Question
Please, I need this to be done in Java Eclipse, make sure you add comments and test it. implement the code for LoginResponse, loginRequest and
Please, I need this to be done in Java Eclipse, make sure you add comments and test it.
implement the code for LoginResponse, loginRequest and extendthe XmlUtility classes(write the new method to the XmlUtility class to generate the XML for that class.).
The LoginResponse XML must match the following example:
package week05.xml;
import week05.AtmException;
import week05.core.AtmObject;
import week05.util.LoginRequest;
import week05.util.LoginResponse;
import org.jdom2.Attribute;
import org.jdom2.Document;
import org.jdom2.Element;
/**
* Provides a set of utility methods to manage XML
*
* @author Dina
*
*/
public class XmlUtility
{
/**
* Serializes an AtmObject reference to an XML document
*
* @param obj
* AtmObject to serialize
* @return XML Document
*/
public static Document objectToXml(AtmObject obj) throws AtmException
{
Document dom = null;
if(obj instanceof LoginRequest)
{
dom = generateLoginRequestXml((LoginRequest)obj);
}
else if( obj instanceof LoginResponse)
{
dom = generateLoginResponseXml((LoginResponse)obj);
}
else
{
throw new AtmException("Unknown AtmObject");
}
return dom;
}
/**
* Takes an XML document and converts it to the appropriate ATM domain
* object.
*
* @param dom
* Document reference
* @return Appropriate ATM domain object
*/
public static AtmObject xmlToObject(Document dom)
throws AtmException
{
AtmObject atmObject = null;
// get the root and determine the type of object
Element root = dom.getRootElement();
String elementName = root.getName();
try
{
// extend this section to build the appropriate object
if(elementName.equals("login-request"))
{
// generate the LoginRequest object
atmObject = getLoginObject(root);
}
// extend this section to build the appropriate object
else if(elementName.equals("login-response"))
{
// generate the LoginResponse object
atmObject = getLoginResponseObject(root);
}
}
catch(NumberFormatException ex)
{
throw new AtmException(ex);
}
return atmObject;
}
private static AtmObject getLoginResponseObject(Element root)
throws NumberFormatException
{
throw new RuntimeException("Not Implemented");
}
private static AtmObject getLoginObject(Element root)
throws NumberFormatException
{
String pinString = root.getAttributeValue("pin");
String accountIdString = root.getAttributeValue("account-id");
int pin = Integer.parseInt(pinString);
int accountId = Integer.parseInt(accountIdString);
return new LoginRequest(pin, accountId);
}
private static Document generateLoginRequestXml(LoginRequest request)
{
Document dom = null;
// build the root element
Element root = new Element("login-request");
Attribute version = new Attribute("version", "1.0");
root.setAttribute(version);
String pinFmt = String.format("%d", request.getPin());
Attribute pin = new Attribute("pin", pinFmt);
root.setAttribute(pin);
String accountFmt = String.format("%d", request.getAccountId());
Attribute account = new Attribute("account-id", accountFmt);
root.setAttribute(account);
dom = new Document(root);
return dom;
}
private static Document generateLoginResponseXml(LoginResponse response)
{
throw new RuntimeException("Not Implemented");
}
}
/*****************TestLogIn**********************************/
package week05;
import static org.junit.Assert.*;
import org.junit.Test;
import java.io.IOException;
import java.io.StringReader;
import java.io.StringWriter;
import org.jdom2.Document;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import org.jdom2.output.XMLOutputter;
import test.AbstractJUnitBase;
import week05.core.AtmObject;
import week05.util.LoginRequest;
import week05.util.LoginResponse;
import week05.xml.XmlUtility;
public class TestLogIn extends AbstractJUnitBase
{
/**
* Default constructor
*/
public TestLogIn()
{
}
@Test
public void testGenerateObjectFromXmlLoginResponse()
{
try
{
LoginResponse refRequest = new LoginResponse(true, 100100L);
