Please
Only allowed to import java.util.Scanner.
Not allowed to use System.exit() You are allowed to use any Scanner and String methods. Any other classes not specified are NOT allowed.
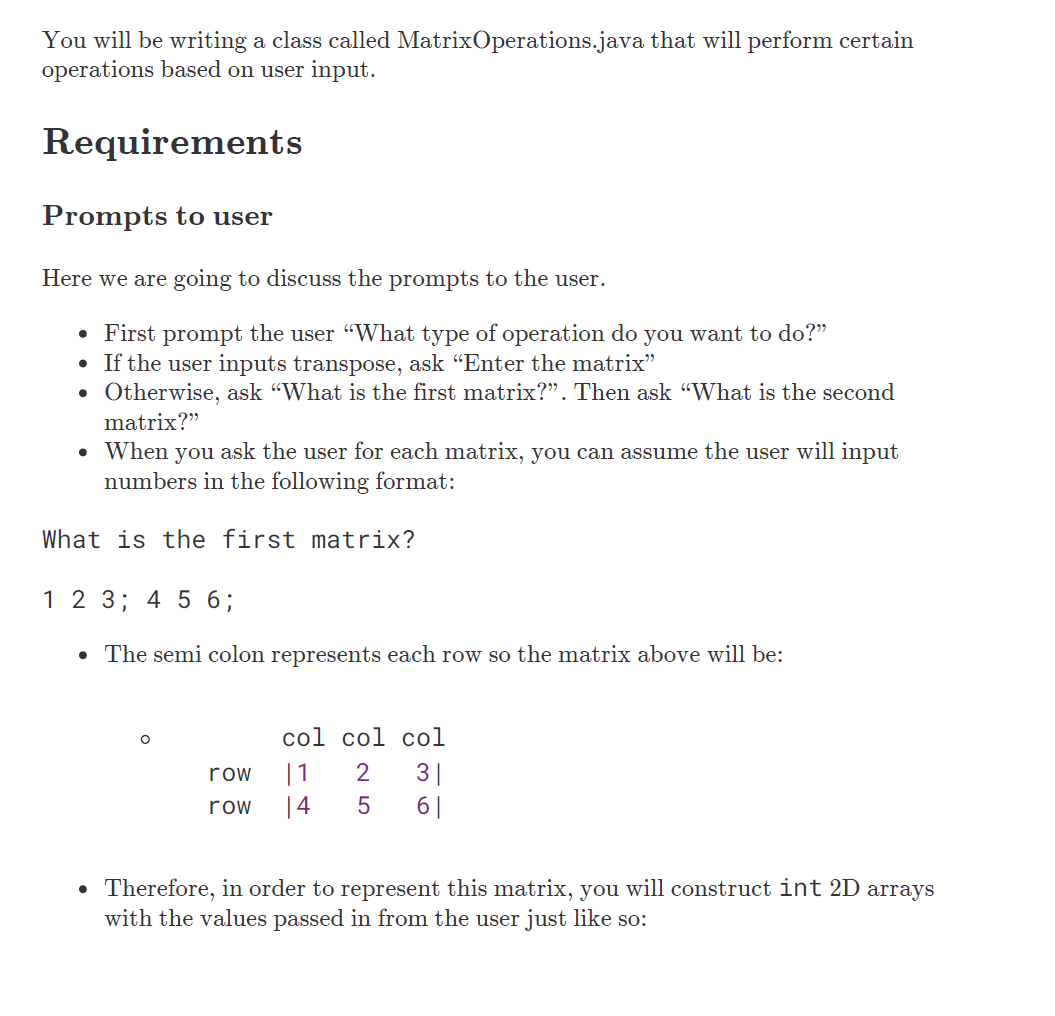
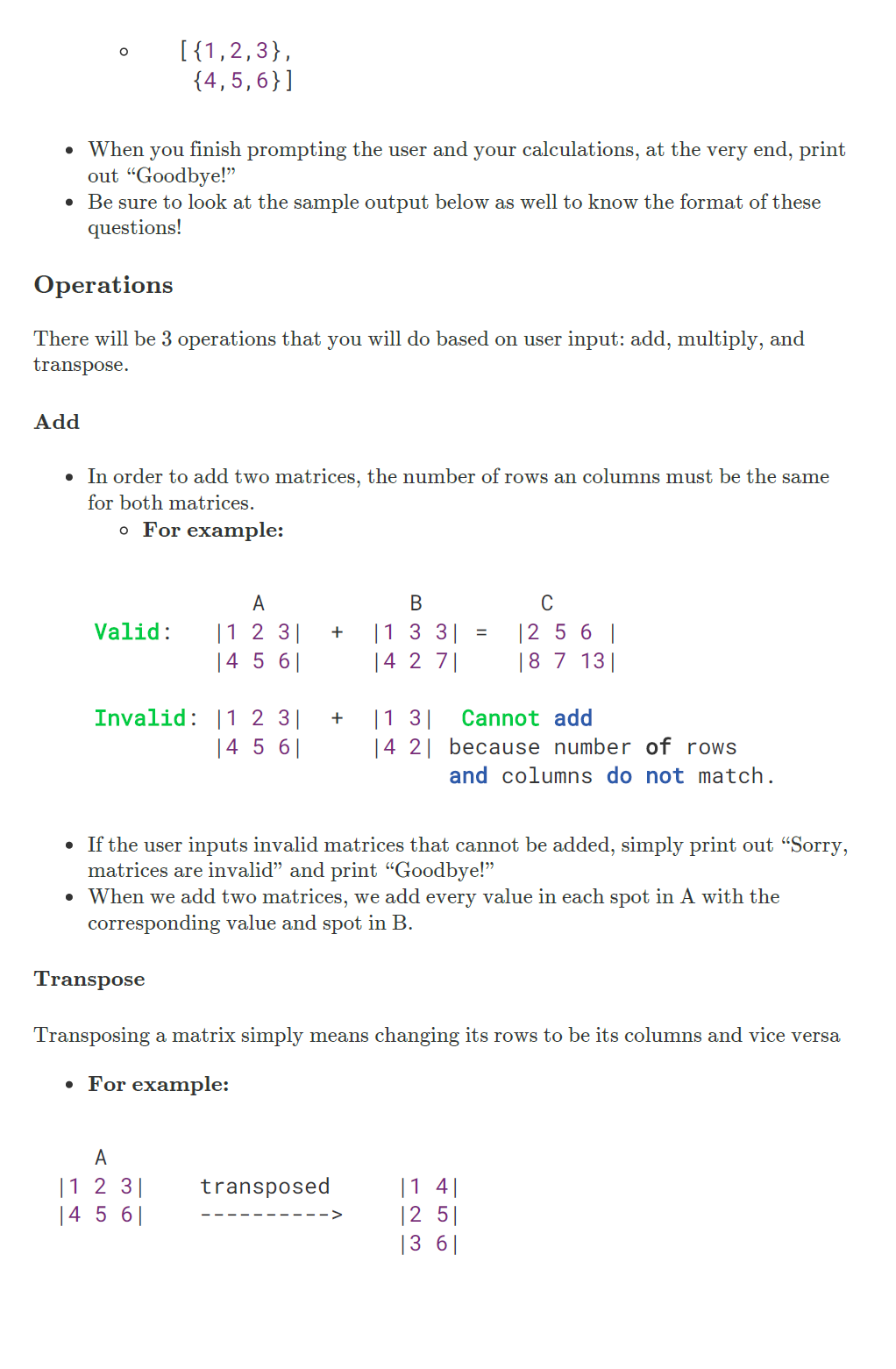
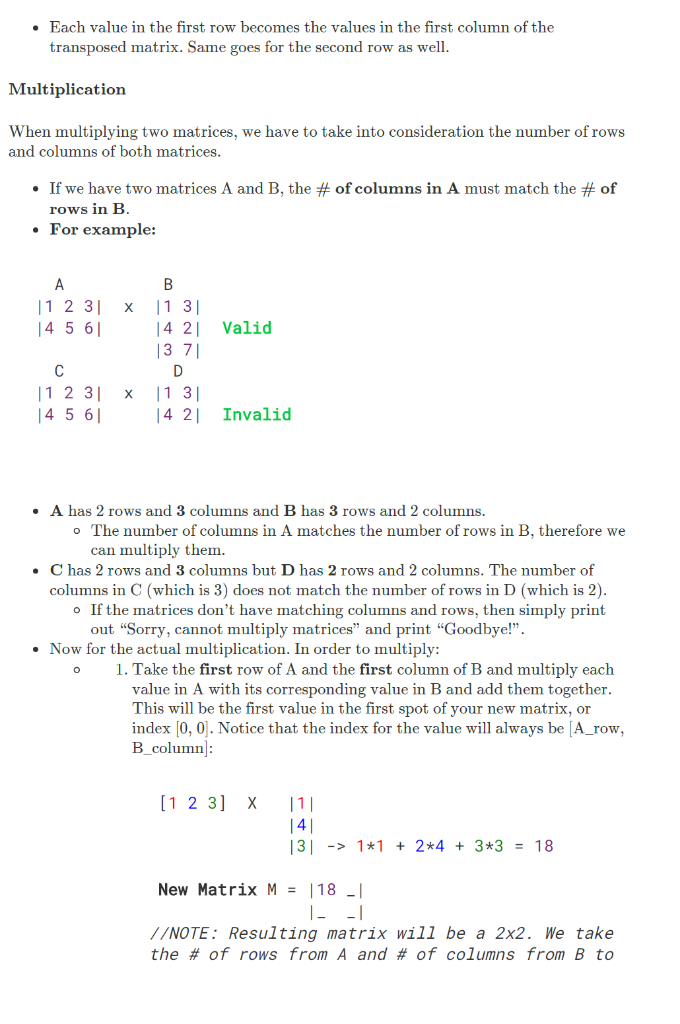
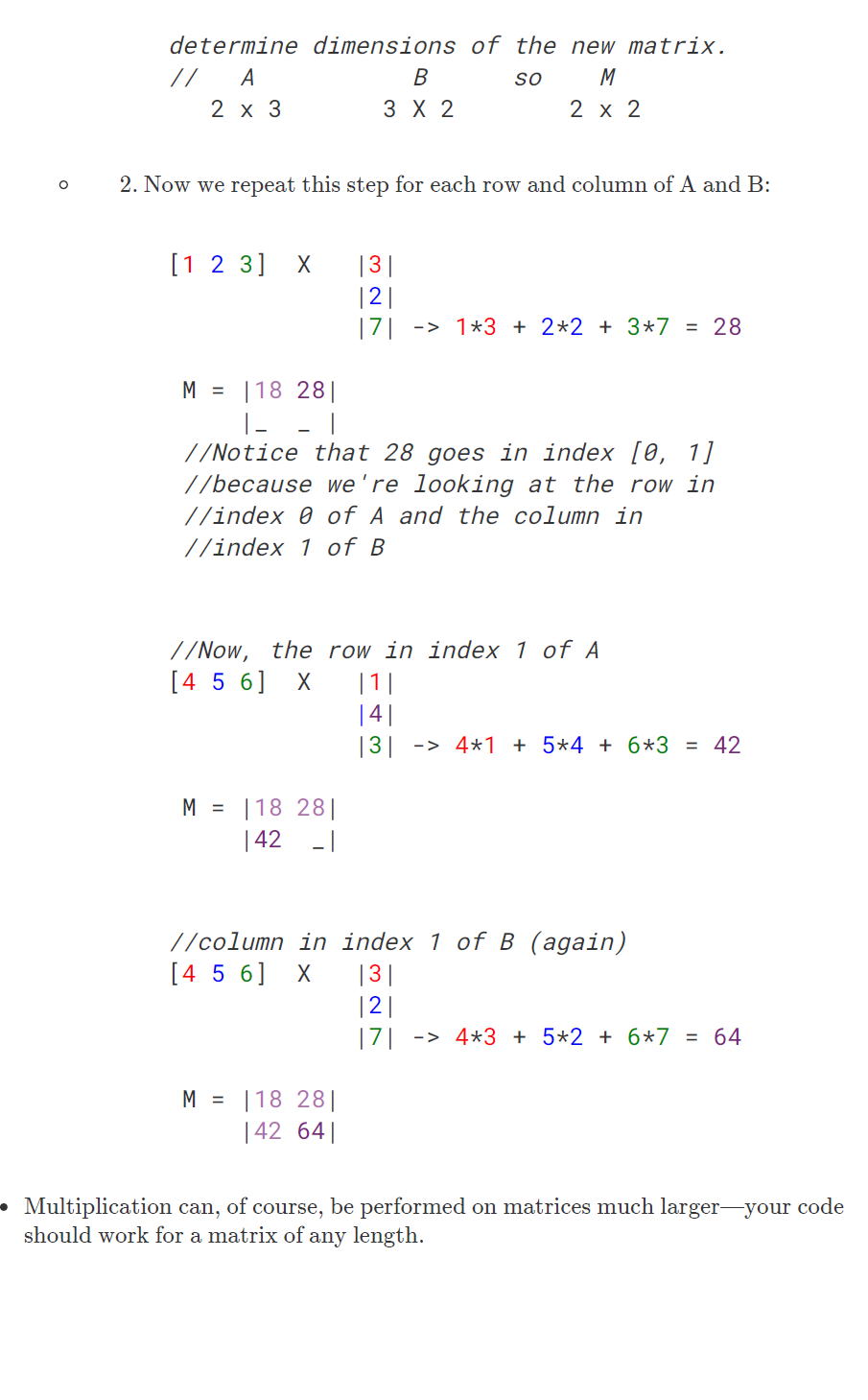
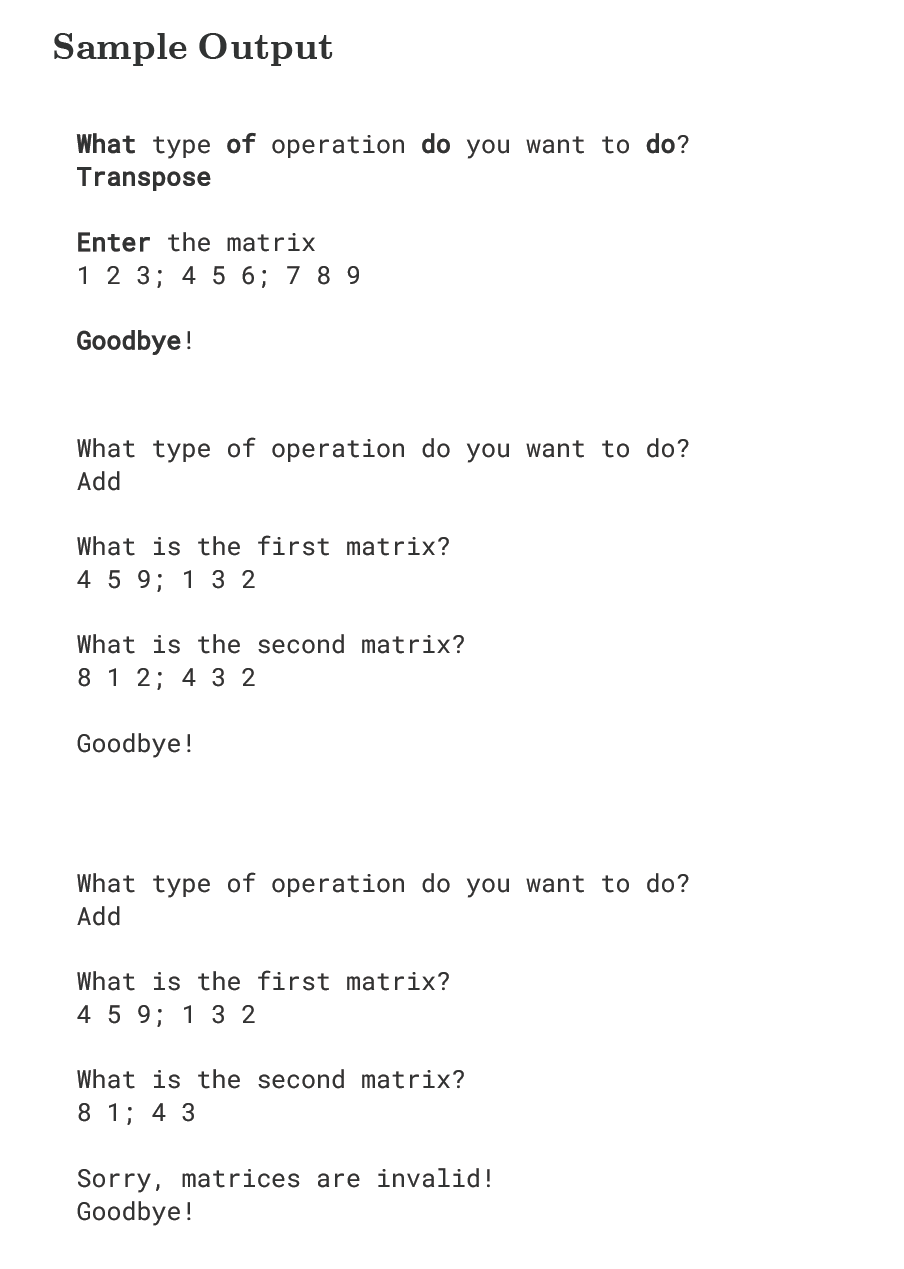
You will be writing a class called Matrix Operations.java that will perform certain operations based on user input. Requirements Prompts to user Here we are going to discuss the prompts to the user. First prompt the user What type of operation do you want to do? If the user inputs transpose, ask "Enter the matrix" Otherwise, ask "What is the first matrix?. Then ask What is the second matrix? When you ask the user for each matrix, you can assume the user will input numbers in the following format: What is the first matrix? 1 2 3; 4 5 6; The semi colon represents each row so the matrix above will be: row row col col col 11 2 3|| 14 5 6 Therefore, in order to represent this matrix, you will construct int 2D arrays with the values passed in from the user just like so: o [{1,2,3}, {4,5,6}] When you finish prompting the user and your calculations, at the very end, print out "Goodbye!" Be sure to look at the sample output below as well to know the format of these questions! Operations There will be 3 operations that you will do based on user input: