Question
Please modify the accounts payable application to include the complete functionality of the payroll application. The application should still process two invoice objects, but now
Please modify the accounts payable application to include the complete functionality of the payroll application. The application should still process two invoice objects, but now should process one object of each of the four Employee subclasses (Figs. 10.510.8). If the object currently being processed is aBasePlusCommissionEmployee, the application should increase the BasePlusCommissionEmployees base salary by 10%.Finally, the application should output the payment amount for each object.Complete the following steps to create the new application: a) Modify classes HourlyEmployee and CommissionEmployee to place them in the Payable hierarchy as subclasses of the version of Employee that implements Payable. [Hint: You should introduce a getPaymentAmount method in each subclass so that the class satisfies its inherited contract with interface Payable. The getPaymentAmount can call earning to calculate the payable salary. b) Modify class BasePlusCommissionEmployee such that it extends the version of class CommissionEmployee created in Part a. c) Modify PayableInterfaceTest to polymorphically process two Invoices, one SalariedEmployee, one HourlyEmployee, oneCommissionEmployee and one BasePlusCommissionEmployee. First output a string representation of each Payable object. Next, if an object is a BasePlusCommissionEmployee, increase its base salary by 10%. Finally, output the payment amount for each Payable object. (JAVA LANGUAGE)
Invoice.java
// Problem 2: Invoice.java // Invoice class implements Payable.
public class Invoice implements Payable { private String partNumber; private String partDescription; private int quantity; private double pricePerItem;
// four-argument constructor public Invoice( String part, String description, int count, double price ) { partNumber = part; partDescription = description; setQuantity( count ); // validate and store quantity setPricePerItem( price ); // validate and store price per item } // end four-argument Invoice constructor
// set part number public void setPartNumber( String part ) { partNumber = part; } // end method setPartNumber
// get part number public String getPartNumber() { return partNumber; } // end method getPartNumber
// set description public void setPartDescription( String description ) { partDescription = description; } // end method setPartDescription
// get description public String getPartDescription() { return partDescription; } // end method getPartDescription
// set quantity public void setQuantity( int count ) { quantity = ( count < 0 ) ? 0 : count; // quantity cannot be negative } // end method setQuantity
// get quantity public int getQuantity() { return quantity; } // end method getQuantity
// set price per item public void setPricePerItem( double price ) { pricePerItem = ( price < 0.0 ) ? 0.0 : price; // validate price } // end method setPricePerItem
// get price per item public double getPricePerItem() { return pricePerItem; } // end method getPricePerItem
// return String representation of Invoice object public String toString() { return String.format( "%s: %s: %s (%s) %s: %d %s: $%,.2f", "invoice", "part number", getPartNumber(), getPartDescription(), "quantity", getQuantity(), "price per item", getPricePerItem() ); } // end method toString
// method required to carry out contract with interface Payable public double getPaymentAmount() { return getQuantity() * getPricePerItem(); // calculate total cost } // end method getPaymentAmount } // end class Invoice
Employee.java
public abstract class Employee implements Payable { private String firstName; private String lastName; private String socialSecurityNumber; public Employee(String first, String last, String ssn) { firstName = first; lastName = last; socialSecurityNumber = ssn; } public void setFirstName(String first) { firstName = first; } public String getFirstName() { return firstName; } public void setLastName(String last) { lastName = last; } public String getLastName() { return lastName; } public void setSocialSecurityNumber(String ssn) { socialSecurityNumber = ssn; } public String getSocialSecurityNumber() { return socialSecurityNumber; } public String toString() { return String.format( "%s %s social security number: %s", getFirstName(), getLastName(), getSocialSecurityNumber() ); } public abstract double earnings(); }
Payable.java
// Problem 2: Payable.java // Payable interface declaration.
public interface Payable { double getPaymentAmount(); // calculate payment; no implementation } // end interface Payable
PayableInterfaceTest.java
import java.util.*; public class PayableInterfaceTest { public static void main(String args[]) { Payable payableObjects[] = new Payable[7]; payableObjects[0] = new Invoice("01234", "seat", 2, 375.00); payableObjects[1] = new Invoice("56789", "tire", 4, 79.95); payableObjects[2] = new SalariedEmployee( "John", "Smith", "111-11-1111", 800.00 ); payableObjects[3] = new HourlyEmployee("Karen", "Price", "222-22-2222", 16.75, 40); payableObjects[4] = new CommissionEmployee("Sue", "Jones", "333-33-3333", 10000, .06); payableObjects[5] = new BasePlusCommissionEmployee("Bob", "Lewis", "444-44-4444", 5000, .04, 300); payableObjects[6] = new Pieceworker("Rick", "Bridges", "555-55-5555", 2.25, 400); System.out.println(" Invoices and Employees processed polymorphically:"); for (Payable currentPayable: payableObjects) { System.out.printf("%s ", currentPayable.toString()); if (currentPayable instanceof BasePlusCommissionEmployee) { BasePlusCommissionEmployee employee = (BasePlusCommissionEmployee) currentPayable; employee.setBaseSalary(1.10 * employee.getBaseSalary()); System.out.printf("new base salary with 10%% increase is: $%,.2f ", employee.getBaseSalary() ); } System.out.printf("%s: $%,.2f ", "payment due", currentPayable.getPaymentAmount()); // System.out.printf("earned $%,.2f ", currentEmployee.earnings()); } } }
Desired Output:
Invoices and Employees processed polymorphically:invoice:part number: 01234 (seat)quantity: 2price per item: $375.00payment due: $750.00invoice:part number: 56789 (tire)quantity: 4price per item: $79.95payment due: $319.80salaried employee: John Smithsocial security number: 111-11-1111weekly salary: $800.00payment due: $800.00hourly employee: Karen Pricesocial security number: 222-22-2222hourly wage: $16.75; hours worked: 40.00payment due: $670.00commission employee: Sue Jonessocial security number: 333-33-3333gross sales: $10,000.00; commission rate: 0.06payment due: $600.00base-salaried commission employee: Bob Lewissocial security number: 444-44-4444gross sales: $5,000.00; commission rate: 0.04; base salary:$300.00new base salary with 10% increase is: $330.00 payment due: $530.00
*Last time I posted this question someone just copied the question and put it in the answer. Please do not do this!*
Step by Step Solution
There are 3 Steps involved in it
Step: 1
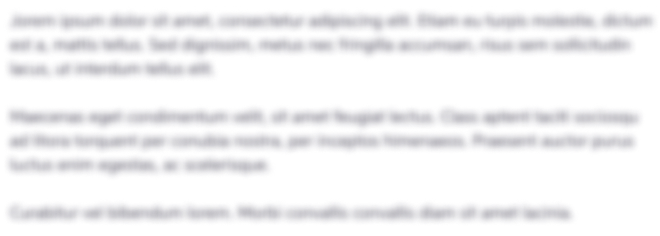
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started