Question
Please note!! This is only one question, I explained the question in the detailed formated with instructions and example, you only have to edit the
Please note!! This is only one question, I explained the question in the detailed formated with instructions and example, you only have to edit the given code to get the similar output in the example. Please answer it in step by step format. Thank you!!
In the quickselect/tests/ directory you have eight given input test cases. In each test case file the format is as follows:
The first line is N, the number of integers in the list.
The second line is k, where you are asked to report the k-th smallest number in the list. For example, k=0 would mean you should return the smallest number. k=N-1 would mean you should return the largest number.
The third line is blank.
All subsequent lines contain the unordered integers in the list. Numbers may repeat.
An example input file would look like:
8 6 10 6 5 7 5 0 10 12
Which means the list of 8 numbers is [10,6,5,7,5,0,10,12]; if sorted the list is [0,5,5,6,7,10,10,12], the 6th number (where indexing starts from 0) into this ordered list is 10.
You should write a C program that takes as a command line input the path to the test case file:
./quickselect tests/test0.txt
Expected output format:
In the answers/ directory you have the corresponding expected answers. You should print to the command line the k-th smallest number in the list. For example, the expected output for the test case above is:
10
Below is the given C program to edit and the get the output in the example above:
#include
#include
#include
// A translation from pseudocode to C code
// https://en.wikipedia.org/wiki/Quicksort
// Hoare's partition scheme
// Divides array into two partitions
int partition ( int size, int array[size] ) {
// Pivot value
int pivot = array[ (size-1)/2 ]; // The value in the middle of the array
// Left index
int left = -1;
// Right index
int right = size;
while (true) {
// Move the left index to the right at least once and while the element at
// the left index is less than the pivot
do left++; while (array[left] < pivot);
// Move the right index to the left at least once and while the element at
// the right index is greater than the pivot
do right--; while (pivot < array[right]);
// If the indices crossed, return
if ( right<=left ) return right;
// Swap the elements at the left and right indices
int temp = array[left];
array[left] = array[right];
array[right] = temp;
}
}
/**/
int main(int argc, char* argv[])
{
FILE* inputFile = fopen(argv[1], "r");
if (!inputFile) {
perror("fopen failed");
return EXIT_FAILURE;
}
char buf[256];
char* len_string = fgets(buf, 256, inputFile);
int len = atoi(len_string);
char* kth_string = fgets(buf, 256, inputFile);
int kth = atoi(kth_string);
fgets(buf, 256, inputFile);
int* array = calloc( len, sizeof(int) );
for (int i=0; i char* int_string = fgets(buf, 256, inputFile); array[i] = atoi(int_string); } printf("%d", quickselect ( len, array, kth )); free(array); return EXIT_SUCCESS; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
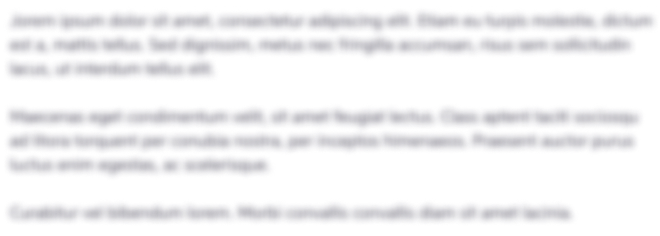
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started