Question
Please Provide me all necssary Screenshort and Commentds:(I use eclipse) Requirements For this program you will be asked to write a simple data management program.
Please Provide me all necssary Screenshort and Commentds:(I use eclipse)
Requirements
For this program you will be asked to write a simple data management program. Start by choosing what sort of thing you would like to keep track of. For example,
Student information
Vacation or travel information
Information about courses
Plants
Pets
Food
Real estate properties
Any set of similar "things"
Your program will manage your data collection. We will start with the simplest collection of data, a list. Your list will contain the Things of your choice.
Requirements for YourChoiceOfThing Class
Implement the sort of Thing to be stored as a class. The object/class must have the following characteristics:
At least 5 attributes/characteristics
At least one numeric attribute
At least one String attribute
One String attribute should be usable as a search key (for example, the name of a Food item)
Implement getters and setters for all the attributes of your Thing of choice.
Implement an equals() method for your Thing class.
Requirements for the CollectionOfYourThings Class
Create a collection of Things using an array. You may NOT use the library classes ArrayList or Vector.
Implement a constructor which accepts as an argument the maximum size of the data collection.
Implement a search method which returns a single object.
Implement an add, update, delete methods.
User Interface Class
Use the user interface class provided for you in class. A soft copy is available for download from D2L.
Alter the user interface class to use YOUR classes. Change the names of the classes to reflect your item of interest.
Allow the user to:
Enter data and store it in the list.
Update an entry in the list
Delete an entry from the list
Search for an item in the list.
Duplicates should not be allowed.
Display the complete list. The list which is displayed should accurately reflect the items stored in the data structure. That is, if an item is updated or deleted, the list which is displayed should show that.
Given a search key by the user, search your list for a matching object. Retrieve and display all information for the matching object. The search key should be CASE INSENSITIVE. For example, ITEM should be a match for item or IteM. Your search key must be a string, not a number.
Display the number of items in the list.
Use of a graphical user interface such as swing is required.
Grading Criteria
Methods must be of appropriate length usually less than 1 page in length
Appropriate private and public access modifiers must be used. Almost always data is private and methods are public although you may certainly have private or protected methods if appropriate.
User interface is intuitive and easy to use.
Meets program specifications as described above
The program is robust with no runtime errors or problems
Program is readable
Comments
Use Javadocs conventions (Appendix H)
Include comments on the class as a whole including
Description of program
Your name (use @author)
Date (due or date you started or last modified)
Source of any borrowed code
For each method include
Concise description of what method does
Arguments for each method (use @param)
Returned value (use @returns)
Exceptions which are thrown (@throws)
Consistent and correct indenting
Meaningful identifiers
Normal capitalization conventions are used
The source of any "borrowed" code is clearly identified
ArrayBag.java
import java.util.Iterator;
// File: ArrayBag.java from the package edu.colorado.collections
// Additional javadoc documentation is available from the IntArrayBag link in:
// http://www.cs.colorado.edu/~main/docs
/******************************************************************************
* An ArrayBag is a collection of Item objects.
*
* @note
* (1) The capacity of one of these bags can change after it's created, but
* the maximum capacity is limited by the amount of free memory on the
* machine. The constructor, addItem, clone,
* and union will result in an OutOfMemoryError
* when free memory is exhausted.
*
* (2) A bag's capacity cannot exceed the maximum integer 2,147,483,647
* (Integer.MAX_VALUE). Any attempt to create a larger capacity
* results in a failure due to an arithmetic overflow.
*
* (3) Because of the slow linear algorithms of this
* class, large bags will have poor performance.
*
* @author Michael Main
*
* @version
* Jul 5, 2005
*
******************************************************************************/
public class ArrayBag implements Cloneable
{
// Invariant of the ArrayBag class:
// 1. The number of elements in the bag is in the instance variable
// manyItems, which is no more than data.length.
// 2. For an empty bag, we do not care what is stored in any of data;
// for a non-empty bag, the elements in the bag are stored in data[0]
// through data[manyItems-1], and we dont care whats in the
// rest of data.
private Item[ ] data;
private int manyItems;
/**
* Initialize an empty bag with an initial capacity of 10. Note that the
* addItem method works efficiently (without needing more
* memory) until this capacity is reached.
