Question
Please Please Do not copy from other experts Please Please Make sure to complete all 4 java codes and they compile =================================================================== Ride.java (represents any
Please Please Do not copy from other experts
Please Please Make sure to complete all 4 java codes and they compile
===================================================================
Ride.java (represents any amusement park ride. Ride should never be instantiated. Instead, must only serve as a superclass for more specific Rides.)
Variables:
1. id (String) - the identifier for the ride. - must be immutable after construction of an instance and visible only to subclasses of Ride.
2. earnings (double) - the total amount of money this ride has earned that day. - must be visible only to the subclasses of Ride.
3. runsSinceInspection (int) - the number of times the ride has run since the last inspection. - must be visible only to the subclasses of Ride.
4. passengers (array of Strings) - the passengers aboard the ride. Each item in the array represents a passengers name. - must be visible only to subclasses of Ride.
Constructors:
- takes in id, runsSinceInspection, passengers, and initializes earnings to a default value of 0. - takes in id, passengers, and initializes both earnings and runsSinceInspection to a default value of 0.
Methods:
1. canRun - Takes in an int number of runs and returns a boolean that represents whether the ride can run that many times before it must be inspected. - This method will not have an implementation in the Ride class. HINT: What keyword allows us to declare a method in a class without providing an implementation? 2. inspectRide - Takes in an array of Strings that represent different components of the ride. - Resets runsSinceInspection to 0 if the ride passes inspection and returns true. - Returns false if the ride is does not pass inspection. - This method will not have an implementation in the Ride class. HINT: What keyword allows us to declare a method in a class without providing an implementation? 3. costPerPassenger - Takes in an int number of stops and returns a double cost for the passenger to ride to that many stops. - This method will not have an implementation in the Ride class. HINT: What keyword allows us to declare a method in a class without providing an implementation? 4. addPassengers - Takes in an int number of stops and an array of Strings with passenger names. - Returns true if all passengers can fit on the ride and the ride can travel the given number of stops. - Returns false if all passengers cannot fit on the ride or the ride cannot travel the given number of stops without inspection. - This method will not have an implementation in the Ride class. HINT: What keyword allows us to declare a method in a class without providing an implementation? 5. getPassengerList - Returns a list of passengers, one per line, as a String. - Only non-null elements should be listed. - This method has no parameters. - This method will be very helpful during testing adding and removing of passengers from the Ride. - For a passenger list of [null, Ariel, null, Nicolas, Ricky], the output should be in the following format:
Passenger List for
- If there are no passengers, the list will simply contain the first line in the sample output above. - There should not be a trailing newline character at the end of the String. 6. chargePassenger - Takes in an int number of stops and increases earnings accordingly. - This method should return nothing. 7. removePassenger - Sometimes passengers violate rules or dont meet ride requirements. - This method will take in the name of a passenger. - If the name is not in the passenger array, return false. - If the name is in the passenger array, set that index to null and return true. IMPORTANT: This method should only remove the first occurrence of the named passenger, case-insensitive. 8. equals - Overrides from Object. - Two Rides are equal if they have the same id and runsSinceInspection. 9. toString - Overrides from Object. - Returns the String:
=============================================================
RollerCoaster.java (represents a regular roller coaster in your amusement park. It must be a subclass of Ride.)
Variable:
1. rate (double) - the price of one run.
2. photoFees (double) - the total fees to purchase the mandatory photo package. Out of the generosity of our hearts, the ride may be ridden multiple times and the fee must only be paid once.
3. maxNumRuns (int) - the total number of times that the ride can run before it must be inspected.
Constructors:
- takes in id, runsSinceInspection, passengers, rate, photoFees, and maxNumRuns. - takes in id, runsSinceInspection, maxNumRuns, and defaults passengers to an empty String array of length 4, rate to 10, and photoFees to 15. - takes in id, defaults runsSinceInspection to 0, passengers to an empty String array of length 4, rate to 10, photoFees to 15, and maxNumRuns to 200.
Methods:
1. canRun - Takes in an int number of runs and returns whether the RollerCoaster can run that many times before needing an inspection. - Make sure to consider how many times the RollerCoaster has run already. - A ride cannot run a negative number of times. 2. inspectRide - Takes in an array of String that represent different components of the ride. You may assume that the array of Strings passed in will not be null. - The array must contain the Strings Tracks Clear and Brakes Ok for the ride to pass inspection. - The array may also contain other Strings in any order. - The characters of these Strings may be uppercase or lowercase. - Resets runsSinceInspection to 0 if the ride passes inspection and returns true. - Returns false if the ride does not pass inspection. 3. costPerPassenger - Takes in an int number of stops and returns the double cost for the RollerCoaster to run that many times. Use the descriptions for the rate and photoFees fields to determine the cost per stop. NOTE: A stop for a RollerCoaster is an entire run. 4. addPassengers - Takes in an int number of stops and an array of Strings with passenger names. - This array will not contain null elements. - Assume the array will have a length of at least 1. - The Roller Coaster should be able to complete the requested number of runs without exceeding maxNumRuns, or the passengers cannot be added. - For each passenger, add them to the passengers array, starting from the lowest available index. Only add the passengers if there are seats for ALL passengers on the RollerCoaster and if the RollerCoaster can run the number of times specified! - Seats are available if the corresponding element in the array does not already have a passenger assigned. - Do not assume that passengers will always be at the front of the array; empty seats may be spread throughout the array. The passenger array can be given to the constructor, and its state could be [null, Ariel, Nicolas, null]. HINT: Its a good idea to write a private helper method to count the number of empty seats.