String testResponse = "
java.io.StringReader reader = new StringReader(testResponse);
org.jdom2.input.SAXBuilder builder = new SAXBuilder();
Document dom = builder.build(reader);
AtmObject request = XmlUtility.xmlToObject(dom);
if( !refRequest.equals(request))
{
String msg = "LoginRequest objects don't match";
trace(msg);
fail(msg);
}
}
catch(IOException ex)
{
String msg = " Failed: testGenerateObjectFromXmlLoginResponse " + ex.getMessage();
trace(msg);
fail(msg);
}
catch(JDOMException ex)
{
String msg = " Failed: testGenerateObjectFromXmlLoginResponse " + ex.getMessage();
trace(msg);
fail(msg);
}
catch(AtmException ex)
{
String msg = " Failed: testGenerateObjectFromXmlLoginResponse " + ex.getMessage();
trace(msg);
fail(msg);
}
}
@Test
public void testGenerateObjectFromXmlLoginRequest()
{
try
{
LoginRequest refRequest = new LoginRequest(1234, 100100L);
String testRequest = "
java.io.StringReader reader = new StringReader(testRequest);
org.jdom2.input.SAXBuilder builder = new SAXBuilder();
Document dom = builder.build(reader);
AtmObject request = XmlUtility.xmlToObject(dom);
if( !refRequest.equals(request))
{
String msg = "LoginRequest objects don't match";
trace(msg);
fail(msg);
}
}
catch(IOException ex)
{
String msg = "Failed: testGenerateObjectFromXmlLoginRequest " + ex.getMessage();
trace(msg);
fail(msg);
}
catch(JDOMException ex)
{
String msg = "Failed: testGenerateObjectFromXmlLoginRequest " + ex.getMessage();
trace(msg);
fail(msg);
}
catch(AtmException ex)
{
String msg = "Failed: testGenerateObjectFromXmlLoginRequest " + ex.getMessage();
trace(msg);
fail(msg);
}
}
@SuppressWarnings("unused")
@Test
public void tesGenerateXmlFromLoginRequest()
{
trace(" tesGenerateXmlFromLoginRequest");
boolean result = true;
try
{
AtmObject logRequest = new LoginRequest(1234, 100100L);
if(logRequest == null)
{
String msg = "Failed to create LoginRequest ";
trace(msg);
fail(msg);
}
Document dom = XmlUtility.objectToXml(logRequest);
String xml = dumpDocument(dom);
if(xml.equals(""))
{
String msg = "Failed to generate XML output ";
trace(msg);
fail(msg);
}
trace("Generated XML " + xml);
}
catch(Exception ex)
{
String msg = "Failed to generate XML output " + ex.getMessage();
trace(msg);
fail(msg);
}
}
private String dumpDocument(Document dom)
{
XMLOutputter outputter = new XMLOutputter();
java.io.StringWriter writer = new StringWriter();
try
{
outputter.output(dom, writer);
}
catch(IOException ex)
{
String msg = "Failed to dump XML output " + ex.getMessage();
trace(msg);
fail(msg);
ex.printStackTrace();
}
return writer.toString();
}
private boolean testLogRequestCreation()
{
trace(" testLogRequestCreation");
boolean result = true;
try
{
@SuppressWarnings("unused")
AtmObject logRequest = new LoginRequest(1234, 100100L);
}
catch(Exception ex)
{
String msg = "Failed to create " + ex.getMessage();
trace(msg);
fail(msg);
}
return result;
}
}
class data core: AtmObject Student implements this class Student extends this dass to generate XML for LoginResponse util: LoginResponse m_loggedln :Boolean m_sessionld :long + getLoggedlno Boolearn + getSessionld long + LoginResponse(Boolean, long) xml::XmlUtility + objectToXml(AtmObiect) String util: LoginRequest m_sccountld long m_pin :int + getAccountldo long + getPin0 :int + LoginRequest int, long) + setAccountld(long) :void + setPin(int) :void class data core: AtmObject Student implements this class Student extends this dass to generate XML for LoginResponse util: LoginResponse m_loggedln :Boolean m_sessionld :long + getLoggedlno Boolearn + getSessionld long + LoginResponse(Boolean, long) xml::XmlUtility + objectToXml(AtmObiect) String util: LoginRequest m_sccountld long m_pin :int + getAccountldo long + getPin0 :int + LoginRequest int, long) + setAccountld(long) :void + setPin(int) :voidStep by Step Solution
There are 3 Steps involved in it
Step: 1
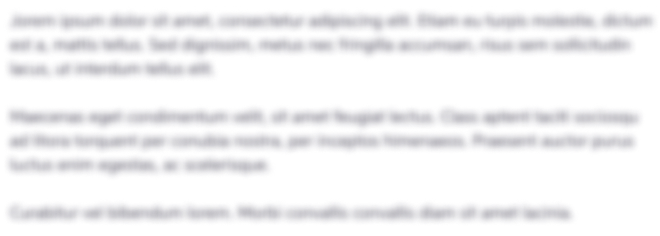
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started