Question
please please help me fix my code please use c++ and show your output question Base class Employee The Employee class below is the parent
please please help me fix my code
please use c++ and show your output
question
Base class Employee
The Employee class below is the parent class. You can add an arg-constructor to the Employee class if needed but do not change the provided code. An employee's net pay is calculated biweekly according to the additional attributes. You will define two child classes, HourlyEmployee and SalariedEmployee.
class Employee {
private:
string code; "S" for Salaried Employee, "H" for Hourly Employee
string ssn;
string first;
string last;
string department;
string role;
double netPay;
static int count;
public:
Employee(); //constructor
~Employee();
string getCode() const;
void setCode(string);
string getSSN() const;
void setSSN(string);
string getFirst()const;
void setFirst(string);
string getLast() const;
void setLast(string);
string getDept() const;
void setDept(string);
string getRole() const;
void setRole(string);
double getNetPay() const;
void setNetPay(double);
void display();
static int getEmpCount();
};
#endif
Child Classes : HourlyEmployee and SalariedEmployee
The HourlyEmployee class has two additional attributes, payRate as double and workHours as int, whereas the SalariedEmployee class has only one additional attribute, biweeklySalary. Since the net pay of an employee is calculated biweekly, the net pay of a salaried employee will be the same as biweeklySalary.
Testing the classes
By utilizing the provided employee.txt, build an array of hourly employees in the heap and an array of salaried employees in the stack. Both arrays have the size of 3. Your program is required to display the following.
R1: The sequence of constructor calls
R2: The information of all the employees that includes net pays but not pay rate nor work hours.
R3: Changes in employee count
R4: The sequence of destructor call
here is my code
Employee.h
#ifndef EMPLOYEE_H #define EMPLOYEE_H #include #include using namespace std; class Employee { private: string code; //"S" for Salaried Employee, "H" for Hourly Employee string ssn; string first; string last; string department; string role; double netPay; static int count; public: Employee(); //constructor Employee(string code,string ssn,string fname,string lname,string dep,string role); ~Employee(); string getCode() const; void setCode(string); string getSSN() const; void setSSN(string); string getFirst()const; void setFirst(string); string getLast() const; void setLast(string); string getDept() const; void setDept(string); string getRole() const; void setRole(string); double getNetPay() const; void setNetPay(double); void display(); static int getEmpCount(); }; #endif |
####################################################################
Employee.cpp
#pragma once #include "Employee.h" int Employee::count = 0; //Default constructor Employee::Employee(){ Employee::count += 1; } //Arg-constructor Employee::Employee(string code,string ssn,string fname,string lname,string dep,string role){ setCode(code); setSSN(ssn); setFirst(fname); setLast(lname); setDept(dep); setRole(role); Employee::count += 1; } //Destructor Employee::~Employee(){ if(code == "H") cout << "Houred employee destructed." << endl; else if(code == "S") cout << "Salaried employee destructed." << endl; } //Getters string Employee::getCode() const{ return this->code; } string Employee::getSSN() const{ return this->ssn; } string Employee::getFirst() const{ return this->first; } string Employee::getLast() const{ return this->last; } string Employee::getDept() const{ return this->department; } string Employee::getRole() const{ return this->role; } double Employee::getNetPay() const{ return this->netPay; } int Employee::getEmpCount(){ return count; } //Setters void Employee::setCode(string code){ this->code = code; } void Employee::setSSN(string ssn){ this->ssn = ssn; } void Employee::setFirst(string fname){ this->first = fname; } void Employee::setLast(string lname){ this->last = lname; } void Employee::setDept(string dep){ this->department = dep; } void Employee::setRole(string role){ this->role = role; } void Employee::setNetPay(double pay){ this->netPay = pay; } //Display void Employee::display(){ cout << "Code : " << getCode() << endl; cout << "SSN : " << getSSN() << endl; cout << "Name : " << getFirst() << " " << getLast() << endl; cout << "Department : " << getDept() << endl; cout << "Role : " << getRole() << endl; cout << "Net Pay : " << getNetPay() << endl; } |
####################################################################
Step by Step Solution
There are 3 Steps involved in it
Step: 1
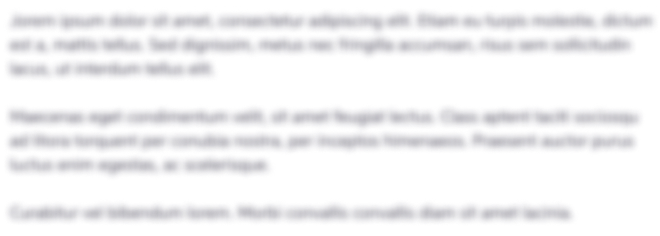
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started