Question
Please include java doc and football.txt file also thank you Different shells has been provided for you to fill in. You must start implementing the
Please include java doc and football.txt file also thank you
Different shells has been provided for you to fill in. You must start implementing the files in the following order: 1. Team class 2. TeamNode 3. shellLinkList 4. TeamLinkList 5. TeamLinkListClient The code for some of the methods has been provided for you. Problem: Code a class encapsulating a singly link list of football teams. A football team has the following attributes: 1. Teams nickname 2. Its number of wins 3. Its number of losses ( assumed there are no tie games) Implement the following methods: 1. Constructor 2. Insert 3. Delete 4. Peek 5. toString: returns a string containing the info about all the teams 6. mostWins: this method returns the team with the most wins 7. bestFive: this method returns the top five teams based on the winning percentage. If multiple teams have the same winning percentage, just return the first five teams 8. Search: this method search the list for a particular team and returns the information for the team. If the team is not in the list it should return null. 9. Count: this method returns the number of the teams in the list. 10. isEmpty: returns true if the list is not empty, and returns false otherwise. Write a driver class that does the following: 1. creates an object of the list 2. write a method called populate that populate the list by reading from a file that you need to create 3. Write a method that accepts the name of the team and the list of all the teams as its parameter, and then call the method delete to delete that team from the list. 4. Write a method called first team that accepts the list of the team as its parameter and returns the first team in the list. 5. Write a method call insert that accepts the list of the team as its parameter. This method asks for a team information, then adds the team to the list. 6. Read the following information for each team from a file and create the link list by calling appropriate methods.
/* The DataStructureException Class
Anderson, Franceschi
*/
public class DataStructureException extends Exception
{
/** constructor
* @param s error message
*/
public DataStructureException( String s )
{
super( s );
}
}
/* The ShellLinkedList class
Anderson, Franceschi
*/
public abstract class ShellLinkedList
{
protected TeamNode head;
protected int numberOfItems;
//set the head to null
//set the numberOfItems to 0
public ShellLinkedList( )
{
//set the head to null
//set the numberOfItems to 0
}
public int getNumberOfItems( )
{
return 0;
}
public boolean isEmpty( )
{
// return the number of the items in the list
return false;
}
public String toString( )
{
//returns a string representing all the items in the list
return "";
}
}
/* The Team Class
Anderson, Franceschi
*/
public class Team
{
private String nickname;
private int wins;
private int losses;
/** constructor
* @param newNickname starting value for nickname
* @param newWins starting balue for wins
* @param newLosses starting value for losses
*/
public Team( String newNickname, int newWins, int newLosses )
{
}
/** getNickname
* @return nickname
*/
public String getNickname( )
{
return "";
}
/** getWins
* @return wins
*/
public int getWins( )
{
return 0;
}
/** getLosses
* @return losses
*/
public int getLosses( )
{
return 0;
}
/** setNickname
* @param newNickname new value for nickname
*/
public void setNickname( String newNickname )
{
}
/** setWins
* @param newWins new value for wins
* newWins must be >= 0. otherwise value is unchanged
*/
public void setWins( int newWins )
{
}
/** setLosses
* @param newLosses new value for losses
* newLosses must be >= 0. otherwise value is unchanged
*/
public void setLosses( int newLosses )
{
}
/** equals
* @param o another Team object
* @return true if the instance variables in this object are equal to
the
* instance variables in t; false otherwise
*/
public boolean equals( Object o )
{
return false;
}
/** toString
* @return String representation of nickname, wins, and losses
*/
public String toString( )
{
return "";
}
/** winningPercentage
* @return wins / total games; 0 if no games have been played
*/
public double winningPercentage( )
{
return 0;
}
}
/* The TeamLinkedList class
Anderson, Franceschi
*/
import java.util.ArrayList;
public class TeamLinkedList extends ShellLinkedList
{
// head and numberOfItems are inherited instance variables
public TeamLinkedList( )
{
super( );
}
/** insert method
* @param t Team object to insert
*/
public void insert( Team t )
{
// create a TeamNode object and store it in tn
//call the method setNext on the object tn with the parameter head
//set the head to tn
//increment numberOfItems
}
/** delete method
* @param searchNickname nickname of Team to delete
* @return the Team deleted
*/
public Team delete( String searchNickname )
throws DataStructureException
{
//make a copy of the head node called current
//create an objcet of TeamNode called previous and set it to null
TeamNode current = head;
TeamNode previous = null;
//as longas current is not null and the name of the team is not the
name of the team of the current node
{
//set previous to current
//set the current to current.next
}
if ( current == null ) // not found
throw new DataStructureException( searchNickname