add, multiply, and transpose. Add In order to add two matrices, the number of rows an columns must be the same for both matrices. o For example: Valid: A [1 2 3] 14 5 6 BC + 11 3 3 = 12 5 6 || 14 2 71 18 7 13|| Invalid: [1 2 3] 14 5 6 + [13] Cannot add 14 21 because number of rows and columns do not match. If the user inputs invalid matrices that cannot be added, simply print out "Sorry, matrices are invalid and print "Goodbye!" When we add two matrices, we add every value in each spot in A with the corresponding value and spot in B. Transpose Transposing a matrix simply means changing its rows to be its columns and vice versa For example: A transposed 11 2 3| 14 5 6| ------> 11 41 12 51 13 6 Each value in the first row becomes the values in the first column of the transposed matrix. Same goes for the second row as well. Multiplication When multiplying two matrices, we have to take into consideration the number of rows and columns of both matrices. If we have two matrices A and B, the # of columns in A must match the # of rows in B. For example: A 11 2 3 14 5 61 B X 11 31 14 21 Valid 13 71 D X 11 31 14 21 Invalid C 1 2 3 14 5 6 A has 2 rows and 3 columns and B has 3 rows and 2 columns. The number of columns in A matches the number of rows in B, therefore we can multiply them. Chas 2 rows and 3 columns but D has 2 rows and 2 columns. The number of columns in C (which is 3) does not match the number of rows in D (which is 2). If the matrices