- If all passengers fit on the RollerCoaster, then charge each passenger for their ride. Remember that no matter how many passengers there are, you will only increase runsSinceInspection once for each stop. HINT: Reuse code! What method have we already created to do this? - Returns true if all customers can fit on the RollerCoaster and the RollerCoaster can travel the given number of runs. - Returns false if the customers cannot fit on the RollerCoaster or the RollerCoaster cannot travel the given number of runs. IMPORTANT: No passengers should be added if the entire group cannot fit. 5. equals - Overrides from Ride. - Two roller coasters are equal if they have the same id, runsSinceInspection, rate, photoFees, and maxNumRuns. 6. toString - Overrides from Ride. - Returns the String: Roller Coaster
Trolley.java (represents a regular trolley in your transport network. It must be a subclass of Ride.)
Variables:
1. stations (stored in a String array.) - the general areas of Trolley routes.
2. currentStation - the general area of the Trolleys current station. - For example, it could be Midtown, Atlantic Station, Downtown, or more. HINT: The current station is an index to an element in the stations array.
Constructor(s): - takes in id, runsSinceInspection, stations, currentStation, and defaults a String array of length 20 for passengers. - takes in id, stations, currentStation, and defaults runsSinceInspection to 0, and passengers to a String array of length 20.
Methods:
1. canRun - Takes in an int number of runs and returns whether the trolley can run that many loops. - Since trolleys are well kept, they will always be ready to drive, unless the number of runs is negative. 2. inspectRide - Takes in an array of Strings that represent different components of the ride. - The array must contain the Strings, Gas Tank Not Empty and Brakes Ok for the ride to pass inspection. - The array may also contain other Strings in any order. - The characters of these Strings may be uppercase or lowercase. - Resets runsSinceInspection to 0 if the ride passes inspection and returns true. - Returns false if the ride does not pass inspection. 3. costPerPassenger - Takes in an int number of stops and returns the double cost for the passenger to travel that number of stops. - The cost is calculated by taking the number of stops multiplied by 3 and then dividing by the number of stations per loop. In other words, the fewer stops a trolley makes the more itll cost to ride! 4. addPassengers - Takes in an int number of stops and an array of Strings with passenger names. - This array will not contain nulls. - Assume the array will have a length of at least 1. - For each passenger, add them to the passengers array, starting from the lowest available index. Only add passenger names to the passengers array if the Trolley can travel the requested number of stops. - Seats are available if the corresponding element in the array does not already have a passenger assigned. - Do not assume that passengers will always be at the front of the array; empty seats may be spread throughout the array. The passenger array can be given to the constructor, and its state could be [null, Ariel, Nicolas, null]. - Not all passengers from the passed in array need to fit on the trolley. Add the passenger names to the passengers array in order, starting from the front of the array. If there are passengers that cannot fit on the trolley, do nothing with them. - Charge each passenger that boards the trolley for the number of stops they are travelling. - Each rider gets charged for their trip separately, but be sure that runsSinceInspection is increased correctly for each trip because everyone is riding together. For example, if 3 people board at once, runsSinceInspection should only increase by completed runs for the number of stops in the trip. HINT: use moveTrolley to update it! - Returns true if the trolley can travel the given number of loops. - Returns false if the trolley cannot travel the given number of loops. 5. equals - Overrides from Object. - Two Trolleys are equal if they have the same id, runsSinceInspection, currentStation, and stations. - Note that Trolley stations are equal if and only if they have the same names (case- insensitive) and are in the same order. 6. moveTrolley - Takes in the number of stations to advance and returns nothing. - Increments runsSinceInspection for the number of loops that will be completed after travelling the requested number of stops. - A loop is completed after leaving the last station and returning to the first. - Sets currentStation to the next station on the route after travelling the requested number of stops. Think carefully and test, test, test! 7. toString - Overrides from Ride. - Returns the String: Trolley
====================================
Driver.java (test with main)
Methods: 1. main - Create 2 RollerCoaster objects - Call addPassengers() on at least one of the RollerCoaster objects - Call toString() on both RollerCoaster objects - Check to see if the 2 RollerCoaster objects are equal - Create 2 Trolley objects - Call addPassengers() on at least one of the Trolley objects - Call toString() on both Trolley objects - Check to see if the 2 Trolley objects are equal
Step by Step Solution
There are 3 Steps involved in it
Step: 1
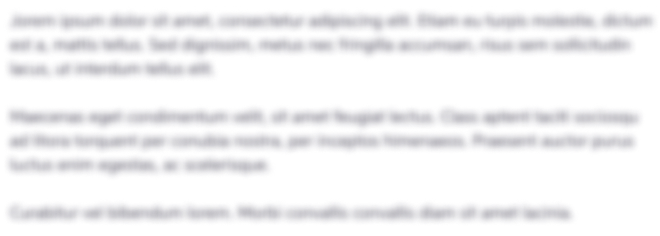
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started