Question
Please I need help with Workshop 10 https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS10 Look at the code provided in the Collection template module, study it and understand it. The Collection
Please I need help with Workshop 10
https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS10
Look at the code provided in the Collection template module, study it and understand it. The Collection class is a template that works like a dynamic array of objects and resizes itself as objects are added to it. The code snippet below demonstrates how Collection works:
Collection
Output:
There are 3 items in the Collection! 1.23 2.34 3.45
Supplied Modules:
- Collection
- ReadWrite
- Employee
- Student
- Car
Do not modify these modules! Look at the code and make sure you understand them.
Modules that you will modify (The searchNlist module and the main module)searchNlist module
Implement this module in 'searchNlist.h' header file.
- "check": to check a value in a compound type array against a given key is a specific index
- "search": to search through a compound type array (using the check function before) and insert the matches found into a Collection of the same compound type.
- "listArrayElements": to list elements of an array with a title
- "sizeCheck": to compare the sizes of two collections of the same compound type.
check function template
Create a function template called check to check a value in a compound type array against a given key in a specific index
- An array of templated objects; the same type as the Collection type. (template type 1)
- An integer variable that represents the index to use in the comparison
- A key templated value to search for, in the array of objects. (template type 2)
The check function is rather simple and will return true or false based on the following condition:
- if the element at index i of the array equals the key or not Use the "==" operator to check for a match between the objects and the key. This operator is overloaded in each testing class used.
search function template
Create a function template called search that accepts four arguments in any order you prefer:
- An array of templated objects; the same type as the Collection type. (template type 1)
- An integer represents the number of elements in the array of objects
- A key templated value to search for, in the array of objects. (template type 2)
- A reference to a Collection of templated objects (template type 1). The Collection is defined in Collection.h
The search function template returns a bool that is true only if at least one match to the key is found in the array of objects and false otherwise. The search function goes through all the elements of the array of objects and adds all the matches found to the Collection. You are required to use the check function created above for a match between the object at a specific location and the key
listArrayElements function template
Lists all the elements of an array.
Create a function template called listArrayElements that accepts three arguments in any order you prefer:
- A const char* for a Title to be printed as the Title of the list.
- A constant array of templated objects (template type)
- The number of the elements of the array.
This function first prints the Title and then goes to newline.
Then it will print the row number and then insert each element of the array into cout with a newline attached.
For example, if this function is called for the following array of integers.
int a[]{10,20,30,40};
using the Title value of "INTEGERS" and the number of elements as 4, the output would be like the following.
INTEGERS 1: 10 2: 20 3: 30 4: 40
sizeCheck function template
Create a function template called sizeCheck that accepts two arguments in any order you prefer:
- A reference to a Collection of templated objects representing collection 1. The Collection is defined in Collection.h
- A reference to a Collection of templated objects representing collection 2. The Collection is defined in Collection.h
This function simply compares the sizes of the two collections and return true if the size of the first collection is larger and false otherwise.
Template type requirements
Have a comment section for each function and explain what requirements each type of your templates must have to be able to work with your logic. Then also copy these comments to the part 2 reflection of the workshop.
The main module
In this workshop you are modifying the main module. Make sure to update the comments at the top to reflect your work on the module
Modify the main module and call the search function at the condition of the five if statements in the source code.
See the comments in main.cpp on where to use this function
Modify the main module and call the sizeCheck function at the condition of the specified if statement in the source code.
See the comments in main.cpp on where to use this function
Also, call the listArrayElement function four times before the first three if statements and at the end of the main() function to list the elements of the integer array a.
See the comments in main.cpp on where to use this function
// Workshop 10: // Version: 2.0 // Date: 2021-11-18 // Author: Fardad Soleimanloo // // Description: // This file tests the lab section of your workshop // Modify the main function as stated in the workshop description ///////////////////////////////////////////// #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
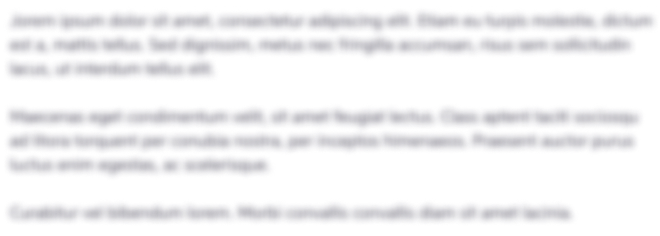
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started