don't have matching columns and rows, then simply print out "Sorry, cannot multiply matrices" and print "Goodbye!". Now for the actual multiplication. In order to multiply: 1. Take the first row of A and the first column of B and multiply each value in A with its corresponding value in B and add them together. This will be the first value in the first spot of your new matrix, or index (0,0). Notice that the index for the value will always be A_row, B_column] (1 2 3] x [1] 14 131 -> 1*1 + 2*4 + 3+3 = 18 New Matrix M = 18 - - - //NOTE: Resulting matrix will be a 2x2. We take the # of rows from A and # of columns from B to determine dimensions of the new matrix. // A B SOM 2 x 3 3 X 2 2 x 2 0 2. Now we repeat this step for each row and column of A and B: [1 2 3] x 131 121 171 -> 1*3 + 2*2 + 3*7 = 28 M = 118 281 //Notice that 28 goes in index [0, 1] //because we're looking at the row in //index of A and the column in //index 1 of B //Now, the row in index 1 of A [4 5 6] x [1|| 141 13 -> 4*1 + 5*4 + 6*3 = 42 M = 118 28| 142 | //column in index 1 of B (again) [4 5 6] x 13 121 17] -> 4*3 + 5*2 + 6*7 = 64 M = 118 28| | 42 641 Multiplication can, of course, be performed on matrices much largeryour code should work for a matrix of any length. Sample Output What type of operation do you want to do? Transpose Enter the matrix 1 2 3; 4 5 6; 7 8 9 Goodbye! What type of operation do you want to do? Add What is the first matrix? 4 5 9; 1 3 2 What is the second matrix? 