Question
Please provide a runnable source code. Hints: 1) The parent thread initializes an unsorted array with integers numbers. The easiest approach for sharing data is
Please provide a runnable source code.
Hints:
1) The parent thread initializes an unsorted array with integers numbers. The easiest approach for sharing data is to create global data. For example, at file scope:
#define SIZE ( sizeof(list)/sizeof(*list) )
int list[] = { 7,12,19,3,18,4,2,-5,6,15,8};
int result[SIZE] = {0};
2) The parent thread then creates sorter threads, passing each a parameter containing the location (pointer) and size (unsigned) of the unsorted array. The easiest approach for passing data to threads is to create a data structure using a typedef and struct. For example,
typedef struct
{ int * subArray;
unsigned int size;
} SortingThreadParameters;
typedef struct
{ SortingThreadParameters left;
SortingThreadParameters right;
} MergingThreadParameters;
3) The parent creates worker threads using a strategy similar to:
SortingThreadParameters * paramsLeft = malloc( sizeof (SortingThreadParameters) );
paramsLeft -> subarray = list;
paramsLeft -> size = SIZE/2;
// Now create the first soting thread passing it paramsLeft as a parameters
SortingThreadParameters * paramsRight = malloc (sizeof (SortingThreadParameters ) );
paramsRight -> subarray = list + paramsLeft -> size;
paramsRight -> size = SIZE - paramsLeft -> size;
//Now create the second sorting thread passing it paramsRight as a parameter
//wait for the sorting threads to complete
MergingThreadParameters * paramsMerge = malloc ( sizeof (SortingThreadParameters) );
paramsMerge -> left = paramsLeft;
paramsMerge -> right = paramsRight;
//Now create the merging thread passing it paramsMerge as a parameter
4) The pointer-to-parameter (e.g paramsLeft, paramsRight, and paramsMerge) is passed to the pthread_create() function, which in turn is passed as a parameter to the function that is to run as a seperated thread.
5) The sorting threads perform an in-place sort. The merging thread does not.
1) (20 pts) Write a POSIX multithreaded sorting program (in C) called sorting.c that works as follows: A list of integers is divided into two smaller lists of (nearly)equal size. Two separate worker threads (which we will term sorting threads) sort each sublist using a sorting algorithm of your choice. The two sublists are then merged by a third worker thread a merging thread which merges the two sorted sublists into a single sorted list. Because we are striving for Data Parallelism (same algorithm on different data) and because global data are shared across all threads, perhaps the easiest way to set up the data is to create a global (file scoped) array. Each sorting thread will work on one half of this array. A second global array of the same size as the unsorted integer array will also be established. The merging thread will then merge the two sublists into this second array. Graphically, this program is structured as original list 7,12, 19,3,18,4, 2,6,15, 8 sorting shreadi threado 7, 12, 19,3, 18 4, 2,6,15,8 merge thread 2,3,4,6,7,8, 12, 15, 18,19 sorted list This programming project will require passing parameters to each of the sorting threads. In particular, it will be necessary to identify the starting address from which each thread is to begin sorting, and the number of items to be sorted. See Hints below The parent thread will output the sorted array once all sorting has completed Other information: 1. The list of integers to be sorted is (use the exact values and order provided below) 17, 12, 19, 3, 18, 4, 2, -5, 6, 15, 8) 2. gcc-pthread sorting.c -osorting is an example command to compile and link your program 3. ./sorting collecting your program's output to a text file and seeing the output on the console I tee sorting_output.txt is an example command to run your program while 1) (20 pts) Write a POSIX multithreaded sorting program (in C) called sorting.c that works as follows: A list of integers is divided into two smaller lists of (nearly)equal size. Two separate worker threads (which we will term sorting threads) sort each sublist using a sorting algorithm of your choice. The two sublists are then merged by a third worker thread a merging thread which merges the two sorted sublists into a single sorted list. Because we are striving for Data Parallelism (same algorithm on different data) and because global data are shared across all threads, perhaps the easiest way to set up the data is to create a global (file scoped) array. Each sorting thread will work on one half of this array. A second global array of the same size as the unsorted integer array will also be established. The merging thread will then merge the two sublists into this second array. Graphically, this program is structured as original list 7,12, 19,3,18,4, 2,6,15, 8 sorting shreadi threado 7, 12, 19,3, 18 4, 2,6,15,8 merge thread 2,3,4,6,7,8, 12, 15, 18,19 sorted list This programming project will require passing parameters to each of the sorting threads. In particular, it will be necessary to identify the starting address from which each thread is to begin sorting, and the number of items to be sorted. See Hints below The parent thread will output the sorted array once all sorting has completed Other information: 1. The list of integers to be sorted is (use the exact values and order provided below) 17, 12, 19, 3, 18, 4, 2, -5, 6, 15, 8) 2. gcc-pthread sorting.c -osorting is an example command to compile and link your program 3. ./sorting collecting your program's output to a text file and seeing the output on the console I tee sorting_output.txt is an example command to run your program while
Step by Step Solution
There are 3 Steps involved in it
Step: 1
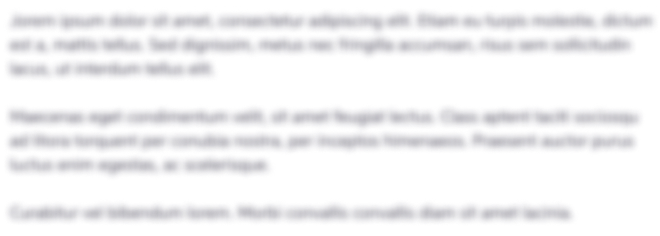
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started