* @param - none
* @postcondition
* This bag is empty and has an initial capacity of 10.
* @exception OutOfMemoryError
* Indicates insufficient memory for:
* new int[10].
**/
public ArrayBag( )
{
final int INITIAL_CAPACITY = 10;
manyItems = 0;
data = new Item[INITIAL_CAPACITY];
}
/**
* Initialize an empty bag with a specified initial capacity. Note that the
* addItem method works efficiently (without needing more
* memory) until this capacity is reached.
* @param initialCapacity
* the initial capacity of this bag
* @precondition
* initialCapacity is non-negative.
* @postcondition
* This bag is empty and has the given initial capacity.
* @exception IllegalArgumentException
* Indicates that initialCapacity is negative.
* @exception OutOfMemoryError
* Indicates insufficient memory for: new int[initialCapacity].
**/
public ArrayBag(int initialCapacity)
{
if (initialCapacity < 0)
throw new IllegalArgumentException
("The initialCapacity is negative: " + initialCapacity);
data = new Item[initialCapacity];
manyItems = 0;
}
/**
* Add a new element to this bag. If the new element would take this
* bag beyond its current capacity, then the capacity is increased
* before adding the new element.
* @param element
* the new element that is being inserted
* @postcondition
* A new copy of the element has been added to this bag.
* @exception OutOfMemoryError
* Indicates insufficient memory for increasing the bag's capacity.
* @note
* An attempt to increase the capacity beyond
* Integer.MAX_VALUE will cause the bag to fail with an
* arithmetic overflow.
**/
public void add(String element)
{
if (manyItems == data.length)
{ // Ensure twice as much space as we need.
ensureCapacity((manyItems + 1)*2);
}
// TODO - verify item does not currently exist
if (data[manyItems] == null)
data[manyItems] = new Item();
data[manyItems].setData1(element);
manyItems++;
}
/**
* Generate a copy of this bag.
* @param - none
* @return
* The return value is a copy of this bag. Subsequent changes to the
* copy will not affect the original, nor vice versa.
* @exception OutOfMemoryError
* Indicates insufficient memory for creating the clone.
**/
public ArrayBag clone( )
{ // Clone an IntArrayBag object.
ArrayBag answer;
try
{
answer = (ArrayBag) super.clone( );
}
catch (CloneNotSupportedException e)
{ // This exception should not occur. But if it does, it would probably
// indicate a programming error that made super.clone unavailable.
// The most common error would be forgetting the "Implements Cloneable"
// clause at the start of this class.
throw new RuntimeException
("This class does not implement Cloneable");
}
answer.data = data.clone( );
return answer;
}
/**
* Accessor method to count the number of occurrences of a particular element
* in this bag.
* @param target
* the element that needs to be counted
* @return
* the number of times that target occurs in this bag
**/
public int countOccurrences(String target)
{
int answer;
int index;
answer = 0;
for (index = 0; index < manyItems; index++)
if (target.equals(data[index].getData1()))
answer++;
return answer;
}
/**
* Change the current capacity of this bag.
* @param minimumCapacity
* the new capacity for this bag
* @postcondition
* This bag's capacity has been changed to at least minimumCapacity.
* If the capacity was already at or greater than minimumCapacity,
* then the capacity is left unchanged.
* @exception OutOfMemoryError
* Indicates insufficient memory for: new int[minimumCapacity].
**/
public void ensureCapacity(int minimumCapacity)
{
Item[ ] biggerArray;
if (data.length < minimumCapacity)
{
biggerArray = new Item[minimumCapacity];
System.arraycopy(data, 0, biggerArray, 0, manyItems);
data = biggerArray;
}
}
/**
* Accessor method to get the current capacity of this bag.
* The add method works efficiently (without needing
* more memory) until this capacity is reached.
* @param - none
* @return
* the current capacity of this bag
**/
public int getCapacity( )
{
return data.length;
}
/**
* Remove one copy of a specified element from this bag.
