Question
PLEASE INCLUDE COMMENTS AND JAVA DOCS!!!!! /** A quantity and price of a block of stocks. */ public class Block { private final int price;
PLEASE INCLUDE COMMENTS AND JAVA DOCS!!!!!
/** A quantity and price of a block of stocks. */ public class Block { private final int price; private int quantity;
/** Constructor. @param quantity the quantity of this block. @param price the price of this block. */ public Block(int quantity, int price) { this.price = price; this.quantity = quantity; }
public int getQuantity() { return quantity; } public int getPrice() { return price; } public void sell(int shares) { quantity -= shares; } }
.........
import java.util.Scanner; /** Runs a Stock Trading Simulation */ public class SimulationRunner { public static void main(String[] args) { StockSimulator sim = new StockSimulator();
Scanner in = new Scanner(System.in); boolean done = false; System.out.println("Stock Simulator Menu"); System.out.println("-----------------------------------------------"); System.out.println(" > buy stock-symbol quantity price"); System.out.println(" > sell stock-symbol quantity price"); System.out.println(" > quit to quit simulation."); System.out.println(); while (!done) { System.out.print(" > "); String action = in.next(); if (action.equals("buy")) { String symbol = in.next(); int quantity = in.nextInt(); int price = in.nextInt(); sim.buy(symbol, quantity, price); } else if (action.equals("sell")) { String symbol = in.next(); int quantity = in.nextInt(); int price = in.nextInt(); sim.sell(symbol, quantity, price); } else if (action.equals("quit")) { done = true; } } } }
.........
import java.util.LinkedList; import java.util.Queue; import java.util.Map; import java.util.TreeMap; /** Class for simulating trading a single stock at varying prices. */ public class StockSimulator { private Map
/** Constructor. */ public StockSimulator() { . . . }
/** Handle a user buying a given quantity of stock at a given price.
@param quantity how many to buy. @param price the price to buy. */ public void buy(String symbol, int quantity, int price) { . . .
}
/** Handle a user selling a given quantity of stock at a given price. @param symbol the stock to sell @param quantity how many to sell. @param price the price to sell. */ public void sell(String symbol, int quantity, int price) { . . .
} }
COP3337 Assignment 10 This is an additional assignment from the chapter on the Java Collections Framework. Suppose you buy 100 shares of a stock at $12 per share, then another 100 at $10 per share, then you sell 150 shares at $15. You have to pay taxes on the gain, but exactly what is the gain? In the United States, the FIFO rule holds: You first sell all shares of the first batch for a profit of $300, then 50 of the shares from the second batch, for a profit of $250, yielding a total profit of $550. Write a program that can make these calculations for arbitrary purchases and sales of shares in a portfolio. The user enters commands buy symbol quantity price, sell symbol quantity price (which causes the gain on that transaction to be displayed), and quit. Hint: Keep a MapStep by Step Solution
There are 3 Steps involved in it
Step: 1
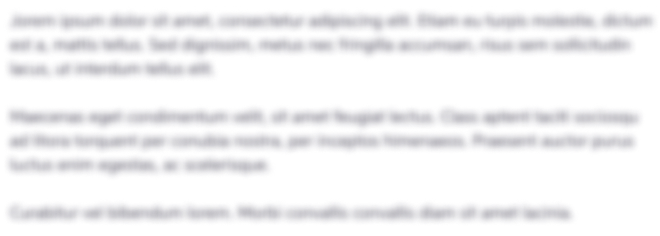
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started