8 1 2; 4 3 2 Goodbye! What type of operation do you want to do? Add What is the first matrix? 4 5 9: 1 3 2 What is the second matrix? 8 1; 4 3 Sorry matrices are invalid! Goodbye! You will be writing a class called Matrix Operations.java that will perform certain operations based on user input. Requirements Prompts to user Here we are going to discuss the prompts to the user. First prompt the user What type of operation do you want to do? If the user inputs transpose, ask "Enter the matrix" Otherwise, ask "What is the first matrix?. Then ask What is the second matrix? When you ask the user for each matrix, you can assume the user will input numbers in the following format: What is the first matrix? 1 2 3; 4 5 6; The semi colon represents each row so the matrix above will be: row row col col col 11 2 3|| 14 5 6 Therefore, in order to represent this matrix, you will construct int 2D arrays with the values passed in from the user just like so: o [{1,2,3}, {4,5,6}] When you finish prompting the user and your calculations, at the very end, print out "Goodbye!" Be sure to look at the sample output below as well to know the format of these questions! Operations There will be 3 operations that you will do based on user input: add, multiply, and transpose. Add In order to add two matrices, the number of rows an columns must be the same for both matrices. o For example: Valid: A [1 2 3] 14 5 6 BC + 11 3 3 = 12 5 6 || 14 2 71 18 