Question
Please provide comments. Thank You 1 Problem Specification For this programming assignment, you will be writing software in support of a grocery store which sells
Please provide comments. Thank You
1 Problem Specification
For this programming assignment, you will be writing software in support of a grocery store which sells rice by the pound, eggs by the dozen, baguette, and flavored baguette (baguette flavored with chocolate, vanilla, garlic, caramel, etc). Your software will be used for the checkout system.
To do this, you will implement an inheritance hierarchy of classes derived from an Item superclass:
The Rice, Egg, and Baguette classes will be derived from the Item class.
The FlavoredBaguette class will be derived from the Baguette class. You will also write a Checkout class which maintains a list of Items.
1.1 The Item Class
The Item class is a superclass from which specific types of Items can be derived. It contains only one data member, a name. It also defines a number of methods. All of the Item class methods in a generic way in the file, Item.java, provided for you along with the other specific files in a directory in moodle. The getCost() method is a method that merely returns zero in the Item class because the method of determining the costs varies based on the type of item and needs to be overridden in every subclass. Tax amounts should be rounded to the nearest cent. For example, the calculating the tax on a food item with a cost of 199 cents with a tax rate of 2.0% should be 4 cents.
DO NOT CHANGE THE Item.java file! Your code must work with this class as it is provided.
1.2 The GroceryStore Class
The GroceryStore class is also provided for you in the file, GroceryStore.java. It contains constants such as the tax rate as well the name of the store, the maximum size of an item name and the width used to display the costs of the items on the receipt. Your code should use these constants wherever necessary! The GroceryStore class also contains the
1
cents2dollarsAndCents method which takes an integer number of cents and returns it as a String formatted in dollars and cents. For example, 105 cents would be returned as 1.05. DO NOT CHANGE THE GroceryStore.java file! Your code must work with this class
as it is provided.
1.3 The Derived Classes
All of the classes which are derived from the Item class must define a constructor. Please see the provided TestCheckout class,TestCheckout.java , to determine the parameters for the various constructors. Each derived class should be implemented by creating a file with the correct name; e.g. Rice.java.
The Rice class should be derived from the Item class. A Rice item has a weight and a price per pound which are used to determine its cost. For example, 2.30 lbs.of rice @ .89 /lb. = 205 cents. The cost should be rounded to the nearest cent.
The Egg class should be derived from the Item class. An Egg item has a number and a price per dozen which are used to determine its cost. For example, 4 eggs @ 399 cents /dz. = 133 cents. The cost should be rounded to the nearest cent.
The Baguette class should be derived from the Item class. A Baguette item simply has a cost.
The FlavoredBaguette class should be derived from the Baguette class. The cost of a FlavoredBaguette is the cost of the Baguette plus the cost of its flavor.
1.4 The Checkout Class
The Checkout class, provides methods to enter grocery items into the cash register, clear the cash register, get the number of items, get the total cost of the items (before tax), get the total tax for the items, and get a String representing a receipt for the grocery items (you need to override the toString method of Object class). The Checkout class needs to store a list of Items. The total tax should be rounded to the nearest cent.
1.5 Testing
A simple testdriver, TestCheckout.java along with its expected output, are provided for you to test your class implementations. You can add additional tests to the driver to more thoroughly test your code.
public class GroceryStore {
public final static double TAX_RATE = 6.5; // 6.5% public final static String STORE_NAME = "Best Grocery Store Ever"; public final static int MAX_ITEM_NAME_SIZE = 25; public final static int COST_WIDTH = 6; public static String cents2dollarsAndCents(int cents) { String s = ""; if (cents < 0) { s += "-"; cents *= -1; } int dollars = cents/100; cents = cents % 100; if (dollars > 0) s += dollars; s +="."; if (cents < 10) s += "0"; s += cents; return s; } }
public class Item { protected String name; /** * Null constructor for Item class */ public Item() { this(""); } /** * Initializes Item data */ public Item(String name) { if (name.length() <= GroceryStore.MAX_ITEM_NAME_SIZE) this.name = name; else this.name = name.substring(0,GroceryStore.MAX_ITEM_NAME_SIZE); } /** * Returns name of Item * @return name of Item */ public String getName() { return name; } /** * Returns cost of Item * @return cost of Item */ public int getCost(){ return 0; }
}
public class TestCheckout {
public static void main(String[] args) { Checkout checkout = new Checkout(); checkout.enterItem(new Rice("Basmati Rice", 2.25, 399)); checkout.enterItem(new Baguette("Wheat Baguette",105)); checkout.enterItem(new FlavoredBaguette("White Baguette",145, "Chocolate", 50)); checkout.enterItem(new Egg("Grade A Organic Eggs", 4, 399)); System.out.println(" Number of items: " + checkout.numberOfItems() + " "); System.out.println(" Total cost: " + checkout.totalCost() + " "); System.out.println(" Total tax: " + checkout.totalTax() + " "); System.out.println(" Cost + Tax: " + (checkout.totalCost() + checkout.totalTax()) + " "); System.out.println(checkout); checkout.clear(); checkout.enterItem(new Baguette("Organic Baguette",145)); checkout.enterItem(new FlavoredBaguette("Wheat Baguette",105, "Caramel", 50)); checkout.enterItem(new Rice("Indian Brown Rice", 1.33, 89)); checkout.enterItem(new Egg("Grade B Egg", 4, 399)); checkout.enterItem(new Rice("Arabic White Rice", 1.5, 209)); checkout.enterItem(new Rice("Spanish Yellow Rice",3.0, 109)); System.out.println(" Number of items: " + checkout.numberOfItems() + " "); System.out.println(" Total cost: " + checkout.totalCost() + " "); System.out.println(" Total tax: " + checkout.totalTax() + " "); System.out.println(" Cost + Tax: " + (checkout.totalCost() + checkout.totalTax()) + " "); System.out.println(checkout); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
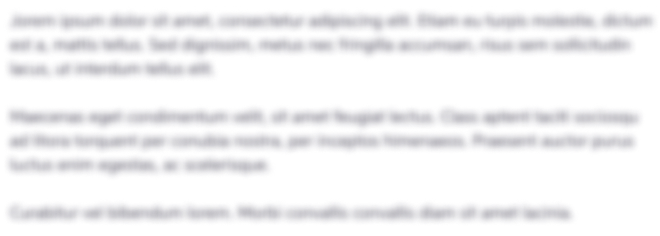
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started