinkedList& source_list, LinkedList& destination_list); Here is a sample driver file for you to test your Linked List class. #include "Linkedlist.h" #include
#include int maino { LinkedList 1; std::cout link) std::cout data ''; return EXIT_SUCCESS; } Part 1 Create a simple link of Node objects, declared on the heap, as shown in the diagram below. This will require you to implement the Node class as described in lecture, and then to link the nodes together via the set_link() method. You will have a Node pointer named start, which will point to the first Node in the sequence. Verify that this has worked by printing each Node out using the data() method. You will do this without the assistance of the LinkedList class, it should be a simple test of Node. Try using these for loops to verify each structure: Start 5 Start 3 1 NULL First Linked List for loop for(int i = 0; i data ' '; start = start->link; } Second Linked List for loop start->link()) for(start; start != NULL; start = { std::cout data() class LinkedList { public: typedef Node::value_type data_type; typedef size_t size_type; // Constructor creates an empty list // *** Linkedlist(); // Summary: Constructor creates a linked list object with count set at 0. // ****** // Accessor functions for the list's head_ptr // **** Node* getHeadPtrO; // Summary: Returns a pointer to the head Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the head has been returned. // *** const Node* getHeadPtr const; // Summary: Returns a pointer to the head Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the head has been returned. // // Accessor functions for the list's tail_ptr // *** Node* getTailPtrO; const Node* getTailPtr(const; // Summary: Returns a pointer to the end Node of the list. // Preconditions: The list has been initialized. // Postconditions: A pointer to the end of the list has been returned. // *** // size_type list_length const; // Summary: This function returns the number of Nodes in the list. // Preconditions: A list exists // Postconditions: Returns if list is empty, otherwise it returns the number of // items in the list, i.e. count // *** ******************** bool isEmpty const; 1/ Summary: This function returns true if the list is empty. // Preconditions: A list object exists // Postconditions: Returns true if the list contains no Nodes and false if the list // contains at least one Node. // *** * *************** // **** void head_insert(const Node::value_type& value); // Summary: This function inserts a new Node at the beginning of the list. // Preconditions: A head_ptr exists and a new value has been passed in. // Postconditions: A new Node has been added to the beginning of the list with the // value in the data_field at the head of the list. // *** // *** void list_insert(Node* prev_ptr, const Node::value_type& value); 1/ Summary: This function inserts a new Node with value in the data field after the // prev_ptr Node. // Preconditions: prev_ptr is a pointer to the Node that is before the the spot // where we want to insert a new Node with data equal to value. // Postconditions: The list with one more Node that contains the value passed into // the function. ************* // *** Node* list_search(const Node::value_type& target); // Summary: This function looks for the Node that has the target value in the data // field. // Preconditions: head_ptr points to the beginning of the list. target is the data // we are looking for. // Postconditions: A pointer to the Node containing the target is returned or NULL // is returned if the target was not in the list. // *** const Node* list_search(const Node::value_type& target) const; // Summary: This function looks for the Node that has the target value in the data // field // Preconditions: head_ptr points to the beginning of the list. target is the data // we are looking for. // Postconditions: A pointer to the Node containing the target is returned or NULL // is returned if the target was not in the list. // *** *************** // private: Node* head_ptr; // Points to front of list Node* tail_ptr; // Points to end of list size_type node_count; // holds the number of Nodes in the list }; void list_copy(const LinkedList& source_list, LinkedList& destination_list); Here is a sample driver file for you to test your Linked List class. #include "Linkedlist.h" #include #include int maino { LinkedList 1; std::cout link) std::cout data ''; return EXIT_SUCCESS; }