7 13|| Invalid: [1 2 3] 14 5 6 + [13] Cannot add 14 21 because number of rows and columns do not match. If the user inputs invalid matrices that cannot be added, simply print out "Sorry, matrices are invalid and print "Goodbye!" When we add two matrices, we add every value in each spot in A with the corresponding value and spot in B. Transpose Transposing a matrix simply means changing its rows to be its columns and vice versa For example: A transposed 11 2 3| 14 5 6| ------> 11 41 12 51 13 6 Each value in the first row becomes the values in the first column of the transposed matrix. Same goes for the second row as well. Multiplication When multiplying two matrices, we have to take into consideration the number of rows and columns of both matrices. If we have two matrices A and B, the # of columns in A must match the # of rows in B. For example: A 11 2 3 14 5 61 B X 11 31 14 21 Valid 13 71 D X 11 31 14 21 Invalid C 1 2 3 14 5 6 A has 2 rows and 3 columns and B has 3 rows and 2 columns. The number of columns in A matches the number of rows in B, therefore we can multiply them. Chas 2 rows and 3 columns but D has 2 rows and 2 columns. The number of columns in C (which is 3) does not match the number of rows in D (which is 2). If the matrices don't have matching columns and rows, then simply print out "Sorry, cannot multiply matrices" and print "Goodbye!". Now for the actual multiplication. In order to multiply: 1. Take the first row of