+ " not found: cannot be deleted" );
// else
{
// if current is equloa to the head nodes
//delete the head node
// delete head and adjust the new head
// else
//delete the node at the location and adjust the refrence
addresees
numberOfItems--;
return current.getTeam( );
}
}
/** orderTeams method
* @return an ArrayList of the teams ordered in descending order by
winning percentages
*/
public ArrayList
{
ArrayList
// Fill list with the linked list of teams
TeamNode current = head;
while ( current != null )
{
list.add( current.getTeam( ) );
current = current.getNext( );
}
ArrayList
// fill orderedList from list using a modified version of selection
sort
// sorting in descending order by winning percentages
double currentMaxWinPercentage = 0;
Team temp, tempMaxWP;
for (int i = 0; i
{
// find best team
tempMaxWP = ( Team ) list.get( 0 );
for ( int j = 1; j
{
temp = ( Team ) list.get( j );
if ( temp.winningPercentage( ) > tempMaxWP.winningPercentage( ) )
tempMaxWP = temp;
}
// add tempMaxWP to orderedList
orderedList.add( tempMaxWP );
// remove it from list
list.remove( tempMaxWP );
}
return orderedList;
}
/** fiveBestTeams method
* @return an ArrayList representing the five best teams based on
winning percentage
*/
public ArrayList
{
ArrayList
//create an orderd list of the teams
ArrayList
Team temp;
if ( ordered.size( )
{
for ( int i = 0; i
{
//get the item at i in the ordered list in the variable temp
//get the name of the team stored in temp
//get the wins of the team stored in temp
//get the losses of the team stored in temp
//cretae an object of Team
//add the object to the array list result
}
}
else
{
for ( int i = 0; i
{
//get the item at i in the ordered list in the variable temp
//get the name of the team stored in temp
//get the wins of the team stored in temp
//get the losses of the team stored in temp
//cretae an object of Team
//add the object to the array list result
}
}
return result;
}
/** maxWins method
* @return an int, the maximum number of wins by any team
*/
public int maxWins( )
{
int max = 0; // number of wins cannot be negative
//copy the head node in the variable called current
// while current is not null
{
//if the wins of the team stored in the current is gretaer than mxa
// set max to the wins of the team
// set the current to the next node
}
return max;
}
/** mostWins method
* @return an ArrayList of Strings storing all the nicknames of the
teams with the most wins
*/
public ArrayList
{
int maxWins = maxWins( );
ArrayList
//make a copy of the head node and call it current
// while current is not null
{
// if the wins of the current team is equal to maxWins
{
//add the current team nikname to the temp
}
//adjust current to the next node
}
return temp;
}
/** peek method
* @param searchNickname nickname of Team to search for
* @return a copy of the Team found
*/
public Team teek( String searchNickname )
throws DataStructureException
{
//make a copy of the head node and store it in the current
//while current is not null and the searchNickName is not equals to
the nukName of the current node
{
//set current to the nextNode
}
//if ( current == null ) // not found
// throw new DataStructureException( searchNickname
// + " not found: cannot be deleted" );
//else
{
//get the name of the current team,
// get the wins of the current team
//get the losses of the current team
//create a Team object
//return the object
}
return null;//this line must be removed
}
}
/* The TeamLinkedListClient class
Anderson, Franceschi
*/
import java.util.ArrayList;
public class TeamLinkedListClient
{
public static void main( String [] args )
{
// construct TeamLinkedList
//read the file and add each team to the list
TeamLinkedList teams = new TeamLinkedList( );
//output the number of the items in the list
//output all the teams on the screen
//call the method mostWins store the result in an array list called
winngest
//output the winnget array list (the array might have 0 or more
elements
//call the method fiveBestTeam store the result in an array list
called
//output the top teams from the array list
//output the winngest team
//output the list of the teams
//remove the first element from the last and add it to the end of the
list
//find the third team in the list
//ask the user for a team name and remove that team from the list
//call the method reverse
}
//his method receives the link list of the teams and prints the list
in the reverse order.
//you can use a stack or array or array list to print the link list in
the reverse order
public static void reverst(Object o)
{
}
}
/* The TeamNode class
Anderson, Franceschi
*/
public class TeamNode
{
private Team team;
private TeamNode next;
/** default constructor
* sets team and next to null
*/
public TeamNode( )
{
}
/** constructor
* @param t a Team object reference
* sets team to t, and next to null
*/
public TeamNode( Team t )
{
}
/** getTeam
* @return team
*/
public Team getTeam( )
{
return null;
}
/** getNext
* @return next
*/
public TeamNode getNext( )
{
return null;
}
/** setTeam
* @param t new value foe team
*/
public void setTeam( Team t )
{
}
/** setNext
* @param t TeamNode reference for next
*/
public void setNext( TeamNode t )
{
}
}
the output
Step by Step Solution
There are 3 Steps involved in it
Step: 1
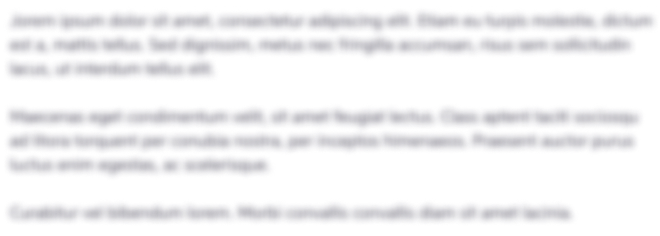
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started