Question
Please implement isvalidcc() and other functions which you may add to the c++ program. Credit card numbers follow certain patterns. A credit card number must
Please implement isvalidcc() and other functions which you may add to the c++ program.
Credit card numbers follow certain patterns. A credit card number must have between 13 and 16 digits. The starting numbers are: 4 for Visa cards, 5 for MasterCard cards, 37 for American Express cards, and 6 for Discover cards.
Example: Validating 4388576018402626
a) Double every second digit from right to left. If doubling of a digit results in a two-digit number, add the two digits to get a single digit number.
b) Now add all single-digit numbers from Step a:
4 + 4 + 8 + 2 + 3 + 1 + 7 + 8 = 37
c) Add all digits in the odd places from right to left in the card number:
6 + 6 + 0 + 8 + 0 + 7 + 8 + 3 = 38
d) Sum the results from Step b and Step c:
37 + 38 = 75
e) If the result from Step d is divisible by 10, the card number is valid; otherwise, it is invalid.
-DO NOT CHANGE THE MAIN METHOD
code-
bool isvalidcc(const string&);
int main()
{
//
// PLEASE DO NOT CHANGE main()
//
vector cardnumbers = {
"371449635398431", "4444444444444448", "4444424444444440", "4110144110144115",
"4114360123456785", "4061724061724061", "5500005555555559", "5115915115915118",
"5555555555555557", "6011016011016011", "372449635398431", "4444544444444448",
"4444434444444440", "4110145110144115", "4124360123456785", "4062724061724061",
"5501005555555559", "5125915115915118", "5556555555555557", "6011116011016011",
"372449635397431", "4444544444444448", "4444434444544440", "4110145110184115",
"4124360123457785", "4062724061724061", "5541005555555559", "5125115115915118",
"5556551555555557", "6011316011016011"
};
int i;
vector::iterator itr;
for (i = 1, itr = cardnumbers.begin(); itr != cardnumbers.end(); ++itr, i++) {
cout << setw(2) << i << " "
<< setw(17) << *itr
<< ((isvalidcc(*itr)) ? " is valid" : " is not valid") << endl;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
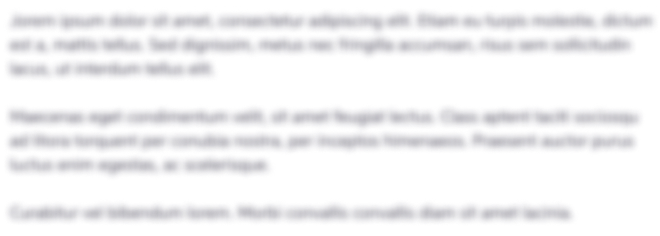
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started