A and the first column of B and multiply each value in A with its corresponding value in B and add them together. This will be the first value in the first spot of your new matrix, or index (0,0). Notice that the index for the value will always be A_row, B_column] (1 2 3] x [1] 14 131 -> 1*1 + 2*4 + 3+3 = 18 New Matrix M = 18 - - - //NOTE: Resulting matrix will be a 2x2. We take the # of rows from A and # of columns from B to determine dimensions of the new matrix. // A B SOM 2 x 3 3 X 2 2 x 2 0 2. Now we repeat this step for each row and column of A and B: [1 2 3] x 131 121 171 -> 1*3 + 2*2 + 3*7 = 28 M = 118 281 //Notice that 28 goes in index [0, 1] //because we're looking at the row in //index of A and the column in //index 1 of B //Now, the row in index 1 of A [4 5 6] x [1|| 141 13 -> 4*1 + 5*4 + 6*3 = 42 M = 118 28| 142 | //column in index 1 of B (again) [4 5 6] x 13 121 17] -> 4*3 + 5*2 + 6*7 = 64 M = 118 28| | 42 641 Multiplication can, of course, be performed on matrices much largeryour code should work for a matrix of any length. Sample Output What type of operation do you want to do? Transpose Enter the matrix 1 2 3; 4 5 6; 7 8 9 Goodbye! What type of operation do you want to do? Add What is the first matrix? 4 5 9; 1 3 2 What is the second matrix? 8 1 2; 4 3 2 Goodbye! What type of operation do you want to do? Add What is the first matrix? 4 5 9: 1 3 2 What is the second matrix? 8 1; 4 3 Sorry matrices are invalid! Goodbye