Question
Please make it so that this code reads a file instead of being hard coded. The array is currently hard coded but needs to read
Please make it so that this code reads a file instead of being hard coded. The array is currently hard coded but needs to read any file, not just 1.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class JaggedGridReader {
private char[][] grid; // 2D array of characters to store the grid read from file
private String fileName; // name of the file to be read
// constructor
public JaggedGridReader(String fileName) {
this.fileName = fileName;
readFile(); // call method to read the file and store data in grid
}
// private method to read the file and store data in grid
private void readFile() {
try {
// create a Scanner object to read the file
Scanner scanner = new Scanner(new File(fileName));
int numRows = 0;
// loop through each line of the file to get the number of rows
while (scanner.hasNextLine()) {
numRows++;
scanner.nextLine();
}
scanner.close(); // close the scanner
scanner = new Scanner(new File(fileName));
// initialize the grid with the number of rows
grid = new char[numRows][];
for (int i = 0; i < numRows; i++) {
String line = scanner.nextLine();
grid[i] = line.toCharArray(); // convert each line to character array and store in grid
}
scanner.close(); // close the scanner
} catch (FileNotFoundException e) {
grid = null; // set grid to null if file not found
}
}
// override toString method to return grid as string
public String toString() {
StringBuilder builder = new StringBuilder();
// loop through each row and each character of the row
for (int i = 0; i < grid.length; i++) {
for (int j = 0; j < grid[i].length; j++) {
builder.append(grid[i][j]); // append each character to builder
}
builder.append(" "); // add new line after each row
}
return builder.toString(); // return the string representation of grid
}
// method to get a copy of the grid
public char[][] getCopy() {
if (grid == null) {
return null; // return null if grid is null
}
char[][] copy = new char[grid.length][];
for (int i = 0; i < grid.length; i++) {
copy[i] = grid[i].clone();
}
return copy;
}
public String getFileName() {
return fileName;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
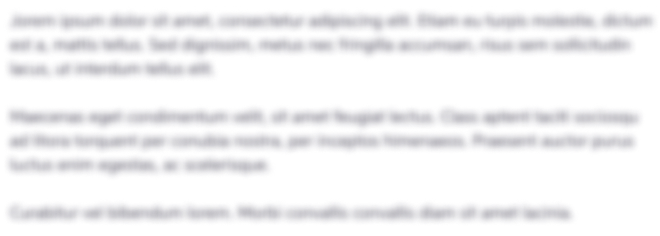
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started