Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please include an interface class or abstract class, an inheritance relationship, and one composition relationship. Please draw a UML diagram based on the following prompt.
Please include an interface class or abstract class, an inheritance relationship, and one composition relationship. Please draw a UML diagram based on the following prompt. Utilize MVC and State Pattern. Have added information on that below. I have attached a picture of how the UML diagram should look like.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
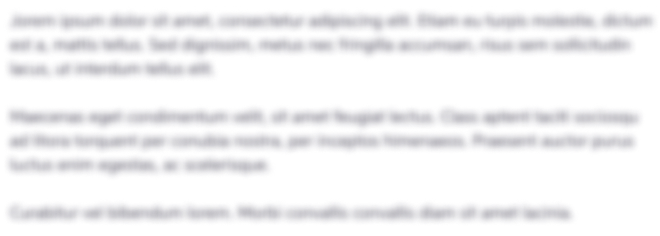
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started