* @param target
* the element to remove from the bag
* @postcondition
* If target was found in the bag, then one copy of
* target has been removed and the method returns true.
* Otherwise the bag remains unchanged and the method returns false.
**/
public boolean remove(String target)
{
int index; // The location of target in the data array.
// First, set index to the location of target in the data array,
// which could be as small as 0 or as large as manyItems-1; If target
// is not in the array, then index will be set equal to manyItems;
for (index = 0; (index < manyItems) && (!target.equals(data[index].getData1())); index++)
// No work is needed in the body of this for-loop.
;
if (index == manyItems)
// The target was not found, so nothing is removed.
return false;
else
{ // The target was found at data[index].
// So reduce manyItems by 1 and copy the last element onto data[index].
manyItems--;
data[index] = data[manyItems];
return true;
}
}
/**
* Determine the number of elements in this bag.
* @param - none
* @return
* the number of elements in this bag
**/
public int size( )
{
return manyItems;
}
/**
* Reduce the current capacity of this bag to its actual size (i.e., the
* number of elements it contains).
* @param - none
* @postcondition
* This bag's capacity has been changed to its current size.
* @exception OutOfMemoryError
* Indicates insufficient memory for altering the capacity.
**/
public void trimToSize( )
{
Item[ ] trimmedArray;
if (data.length != manyItems)
{
trimmedArray = new Item[manyItems];
System.arraycopy(data, 0, trimmedArray, 0, manyItems);
data = trimmedArray;
}
}
public ItemIterator iterator()
{
ItemIterator it;
it = new ItemIterator();
return (it);
}
public class ItemIterator implements Iterator
{
public ItemIterator()
{
// TODO - implement constructor
}
public boolean hasNext()
{
// TODO - implement hasNext
return false;
}
public Item next()
{
// TODO - implement next
return null;
}
}
-----------------------------------------------------------------------------------------------
Item.java
public class Item {
private String data1;
public String getData1() {
return (data1);
}
public void setData1(String data1) {
this.data1 = data1;
}
public String toString() {
String s;
s = "Data1 = \"" + data1 + "\"";
return (s);
}
}
------------------------------------------------------------------------------------------------------
Project1Applet.java
// This applet is a small example to illustrate how to write an interactive
// Applet to test the methods of another class.
// -- Michael Main (main@colorado.edu)
// -- Robin Ehrlich (Robin.Ehrlich@metrostate.edu)
import java.applet.Applet;
import java.awt.*; // Imports Button, Canvas, TextArea, TextField
import java.awt.event.*; // Imports ActionEvent, ActionListener
import java.util.Iterator;
public class Project1Applet extends Applet
{
private static final long serialVersionUID = 1L;
private ArrayBag b = new ArrayBag( );
// These are the interactive Components that will appear in the Applet.
// We declare one Button for each ArrayBag method that we want to be able to
// test. If the method has argument(s), then there is also TextField(s)
// where the user can enter the value of the argument.
// At the bottom, there is a TextArea to write messages.
private Button addButton = new Button("add");
private Button updateButton = new Button("update");
private Button deleteButton = new Button("delete");
private Label elementLabel1 = new Label("Element 1");
private TextField elementText1 = new TextField(20);
private Label elementLabel2 = new Label("Element 2");
private TextField elementText2 = new TextField(20);
private Label elementLabel3 = new Label("Element 3");
private TextField elementText3 = new TextField(20);
private Label elementLabel4 = new Label("Element 4");
private TextField elementText4 = new TextField(20);
private Label elementLabel5 = new Label("Element 5");
private TextField elementText5 = new TextField(20);
private Button searchButton = new Button("search");
private TextField targetText = new TextField(20);
private Button listAllButton = new Button("list all");
private Button sizeButton = new Button("size");
private TextArea feedback = new TextArea(7, 80);
public void init( )
{
// size window to contain all fields
resize(600, 500);
// set a useful window title
Frame frame = (Frame) this.getParent().getParent();
frame.setTitle("ICS 240 - Project 1 -
// remove unneeded menu
frame.setMenuBar(null);
add(new Label("This test program has created a bag."));
add(new Label("Press buttons to activate the bag's methods."));
addHorizontalLine(Color.blue);
// The Button and TextFields for testing the add, update and delete methods
add(elementLabel1);
add(elementText1);
addNewLine( );
add(elementLabel2);
add(elementText2);
addNewLine( );
add(elementLabel3);
add(elementText3);
addNewLine( );
add(elementLabel4);
add(elementText4);
addNewLine( );
add(elementLabel5);
add(elementText5);
addNewLine( );
add(addButton);
add(updateButton);
add(deleteButton);
addHorizontalLine(Color.blue);
// The Button and TextField for testing the search method:
add(searchButton);
add(targetText);
addHorizontalLine(Color.blue);
// The Button for testing the list and size method
add(listAllButton);
add(sizeButton);
addNewLine( );
// A TextArea at the bottom to write messages:
addHorizontalLine(Color.blue);
addNewLine( );
feedback.setEditable(false);
feedback.append("Ready for your first action. ");
add(feedback);
// Tell the Buttons what they should do when they are clicked:
addButton.addActionListener(new AddListener( ));
updateButton.addActionListener(new UpdateListener( ));
deleteButton.addActionListener(new DeleteListener( ));
searchButton.addActionListener(new SearchListener( ));
sizeButton.addActionListener(new SizeListener( ));
listAllButton.addActionListener(new ListAllListener( ));
}
class ListAllListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
int i;
ArrayBag.ItemIterator it;
Item item;
it = b.iterator();
i = 1;
while(it.hasNext()) {
item = it.next();
feedback.append(i + ": " + item.toString() + " ");
i += 1;
}
showStatus("List All action completed");
}
}
class SizeListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
feedback.append("The bag has size " + b.size( ) + ". ");
showStatus("Size action completed");
}
}
class AddListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
try
{
String userInput1 = elementText1.getText( );
String userInput2 = elementText2.getText();
String userInput3 = elementText3.getText();
String userInput4 = elementText4.getText();
String userInput5 = elementText5.getText();
// TODO - validate and do something with all of inputs
b.add(userInput1);
feedback.append(userInput1 + " has been added to the bag. ");
showStatus("Add action completed");
}
catch (NumberFormatException e)
{
feedback.append("Type an integer before clicking button. ");
elementText1.requestFocus( );
elementText1.selectAll( );
}
}
}
class UpdateListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
try
{
showStatus("Update action not yet implemented");
}
catch (NumberFormatException e)
{
feedback.append("Type an integer before clicking button. ");
elementText1.requestFocus( );
elementText1.selectAll( );
}
}
}
class DeleteListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
try
{
showStatus("Delete action not yet implemented");
}
catch (NumberFormatException e)
{
feedback.append("Type an integer before clicking button. ");
elementText1.requestFocus( );
elementText1.selectAll( );
}
}
}
class SearchListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
try
{
String userInput = targetText.getText( );
// TODO - do something with the input
// display Item value
showStatus("Search action not yet implemented");
}
catch (NumberFormatException e)
{
feedback.append("Type a target before clicking button. ");
targetText.requestFocus( );
targetText.selectAll( );
}
}
}
private void addHorizontalLine(Color c)
{
// Add a Canvas 10000 pixels wide but only 1 pixel high, which acts as
// a horizontal line to separate one group of components from the next.
Canvas line = new Canvas( );
line.setSize(10000, 1);
line.setBackground(c);
add(line);
}
private void addNewLine( )
{
// Add a horizontal line in the background color. The line itself is
// invisible, but it serves to force the next Component onto a new line.
addHorizontalLine(getBackground());
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
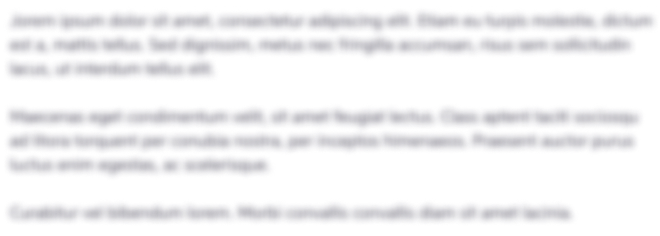
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started